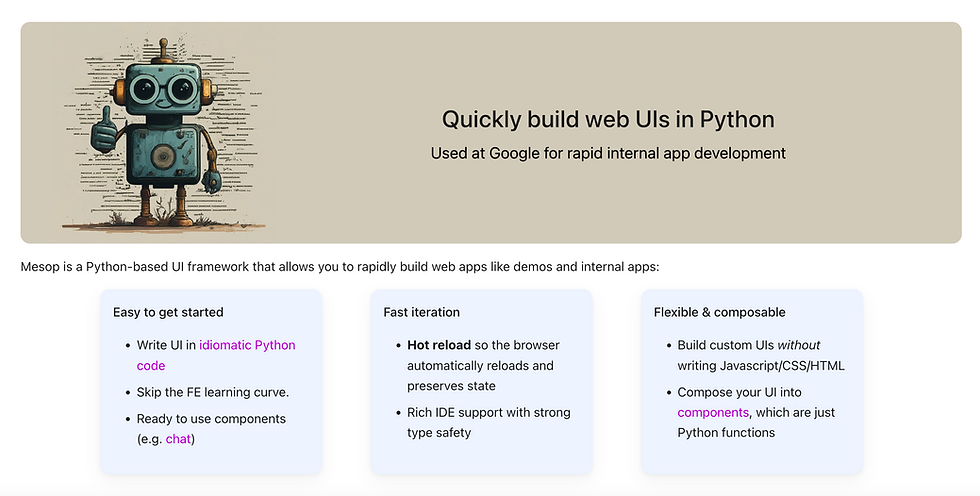
In the ever-evolving landscape of web development, creating beautiful and responsive user interfaces (UIs) has traditionally required a mix of JavaScript, CSS, and HTML. However, recent advancements have made it possible to build sophisticated UIs using just Python. One such tool that has emerged is Mesop, an internal package from a major tech company designed to simplify the process of building web UIs in pure Python. In this blog post, we’ll explore how to get started with Mesop, its key features, and a simple tutorial to create a chat application.
Why Mesop?
Mesop allows developers to build and prototype web applications rapidly without needing to delve into JavaScript, CSS, or HTML. This is particularly beneficial for those who are more comfortable with Python or are working on AI-related projects that can leverage Python's extensive libraries. Mesop offers ready-to-use components, state management, and support for multi-page applications, making it an excellent choice for both beginners and experienced developers.
Key Concepts
Components
Mesop offers a variety of native UI components that you can use out of the box. These include:
Chat Components: For building chatbots and interactive chat interfaces.
Text-to-Text Components: For applications where the input and output are both text.
Text-to-Image Components: For integrating AI models that generate images from text inputs.
File Upload Components: For handling file uploads.
Selection Components: Such as radio buttons and toggles for user selections.
State Management
One of Mesop's standout features is its state management system. This allows you to maintain the state of your application easily, making it simpler to develop interactive and dynamic UIs.
Multi-Page Applications
Mesop supports the creation of multi-page applications, enabling you to build complex UIs that span multiple pages without much hassle.
Getting Started with Mesop
Installation
First, you need to install the Mesop package. You can do this using pip:
pip install mesop
Basic Usage
Creating a Simple "Hello World" Page
Here’s how you can create a simple "Hello World" page with Mesop:
import mesop as me
from mesop import lab
@me.page('/hello')
def hello_world():
me.text("Hello World")
if __name__ == "__main__":
me.run()
When you run this script, it will start a local server. You can navigate to http://localhost:5000/hello to see your "Hello World" page.
Creating a Chat Application
Now, let's create a simple chat application using Mesop’s pre-built chat components.
Define the Chat Page:
import mesop as me
from mesop.lab import Chat
@me.page('/chat')
def chat_page():
me.chat(
title="Simple Chat",
transform=transform_function
)
def transform_function(prompt, history):
return f"Hello, {prompt}"
if __name__ == "__main__":
me.run()
Run the Application: Run the script and navigate to http://localhost:5000/chat. You will see a chat interface where you can type messages, and it will respond with "Hello, [Your Message]".
Advanced Features
State Management
Mesop’s state management allows you to keep track of variables across different components. Here’s an example of a simple counter application:
import mesop as me
class CounterState(me.State):
clicks = 0
@me.page('/counter')
def counter_page():
state = CounterState.get()
me.text(f"Clicks: {state.clicks}")
me.button("Increment", on_click=increment_clicks)
def increment_clicks():
state = CounterState.get()
state.clicks += 1
state.save()
if __name__ == "__main__":
me.run()
When you run this script and navigate to http://localhost:5000/counter, you will see a button that increments the counter each time it’s clicked.
Building More Complex Applications
Mesop also supports more complex applications that involve multiple pages and advanced UI components. For example, you can build an AI-powered chat application that uses an external API for generating responses.
import mesop as me
from mesop.lab import Chat
@me.page('/ai-chat')
def ai_chat_page():
me.chat(
title="AI Chat",
transform=ai_transform_function
)
def ai_transform_function(prompt, history):
# Replace with actual API call to an AI model
response = call_ai_model(prompt)
return response
def call_ai_model(prompt):
# Mock function to simulate an API call
return f"AI Response to: {prompt}"
if __name__ == "__main__":
me.run()
Conclusion
Mesop offers a powerful yet simple way to build beautiful and responsive web UIs using pure Python. Its ready-to-use components, robust state management, and support for multi-page applications make it a versatile tool for both rapid prototyping and production-ready applications. Whether you are an AI researcher, a data scientist, or a Python developer looking to build web interfaces, Mesop is definitely worth exploring.
For further details and to explore more advanced features, check out the GitHub repository and the official documentation:
GitHub: Mesop GitHub Repository
Documentation: Mesop Documentation
Happy coding! If you have any questions or need further assistance, feel free to leave a comment below.
Comments