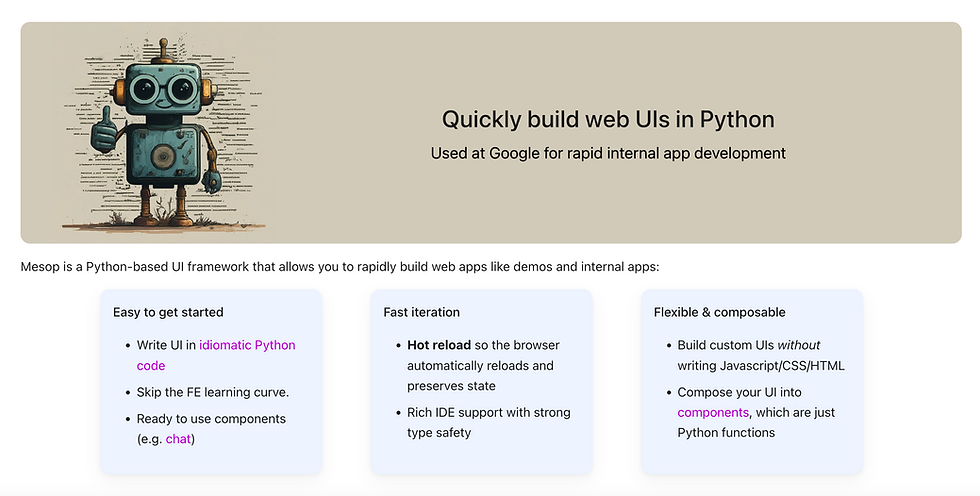
Creating user interfaces for AI models can be daunting, especially for those who are not well-versed in frontend development. Fortunately, Google Mesop simplifies this process, allowing you to create sophisticated user interfaces with just a few lines of code. In this blog post, we will walk through creating a chatbot using Google Mesop and various AI models (Groq, Ollama, Google Generative AI, Llama3).
Setting Up the Environment
Before diving into the code, you need to set up your development environment. Follow these steps:
Create and Activate a Virtual Environment:
conda create -p venv python=3.10
conda activate venv/
Add Required Packages to requirements.txt:
mesop
openai
google-generativeai
ollama
groq
llama-index
llama-index-llms-anthropic
langchain-community
python-dotenv
Install the Required Packages:
pip install -r requirements.txt
Building the Chatbot Interface with Google Mesop
Let's walk through the code to build the chatbot interface and integrate various AI models.
import os
from dotenv import load_dotenv
import mesop as me
import mesop.labs as mel
from mesop import stateclass
import groq
import ollama
import google.generativeai as genai
from langchain_community.llms import Ollama as LangchainOllama
# Load environment variables from .env file
load_dotenv()
# Initialize clients for different AI models
groq_client = groq.Groq(api_key=os.environ["GROQ_API_KEY"])
ollama_llm = LangchainOllama(model="llama3")
genai.configure(api_key=os.environ["GEMINI_API_KEY"])
# Configuration for Google Generative AI
generation_config = {
"temperature": 0.5,
"top_p": 0.90,
"top_k": 65,
"max_output_tokens": 8192,
"response_mime_type": "text/plain",
}
# Initialize Google Generative AI model
genai_model = genai.GenerativeModel(
model_name="gemini-1.5-flash",
generation_config=generation_config,
system_instruction="You are a helpful assistant, you provide helpful answers."
)
# Define the state class to keep track of the selected model
@stateclass
class State:
selected_model: str = "groq"
# Define an option class for model selection
class Option:
def __init__(self, label, value):
self.label = label
self.value = value
# Define the main page for the Mesop app
@me.page(
security_policy=me.SecurityPolicy(
allowed_iframe_parents=["https://google.github.io"]
),
path="/",
title="Mesop Demo Chat",
)
def page(state: State = State()):
options = [
Option(label="Groq", value="groq"),
Option(label="Ollama", value="ollama"),
Option(label="Google GenAI", value="google_genai"),
Option(label="Llama3", value="llama3")
]
# Create a dropdown for model selection
selected_model = me.select(
options=options,
value=state.selected_model,
label="Model Selector"
)
# Update the selected model when the button is clicked
if me.button("Select Model"):
state.selected_model = selected_model
# Create a chat interface
mel.chat(lambda input, history: transform(input, history, state), title="Mesop Demo Chat", bot_user="Mesop Bot")
# Transform function to handle the input and history based on the selected model
def transform(input: str, history: list[mel.ChatMessage], state: State):
model = state.selected_model
if model == "groq":
return groq_transform(input, history)
elif model == "ollama":
return ollama_transform(input, history)
elif model == "google_genai":
return genai_transform(input, history)
elif model == "llama3":
return llama3_transform(input, history)
# Function to handle Groq model responses
def groq_transform(input: str, history: list[mel.ChatMessage]):
messages = [{"role": "system", "content": "You are a helpful assistant."}]
messages.extend([{"role": "user", "content": message.content} for message in history])
messages.append({"role": "user", "content": input})
# Stream response from Groq model
stream = groq_client.chat.completions.create(
messages=messages,
model="llama3-8b-8192",
temperature=0.5,
max_tokens=1024,
top_p=1,
stop=None,
stream=True,
)
for chunk in stream:
content = chunk.choices[0].delta.content
if content:
yield content
# Function to handle Ollama model responses
def ollama_transform(input: str, history: list[mel.ChatMessage]):
messages = [{"role": "user", "content": message.content} for message in history]
messages.append({"role": "user", "content": input})
# Stream response from Ollama model
stream = ollama.chat(model='llama3', messages=messages, stream=True)
for chunk in stream:
content = chunk.get('message', {}).get('content', '')
if content:
yield content
# Function to handle Google Generative AI responses
def genai_transform(input: str, history: list[mel.ChatMessage]):
chat_history = "\n".join(message.content for message in history)
full_input = f"{chat_history}\n{input}"
# Stream response from Google Generative AI
response = genai_model.generate_content(full_input, stream=True)
for chunk in response:
yield chunk.text
# Function to handle Llama3 model responses
def llama3_transform(input: str, history: list[mel.ChatMessage]):
messages = [f"System: You are a helpful assistant."]
messages.extend([f"User: {message.content}" for message in history])
messages.append(f"User: {input}")
query = "\n".join(messages)
# Stream response from Llama3 model
resp = ollama_llm.stream(query)
for chunk in resp:
if chunk:
yield chunk
# Run the Mesop app
if __name__ == "__main__":
me.run()
Running the Application
To run the application, execute the following command in your terminal:
mesop app.py
This will start a local server, and you can open the provided URL in your web browser to interact with the chatbot.
Conclusion
Creating a chatbot with Google Mesop is straightforward and requires minimal code. By leveraging AI models and Mesop's intuitive interface, you can build powerful applications quickly. Whether you're using Groq, Ollama, Google Generative AI, or any other model, the process remains largely the same, making it easy to switch between different AI solutions.
Feel free to experiment with different models and configurations to see what works best for your needs. Happy coding!
I hope you found this guide helpful. If you have any questions or run into issues, feel free to leave a comment below. Happy coding!
Comments