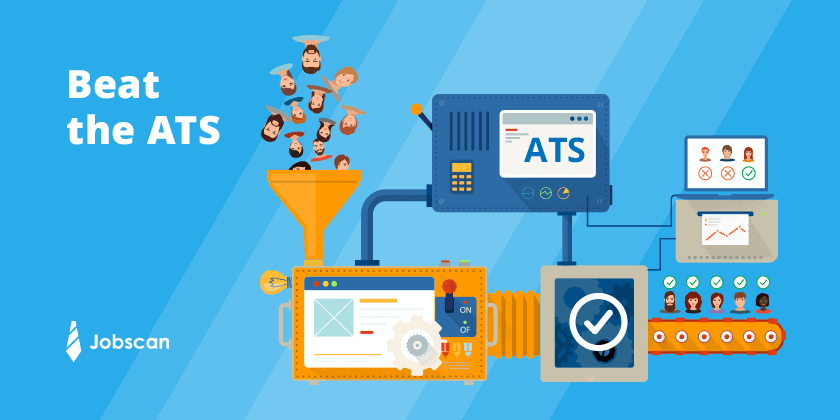
In today's competitive job market, having a resume that can successfully pass through an Application Tracking System (ATS) is crucial. An ATS helps companies filter and rank job applications by scanning resumes and matching them with job descriptions. In this project, we will build an ATS system using Generative AI to match resumes with job descriptions and provide feedback on how to improve the resumes.
Project Overview
Introduction to ATS Systems
Setting Up the Environment
Creating the Requirements File
Writing the Code Step-by-Step
Additional Improvements and Considerations
Introduction to ATS Systems
An ATS system is used by companies to filter and rank job applications. It scans resumes and matches them with job descriptions to ensure only the most qualified candidates move forward in the hiring process. In this project, we will provide a job description and upload a resume in PDF format. The system will then analyze the resume against the job description and provide feedback on how well the resume matches the job requirements, highlighting key points and suggesting improvements.
Setting Up the Environment
First, we need to set up a virtual environment to manage our project dependencies.
conda create -n ats_system_env python=3.10
conda activate ats_system_env
Next, create a .env file to store your API key:
GOOGLE_API_KEY=your_api_key_here
Creating the Requirements File
Create a requirements.txt file with the following content:
streamlit
google-generativeai
python-dotenv
PyPDF2
Install the dependencies:
pip install -r requirements.txt
Writing the Code Step-by-Step
Now, let’s start writing the code. Create a file named app.py and add the following code:
Import Libraries and Load Environment Variables
import streamlit as st
import google.generativeai as genai
import os
import PyPDF2 as pdf
from dotenv import load_dotenv
# Load environment variables from .env file
load_dotenv()
genai.configure(api_key=os.getenv("GOOGLE_API_KEY"))
Function to Load the Generative AI Model and Get Responses
def get_gemini_response(input):
model = genai.GenerativeModel('gemini-pro')
response = model.generate_content(input)
return response.text
Function to Extract Text from PDF
def input_pdf_text(uploaded_file):
reader = pdf.PdfReader(uploaded_file)
text = ""
for page in range(len(reader.pages)):
page = reader.pages[page]
text += str(page.extract_text())
return text
Prompt Template
This prompt will instruct the AI on how to behave and evaluate the resume based on the job description.
input_prompt = """
Hey Act Like a skilled or very experienced ATS(Application Tracking System)
with a deep understanding of tech field, software engineering, data science, data analyst
and big data engineer. Your task is to evaluate the resume based on the given job description.
You must consider the job market is very competitive and you should provide
best assistance for improving the resumes. Assign the percentage Matching based
on Jd and
the missing keywords with high accuracy
resume:{text}
description:{jd}
I want the response in one single string having the structure
{{"JD Match":"%","MissingKeywords:[]","Profile Summary":""}}
"""
Streamlit App Setup
# Initialize Streamlit app
st.title("Smart ATS")
st.text("Improve Your Resume with ATS")
# Input for job description
jd = st.text_area("Paste the Job Description")
# File uploader for resume
uploaded_file = st.file_uploader("Upload Your Resume", type="pdf", help="Please upload the PDF")
submit = st.button("Submit")
# Handling the submit button
if submit:
if uploaded_file is not None:
text = input_pdf_text(uploaded_file)
formatted_prompt = input_prompt.format(text=text, jd=jd)
response = get_gemini_response(formatted_prompt)
st.subheader("ATS Evaluation Result")
st.write(response)
else:
st.write("Please upload the resume")
Running the Application
To start the application, run the following command:
streamlit run app.py
This will open a web interface where you can input a job description, upload a resume in PDF format, and get feedback on how well the resume matches the job description, along with suggestions for improvement.
Additional Improvements and Considerations
While the basic functionality is now in place, there are several improvements and additional features you could consider:
Error Handling: Add more robust error handling to manage issues like unsupported file formats or missing API keys.
Advanced Analysis: Enhance the app to provide more detailed analysis and feedback on the resume.
UI/UX Enhancements: Improve the user interface for better user experience, perhaps by adding more descriptive labels or progress indicators.
Security Measures: Implement security measures to protect sensitive information in resumes and job descriptions.
Conclusion
Congratulations! You've built a complete end-to-end ATS system for resumes using Generative AI. This project showcases how powerful AI can be in automating the analysis of resumes and matching them with job descriptions, making it a valuable tool for both job seekers and recruiters.
If you have any questions or suggestions, feel free to leave a comment. Happy coding!
Comments