Part 1: Getting Started with Python Programming: A Fun and Simple Guide
- Revanth Reddy Tondapu
- Jun 3, 2024
- 4 min read
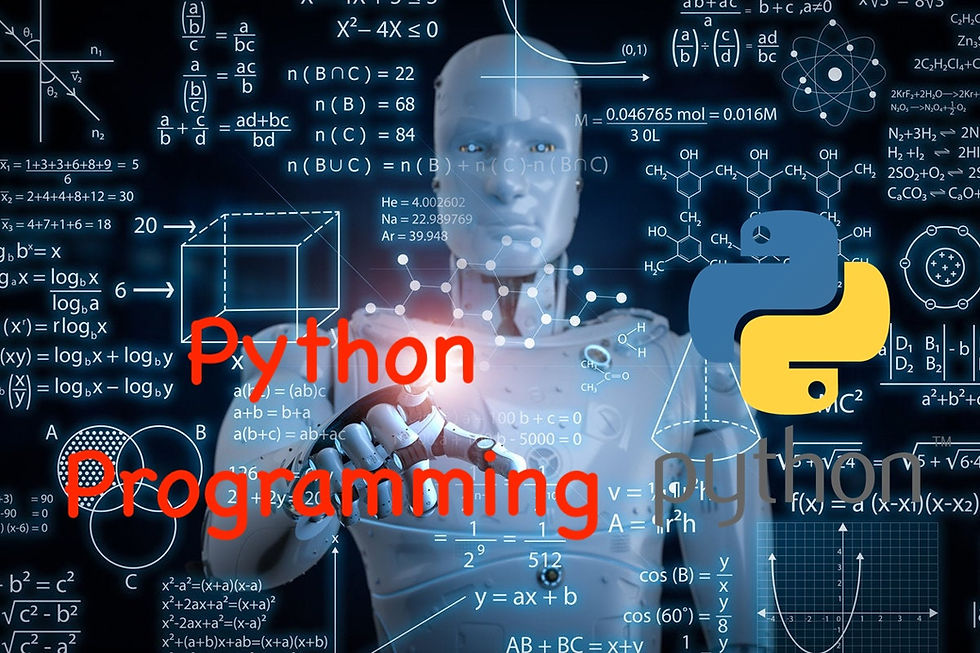
Hello everyone! I’m thrilled to welcome you to this fun and simple guide to learning Python programming. Whether you're completely new to coding or have some experience, this guide will help you understand the basics of Python, a powerful and easy-to-learn programming language.
Python is widely used in many fields, including machine learning and data analysis. It’s a great starting point if you’re interested in exploring these exciting areas. We will cover everything from installing Python to using some of its basic libraries and understanding key concepts. Let’s dive in!
Installing Python and Anaconda
Why Anaconda?
Anaconda is a software distribution that makes it easy to install and manage Python and its libraries. It comes with useful tools like Jupyter Notebook, which is excellent for writing and running Python code interactively.
Steps to Install Anaconda
Download Anaconda:
Open your web browser and search for "Anaconda downloads".
Click on the first link you see.
Navigate to the download section and choose the installer for your operating system (Windows, MacOS, or Linux).
Download the Python 3.7 version.
Install Anaconda:
Run the downloaded installer.
Follow the instructions on the screen and make sure to check all the boxes during the installation process.
Launching Jupyter Notebook:
Open your terminal (Mac/Linux) or command prompt (Windows).
Type jupyter notebook and press Enter. This will open Jupyter Notebook in your default web browser.
Exploring Jupyter Notebook
Jupyter Notebook is an interactive environment where you can write and execute Python code. It’s an excellent tool for learning and practicing Python.
Create a New Notebook:
In Jupyter Notebook, click on “New” and select “Python 3” to create a new notebook.
Write and Execute Code:
You can write Python code in the cells provided and execute them by pressing Shift + Enter. The output will be displayed directly below the cell.
Understanding Basic Python Concepts
Data Types and Variable Assignment
In Python, you can store different types of data in variables. Let’s look at some examples:
# Variable assignment
age = 12
height = 4.5
name = "Revanth"
is_student = True
# Checking data types
print(type(age)) # Output: <class 'int'>
print(type(height)) # Output: <class 'float'>
print(type(name)) # Output: <class 'str'>
print(type(is_student)) # Output: <class 'bool'>
Basic Operations
Python can perform basic arithmetic operations like addition, subtraction, multiplication, and division.
# Arithmetic operations
print(2 + 3) # Output: 5
print(5 - 2) # Output: 3
print(4 * 3) #: 12
print(8 / 2) # Output: 4.0
# Exponentiation
print(2 ** 3) # Output: 8 (2 raised to the power of 3)
Working with Strings
Strings are sequences of characters and can be defined using single or double quotes.
# String assignment
greeting = "Hello"
name = 'Revanth'
# Concatenation
message = greeting + ", " + name + "!"
print(message) # Output: Hello, Revanth!
Print Formatting
You can use the print function to display output. For more complex statements, the format method is handy.
# Using format method
first_name = "Revanth"
last_name = "Nair"
print("My first name is {} and my last name is {}.".format(first_name, last_name))
# Named placeholders
print("My first name is {first} and my last name is {last}.".format(first=first_name, last=last_name))
Using Jupyter Notebook Features
Jupyter Notebook provides several features to enhance your coding experience:
Markdown Cells: Use markdown cells to write formatted text, which is useful for adding descriptions and comments.
# This is a heading
## This is a sub-heading
- This is a bullet point
Keyboard Shortcuts: Jupyter Notebook supports various keyboard shortcuts to speed up your workflow. For example, press Esc + A to add a new cell above or Esc + B to add a new cell below.
Interactive Output: Jupyter Notebook allows you to visualize data interactively, which is very useful for data analysis tasks.
Exploring Python Libraries
NumPy: Working with Arrays
NumPy is a powerful library for numerical computations in Python. It provides support for arrays, which are more efficient than Python lists for numerical operations.
import numpy as np
# Creating arrays
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Basic operations
print(arr + 2) # Output: [3 4 5 6 7]
print(arr * 2) # Output: [ 2 4 6 8 10]
Pandas: Data Manipulation
Pandas is a library for data manipulation and analysis. It provides data structures like Series and DataFrame for handling structured data.
import pandas as pd
# Creating a DataFrame
data = {
"Name": ["Alice", "Bob", "Charlie"],
"Age": [25, 30, 35]
}
df = pd.DataFrame(data)
print(df)
# Reading data from a CSV file
df = pd.read_csv("data.csv")
print(df.head())
Matplotlib: Data Visualization
Matplotlib is a plotting library for creating static, animated, and interactive visualizations in Python.
import matplotlib.pyplot as plt
# Creating a simple plot
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.title("Simple Plot")
plt.show()
Conclusion
In this guide, we covered the basics of setting up Python, working with different data types, and performing basic operations. We also introduced some essential Python libraries for data science. This is just the beginning of your Python programming journey. As we progress, we will delve deeper into more advanced topics like functions, exception handling, classes, and more.
Remember, the key to mastering Python is practice. Make sure to follow along with the examples and try out the code on your own. In the next part of this series, we will explore more about Python data structures like lists, dictionaries, tuples, and sets.
Stay tuned for more exciting content, and happy coding!
Thank you for reading! If you found this post helpful, please share it with your friends and family. Happy coding!
Comments