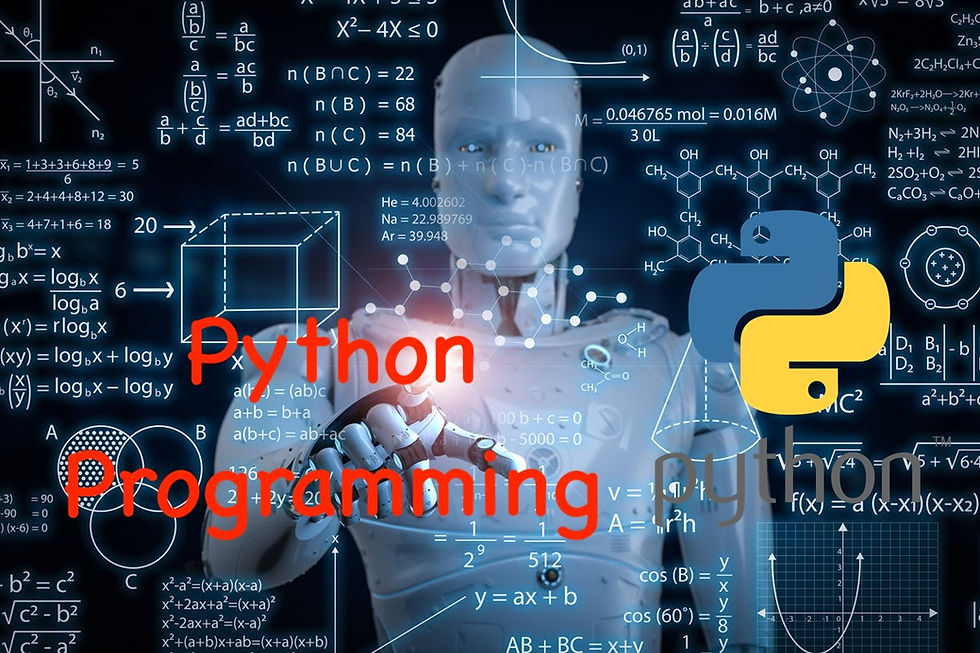
Hello young coders! Welcome to our exciting Python journey. Today, we're going to explore a fascinating concept in Python called "Inheritance." Don't worry if this sounds complicated—I'll break it down into simple and fun steps. By the end of this guide, you'll be a pro at understanding and using inheritance in Python!
What is Inheritance?
Imagine you have a blueprint for a basic car. Now, let's say you want to create a special type of car, like an Audi. Instead of designing the Audi from scratch, you can reuse the blueprint of the basic car and add some extra features. This is exactly what inheritance does in programming—it allows you to create a new class based on an existing class, reusing its properties and methods.
Why Use Inheritance?
Inheritance makes your code:
Reusable: You can use the existing code and add new features.
Organized: It helps in organizing and structuring your code better.
Efficient: Reduces code duplication, making your programs more efficient.
Creating a Basic Car Class
Let's start by creating a basic car class.
class Car:
def __init__(self, windows, doors, engine_type):
self.windows = windows
self.doors = doors
self.engine_type = engine_type
def drive(self):
print("The person drives the car")
Explanation
Class Definition: class Car: defines our Car class.
Constructor: init method initializes the car's attributes like windows, doors, and engine type.
Method: drive() is a method that prints a message.
Creating an Audi Class by Inheriting the Car Class
Now, let's create an Audi class that inherits from the Car class and adds some new features.
class Audi(Car):
def __init__(self, windows, doors, engine_type, enable_ai):
super().__init__(windows, doors, engine_type)
self.enable_ai = enable_ai
def self_driving(self):
print("Audi supports self-driving")
Explanation
Inheritance: class Audi(Car): means Audi class inherits from Car class.
Super Constructor: super().__init__(windows, doors, engine_type) calls the parent class's constructor to initialize inherited attributes.
New Attribute: self.enable_ai is a new attribute specific to Audi.
New Method: self_driving() is a new method specific to Audi.
Using the Audi Class
Let's create an object of the Audi class and see how it works.
audi_q7 = Audi(5, 4, "Diesel", True)
# Access inherited attributes and methods
print(audi_q7.windows) # Output: 5
print(audi_q7.doors) # Output: 4
print(audi_q7.engine_type) # Output: Diesel
audi_q7.drive() # Output: The person drives the car
# Access Audi-specific attributes and methods
print(audi_q7.enable_ai) # Output: True
audi_q7.self_driving() # Output: Audi supports self-driving
Explanation
Creating Object: audi_q7 = Audi(5, 4, "Diesel", True) creates an Audi object.
Access Inherited Attributes: audi_q7.windows, audi_q7.doors, audi_q7.engine_type access attributes inherited from the Car class.
Call Inherited Method: audi_q7.drive() calls the drive method inherited from the Car class.
Access New Attribute: audi_q7.enable_ai accesses the new attribute.
Call New Method: audi_q7.self_driving() calls the new method specific to Audi.
Conclusion
Inheritance is a powerful feature in Python that helps you reuse code, making your programs more efficient and organized. By creating a new class based on an existing class, you can add new features without starting from scratch.
Keep experimenting with inheritance and try creating different types of classes based on existing ones. The more you practice, the better you'll understand how to use inheritance to make your code more powerful and efficient.
I hope you enjoyed this guide. Stay tuned for more fun and advanced Python topics. Happy coding!
Comments