Part 3: Dive into Python Data Structures: Lists, Booleans, and More!
- Revanth Reddy Tondapu
- Jun 1, 2024
- 3 min read
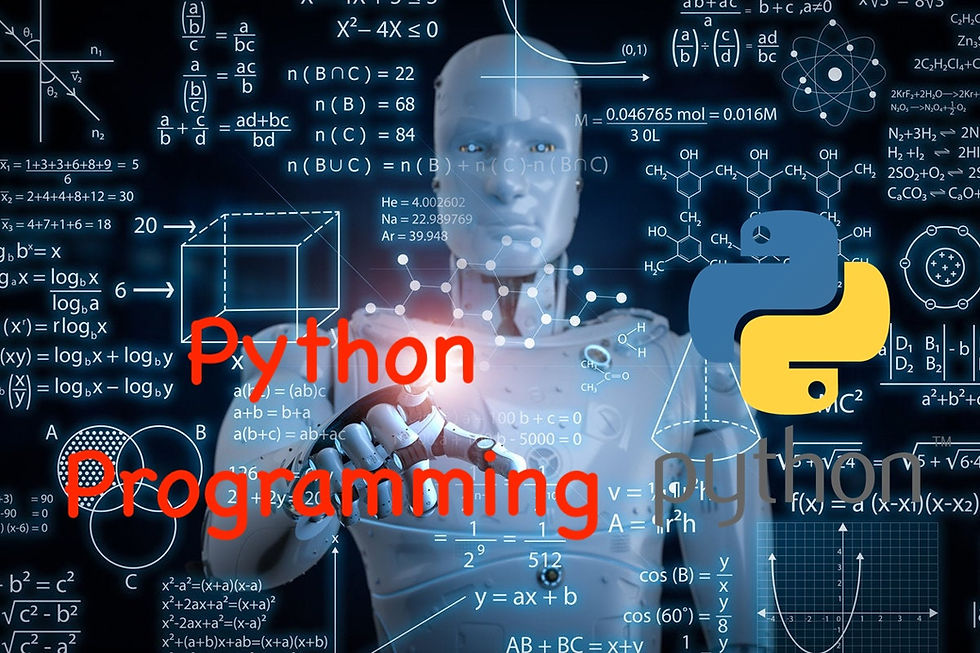
Hello everyone! Today, we’re going to dive deep into some fundamental data structures in Python, including booleans, logical operators, lists, dictionaries, tuples, and sets. Understanding these is crucial, especially when working with data in machine learning. Let’s get started!
Booleans and Logical Operators
What are Boolean Variables?
Booleans are the simplest data type in Python, representing the truth values: True and False. These are useful for making decisions in your code.
To create a boolean variable in Python, you can use the bool function or directly assign True or False:
is_sunny = True
is_raining = False
print(type(is_sunny)) # Output: <class 'bool'>
Logical Operators
Logical operators allow us to combine multiple boolean expressions. The primary logical operators are and, or, and not.
and: Returns True if both expressions are true.
or: Returns True if at least one expression is true.
not: Inverts the truth value of the expression.
Here’s an example to illustrate:
is_sunny = True
is_weekend = False
# Using 'and'
print(is_sunny and is_weekend) # Output: False
# Using 'or'
print(is_sunny or is_weekend) # Output: True
# Using 'not'
print(not is_sunny) # Output: False
Lists
What is a List?
A list is a collection of items that is ordered and changeable. Each item in a list can be of any data type, including numbers, strings, and even other lists.
Here’s how to create a list:
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
mixed = ["apple", 2, True, 3.5]
Accessing List Items
You can access items in a list using their index. Remember, Python indices start at 0.
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
print(fruits[-1]) # Output: cherry (negative index starts from the end)
Adding and Removing Items
You can add items to a list using append() and remove items using pop() or remove():
# Adding items
fruits.append("orange")
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
# Removing items
fruits.pop() # Removes the last item
print(fruits) # Output: ['apple', 'banana', 'cherry']
fruits.remove("banana")
print(fruits) # Output: ['apple', 'cherry']
Slicing Lists
You can get a range of items from a list using slicing:
fruits = ["apple", "banana", "cherry", "date"]
print(fruits[1:3]) # Output: ['banana', 'cherry']
print(fruits[:2]) # Output: ['apple', 'banana']
print(fruits[2:]) # Output: ['cherry', 'date']
Dictionaries
What is a Dictionary?
A dictionary is a collection of key-value pairs. Each key must be unique, and it maps to a value.
Here’s how to create a dictionary:
student = {
"name": "Revanth",
"age": 12,
"grade": "7th"
}
Accessing Dictionary Items
You can access dictionary items using their keys:
print(student["name"]) # Output: Revanth
print(student["age"]) # Output: 12
Adding and Removing Items
You can add new key-value pairs or update existing ones, and remove pairs using del:
# Adding or updating
student["school"] = "XYZ High School"
print(student)
# Removing
del student["age"]
print(student)
Tuples
What is a Tuple?
A tuple is a collection of items that is ordered and unchangeable. Tuples are written with round brackets.
Here’s how to create a tuple:
fruits = ("apple", "banana", "cherry")
Accessing Tuple Items
You access items in a tuple the same way you do with lists:
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
Sets
What is a Set?
A set is a collection of items that is unordered and unindexed. Sets are written with curly brackets.
Here’s how to create a set:
fruits = {"apple", "banana", "cherry"}
Adding and Removing Items
You can add items to a set using add() and remove items using remove():
# Adding items
fruits.add("orange")
print(fruits)
# Removing items
fruits.remove("banana")
print(fruits)
Conclusion
Understanding these basic data structures—booleans, lists, dictionaries, tuples, and sets—is essential when working with Python, especially for data analysis and machine learning. They help you organize and manipulate data efficiently.
Feel free to explore these concepts further by experimenting with the examples given. Happy coding!
Thank you for reading! If you found this post helpful, please share it with your friends and family. Happy learning!
Comments