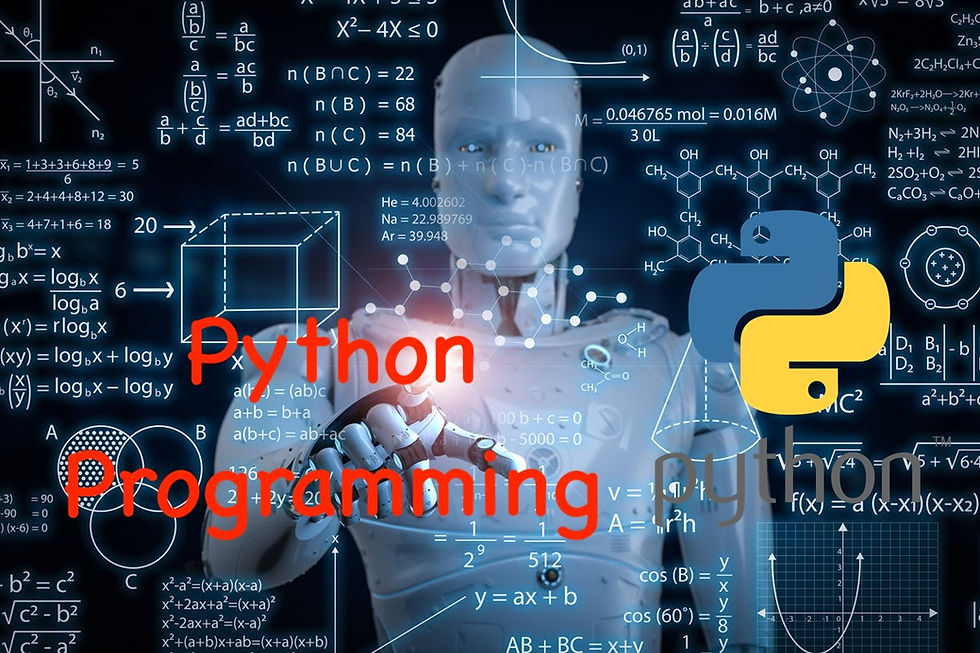
Hello, amazing coders! Welcome to our blog, where we simplify the complexities of coding and make learning fun. Today, we’re going to dive into an interesting question that often pops up in interviews: "Can we have multiple constructors in Python?" We’ll explore this concept using a simple class example, discuss the challenges, and discover a nifty hack to achieve our goal. Ready to get started? Let’s go!
What is a Constructor?
A constructor in Python is a special method called init. It’s automatically invoked when an object of the class is created. Think of it as the initial setup for your object, where you define its attributes.
The Problem: Multiple Constructors
In some programming languages, you can have multiple constructors, each with different parameters. However, in Python, if you try to define more than one init method, the last one will overwrite the previous ones.
Example Code
Let's start with a simple class called Animal.
class Animal:
def __init__(self, name, species):
self.name = name
self.species = species
# Creating an object of Animal
dog = Animal("Dog", "Mammal")
print(dog.name) # Output: Dog
print(dog.species) # Output: Mammal
Here, we have a basic constructor that takes two parameters: name and species. But what if we want another constructor that also takes the animal's age?
Trying Multiple Constructors
class Animal:
def __init__(self, name, species):
self.name = name
self.species = species
def __init__(self, name, species, age):
self.name = name
self.species = species
self.age = age
# Creating an object of Animal
dog = Animal("Dog", "Mammal")
If you run this code, you’ll get an error because the second init method has overwritten the first one.
The Hack: Using Positional Arguments
We can use a clever trick with positional arguments to mimic multiple constructors. Let's see how!
Using *args
We’ll use *args to accept a variable number of arguments and then handle them inside the constructor.
class Animal:
def __init__(self, *args):
if len(args) == 2:
self.name = args[0]
self.species = args[1]
elif len(args) == 3:
self.name = args[0]
self.species = args[1]
self.age = args[2]
def make_sound(self, sound):
return f"{self.name} says {sound}"
# Creating objects of Animal
dog = Animal("Dog", "Mammal")
cat = Animal("Cat", "Mammal", 3)
print(dog.name, dog.species) # Output: Dog Mammal
print(cat.name, cat.species, cat.age) # Output: Cat Mammal 3
print(dog.make_sound("Woof")) # Output: Dog says Woof
How It Works
*args allows us to pass a variable number of arguments.
We use len(args) to check how many arguments were passed and assign them accordingly.
This way, we can handle different numbers of parameters with a single constructor.
Conclusion
Today, we tackled the challenge of having multiple constructors in Python. While Python doesn’t support multiple init methods directly, we used positional arguments to achieve similar functionality. This hack allows us to handle different initialization scenarios without overwriting previous constructors.
Remember, while this trick is handy, it’s essential to use it wisely and keep your code clean and readable. Happy coding, and keep exploring the wonders of Python!
If you enjoyed this blog post, don’t forget to subscribe for more exciting content. Keep coding, stay curious, and have fun!
Thank you for reading, and see you in the next post!
Comments