Part 17: Filtering Fun with Python: Understanding the Filter Function
- Revanth Reddy Tondapu
- May 18, 2024
- 3 min read
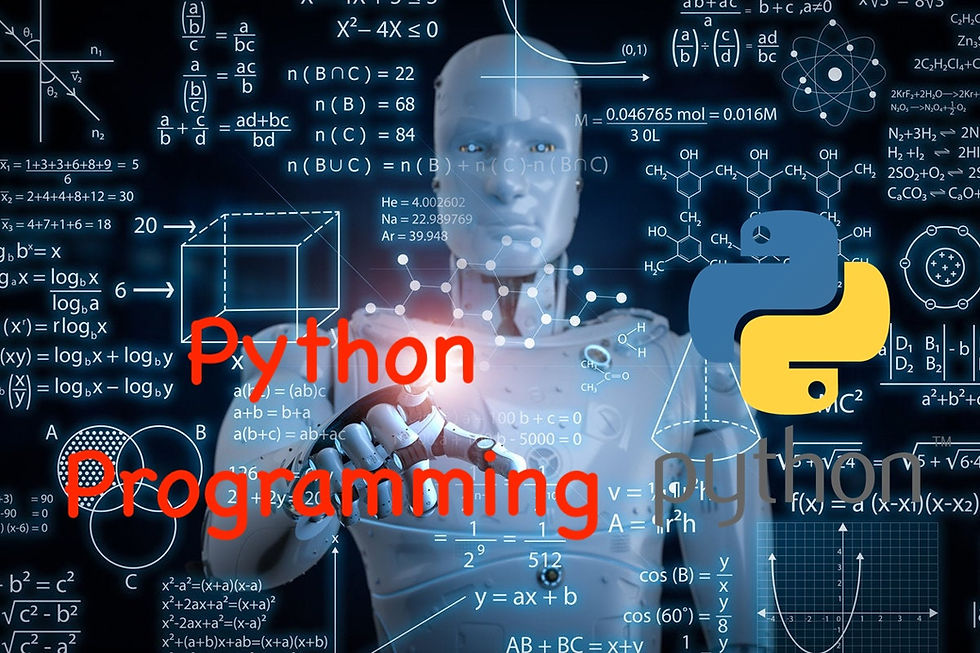
Hello everyone! Today, we're going to explore another powerful tool in Python—the filter function. If you've ever wondered how to sift through a list and pick out only the items you need, then this is the right place for you. We'll learn what the filter function does, how to use it, and see some cool examples. Let's dive in!
What is the Filter Function?
The filter function in Python helps you filter out elements from an iterable (like a list or a tuple) based on a specific condition. Think of it like a sieve that only lets certain items pass through while catching others.
Why Use the Filter Function?
The filter function is useful because it:
Simplifies the process of filtering elements
Makes your code cleaner and more readable
Is more efficient than writing a loop for large datasets
Getting Started with a Simple Example
Let's start by creating a simple function that checks whether a number is even. We'll then use this function to filter out all the even numbers from a list.
Creating the Even Check Function
def is_even(num):
return num % 2 == 0
# Using function
print(is_even(4)) # Output: True
print(is_even(5)) # Output: False
This function takes a number as an input and returns True if the number is even, and False if it's not.
Filtering a List of Numbers
Now, let's say we have a list of numbers and we want to filter out only the even numbers. Normally, we would write a loop to go through each number and check if it's even, but with the filter function, we can do this much more easily.
Using the Filter Function
The filter function takes two arguments:
The function you want to use for filtering
The iterable (like a list) you want to filter
Here's how we use the filter function with our is_even function and a list of numbers:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Using the filter function
even_numbers = filter(is_even, numbers)
# Converting the filter object to a list to see the results
even_numbers_list = list(even_numbers)
print(even_numbers_list) # Output: [2, 4, 6, 8, 10]
How Does It Work?
Function Name: We provide the name of the function (is_even) without parentheses.
Iterable: We provide the list of numbers (numbers).
The filter function applies is_even to each number in the list and returns a filter object containing only the elements that meet the condition (i.e., the even numbers). We then convert this filter object to a list to see the results.
Using Lambda Functions with Filter
We can make our code even shorter and cleaner by using a lambda function. A lambda function is a small anonymous function that can have any number of arguments but only one expression.
Using a Lambda Function with Filter
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Using the filter function with a lambda function
even_numbers = filter(lambda num: num % 2 == 0, numbers)
# Converting the filter object to a list to see the results
even_numbers_list = list(even_numbers)
print(even_numbers_list) # Output: [2, 4, 6, 8, 10]
Here, we use a lambda function to check if a number is even and apply it directly with the filter function.
Comparing Map and Filter Functions
While both the map and filter functions are used to apply a function to an iterable, they serve different purposes:
The map function applies a function to all items and returns a new list with the results.
The filter function applies a function to all items and returns a new list with only the items that meet the condition.
Example with Map
numbers = [1, 2, 3, 4, 5]
# Using the map function to check if numbers are even
result = map(lambda num: num % 2 == 0, numbers)
# Converting the map object to a list to see the results
result_list = list(result)
print(result_list) # Output: [False, True, False, True, False]
Example with Filter
numbers = [1, 2, 3, 4, 5]
# Using the filter function to get only even numbers
even_numbers = filter(lambda num: num % 2 == 0, numbers)
# Converting the filter object to a list to see the results
even_numbers_list = list(even_numbers)
print(even_numbers_list) # Output: [2, 4]
Notice how the map function returns a list of True and False values indicating which numbers are even, while the filter function returns only the even numbers.
Conclusion
The filter function is a powerful and efficient tool in Python that allows you to filter out elements from an iterable based on a specific condition. It's perfect for making your code cleaner and more readable. Whether you're working with numbers, strings, or other data types, the filter function can help you get the job done quickly and elegantly.
I hope you found this guide helpful and fun! Stay tuned for our next session, where we'll explore another useful function called reduce. Until then, happy coding!
Kommentare