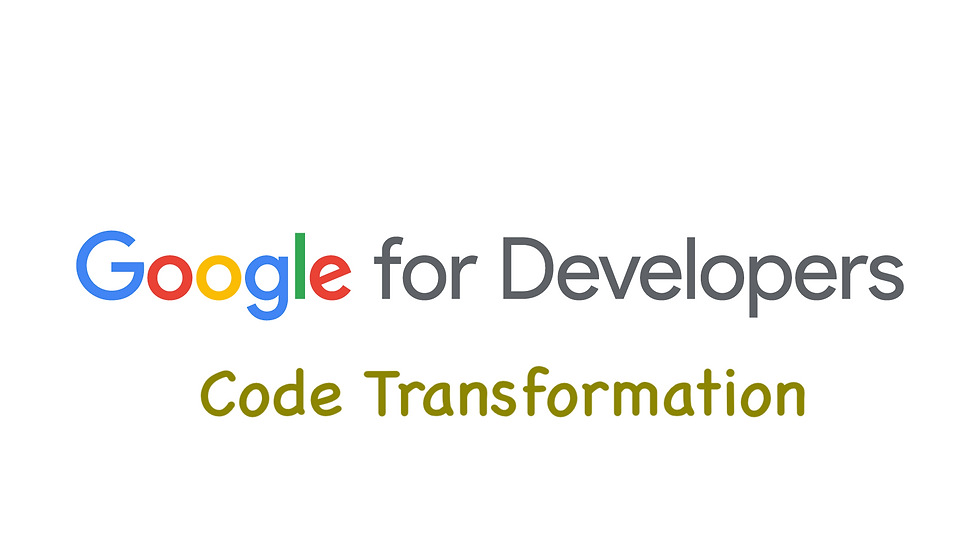
Welcome to another deep dive into the world of AI tools! Today, we're focusing on a remarkable tool from Google that's making waves in the programming community: Google's Code Transformer. This tool is a game-changer for anyone who writes code, especially those who work with Python. Whether you're a seasoned developer or a coding newbie, Code Transformer can help you improve your code quality and efficiency. Let’s dive in and explore what makes this tool so special.
What is Code Transformer?
Code Transformer is an AI-powered tool designed to edit and optimize existing code. It takes in code context along with natural language instructions and generates a code diff—a representation of the changes made to the original code. Currently optimized for Python, it can perform a variety of tasks such as:
Documenting Code: Automatically generates documentation for your code, making it easier to understand.
Reducing Code Nesting: Simplifies complex, nested code structures.
Cleaning Up Code: Fixes errors and optimizes code for better readability and performance.
Completing Code: Generates missing parts of code based on comments or incomplete snippets.
The tool is still experimental and not yet used in production environments, but it's fully functional and free to use without any apparent limitations.
Features and Capabilities
Documenting Code
One of the standout features of Code Transformer is its ability to generate documentation. For example, if you have a complex function that lacks proper documentation, Code Transformer can add meaningful comments and descriptions to help others (or even future you) understand what the code does.
Reducing Code Nesting
Code Transformer can take messy, deeply nested code and transform it into a more streamlined version. This not only makes the code more readable but also easier to maintain and debug.
Cleaning Up Code
Let's say you have a piece of code that has multiple issues, such as redundant conditions or inefficient loops. Code Transformer can analyze the code and suggest fixes, making it more efficient and readable.
Completing Code
If you have an incomplete code snippet or a function with comments indicating what needs to be done, Code Transformer can generate the missing parts for you. This can be incredibly useful when you're stuck or pressed for time.
Real-World Example
Let's look at a practical example to understand how Code Transformer works. Suppose you have the following messy Python code:
def process_data(data_set):
if data_set == 'animal':
if data_set == 'turtle':
return 'Turtle data processed'
if data_set == 'formalTurtle':
return 'Formal Turtle data processed'
else:
if data_set == 'plant':
return 'Plant data processed'
This code has several issues, including redundant conditions and unnecessary nesting. By asking Code Transformer to clean up this code, it might output something like this:
def process_data(data_set):
if data_set == 'turtle':
return 'Turtle data processed'
elif data_set == 'formalTurtle':
return 'Formal Turtle data processed'
elif data_set == 'plant':
return 'Plant data processed'
elif data_set == 'animal':
return 'Animal data processed'
As you can see, the tool has removed unnecessary nesting and made the code more readable and efficient.
Using the Code Transformer Playground
To see Code Transformer in action, you can use their online playground. Here’s a step-by-step guide on how to use it:
Open the Playground: Navigate to the Code Transformer playground.
Default Program: You’ll see a default program loaded in the editor.
Add Documentation: Ask the tool to add documentation to understand what the function does.
Clean Up Code: Request the tool to clean up the messy code.
Complete Code: Provide an incomplete code snippet with comments and let the tool generate the missing parts.
Example: Adding Documentation
Here’s a messy Depth-First Search (DFS) function without documentation:
def dfs(graph, start):
visited = set()
def _dfs(v):
if v not in visited:
visited.add(v)
for neighbor in graph[v]:
_dfs(neighbor)
_dfs(start)
return visited
Ask Code Transformer to add documentation:
def dfs(graph, start):
"""
Perform a Depth-First Search (DFS) on the graph starting from the given node.
Args:
graph (dict): A dictionary representing the adjacency list of the graph.
start (any): The starting node for the DFS.
Returns:
set: A set of visited nodes.
"""
visited = set()
def _dfs(v):
if v not in visited:
visited.add(v)
for neighbor in graph[v]:
_dfs(neighbor)
_dfs(start)
return visited
Example: Completing Code
Here’s an incomplete code snippet with comments:
def print_numbers(x):
# Print numbers from 1 to x
pass
Ask Code Transformer to complete it:
def print_numbers(x):
for i in range(1, x + 1):
print(i)
Example: Cleaning Up Code
Here’s an unoptimized Fibonacci sequence generator:
def fib(n):
a,b,i=0,1,0
while i<n:
print(a)
a,b=b,a+b
i+=1
Ask Code Transformer to clean it up:
def fib(n):
a, b = 0, 1
for _ in range(n):
print(a)
a, b = b, a + b
Conclusion
Google's Code Transformer is an incredibly powerful and versatile tool that can help you write cleaner, more efficient code. Whether you need to document, optimize, clean up, or complete your code, Code Transformer has got you covered. Give it a try and see how it can enhance your coding workflow by visiting their online playground.
Thank you for reading! If you found this post helpful, don't forget to share it with your fellow developers. Happy coding!
Comments