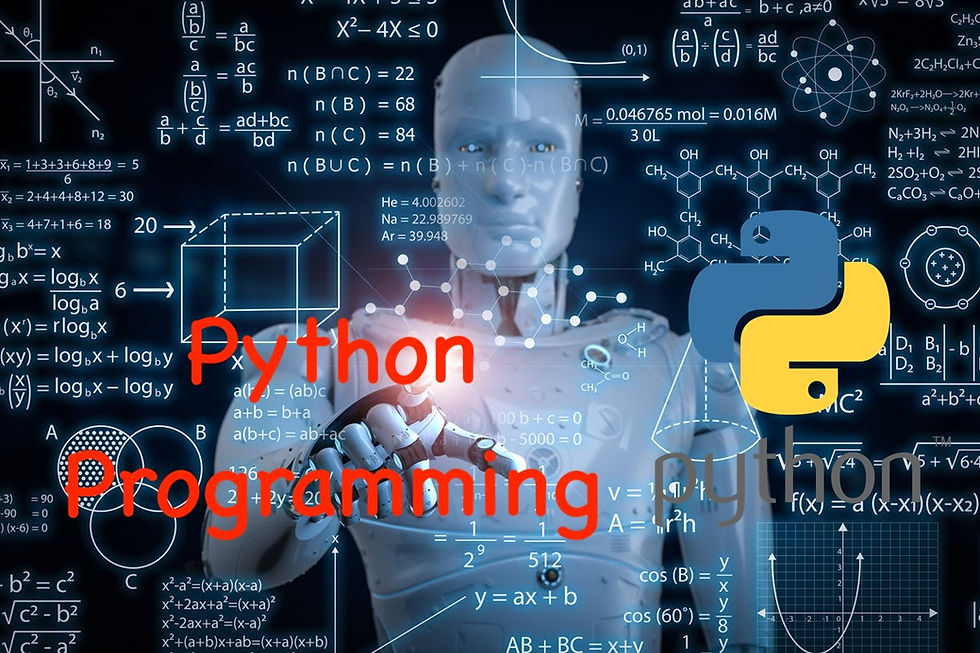
Hello young coders! Welcome to another exciting tutorial in our Advanced Python Series. Today, we are diving into the world of Decorators. These might sound fancy, but don't worry! We'll break them down step-by-step. By the end of this post, understand what decorators are, how to use them, and why they're so useful. Ready? Let's get started!
What Are Decorators?
Decorators are a powerful tool in Python that allow you to modify the behavior of a function or class. They are essentially functions that take another function and extend its behavior without explicitly modifying it.
Why Use Decorators?
Decorators are used to:
Add Functionality: They allow you to add new functionality to an existing function.
Code Reusability: They help you write cleaner, reusable code.
Simplify Code: They can make your code more readable and maintainable.
Steps to Understand Decorators
Before we dive into decorators, let's understand two important concepts: Function Copying and Closures.
Step 1: Function Copying
Imagine you have a function that prints a welcome message. You can assign this function to a new variable and use it just like the original function.
def welcome():
return "Welcome to Revanth's coding class!"
# Copy the function to a new variable
greet = welcome
# Now, greet can be used like the welcome function
print(greet()) # Output: Welcome to Revanth's coding class!
Step 2: Closures
Closures are functions defined inside other functions. The inner function has access to the variables of the outer function even after the outer function has finished executing.
def outer_function(msg):
def inner_function():
print(f"Message: {msg}")
return inner_function
# Create a closure
my_closure = outer_function("Hello, World!")
my_closure() # Output: Message: Hello, World!
Step 3: Understanding Decorators
Now, let's combine these concepts to understand decorators.
Creating a Simple Decorator
A decorator is a function that takes another function as an argument, adds some functionality, and returns a new function.
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
def say_hello():
print("Hello!")
# Use the decorator
decorated_function = my_decorator(say_hello)
decorated_function()
Using the @ Syntax
Python provides a special syntax to apply decorators more conveniently using the @ symbol.
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Explanation:
my_decorator: This is the decorator function.
wrapper: This is the inner function that adds extra functionality.
@my_decorator: This applies the decorator to the say_hello function.
Real-World Example: Logging
Let's create a decorator that logs the execution time of a function.
import time
def timer_decorator(func):
def wrapper():
start_time = time.time()
func()
end_time = time.time()
print(f"Function took {end_time - start_time} seconds to execute.")
return wrapper
@timer_decorator
def slow_function():
time.sleep(2)
print("Slow function executed")
slow_function()
Explanation:
timer_de: This decorator measures and prints the execution time.
slow_function: This function simulates a operation by sleeping for 2 seconds.
Conclusion
Decorators are a fantastic way to add functionality to your functions in a clean and reusable manner. They help keep your code organized and efficient. By understanding function copying and closures, you can easily grasp how decorators work.
Keep practicing these concepts, and soon you'll be a pro at using decorators in Python. Stay tuned for more exciting tutorials, and happy coding!
If you enjoyed this tutorial, make sure to share it with your friends and keep exploring the world of Python. Thank you for joining us, and see you in the next tutorial!
Comments