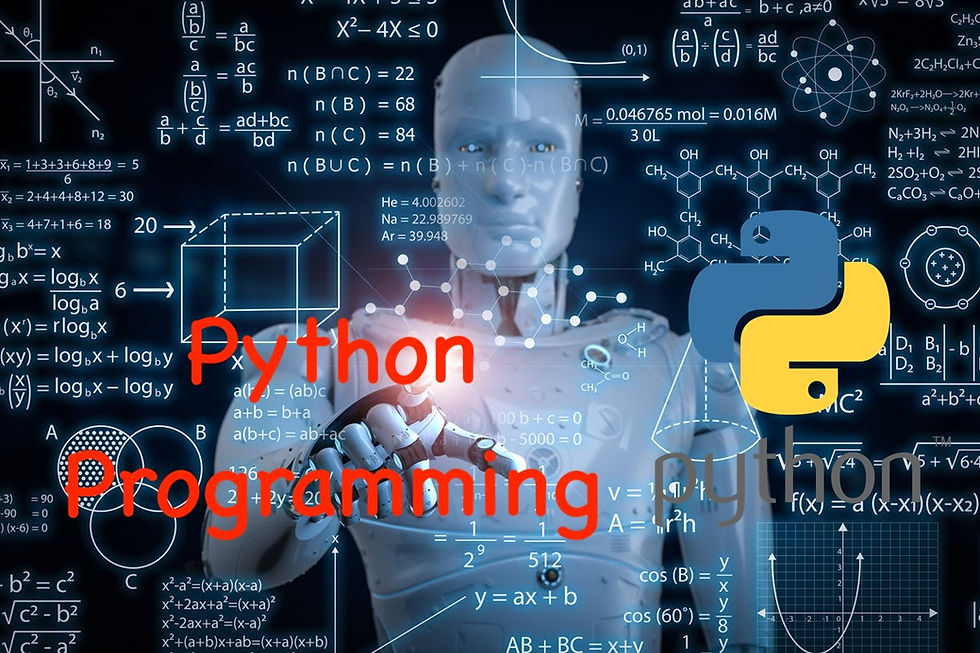
Hello, young coders! Today we're going to explore an exciting way to make your text in Python look exactly how you want it to. This magical trick is called string formatting. It helps you create complex strings with placeholders that get filled dynamically with values. Ready to learn something cool? Let's dive right in!
Why String Formatting?
Imagine you want to greet your friends by name. Instead of writing a new message for each friend, you can use string formatting to create a template that fills in each name automatically. String formatting makes your code cleaner, easier to read, and more flexible.
Basic String Printing
First, let's look at how you normally print a simple message in Python.
print("Hello, world!")
You can also store this message in a variable and print it:
message = "Hello, everyone!"
print(message)
Introducing Placeholders
Sometimes you want parts of your message to change dynamically. For example, you might want to greet different people by name. This is where placeholders come in. Placeholders are special spots in your string that get replaced with actual values when you print the string.
Using the format Method
The format method is a handy way to fill in placeholders. Let's see how it works:
Example 1: Simple Greeting
def greeting(name):
return "Hello, {}! Welcome to the community.".format(name)
# Using the function
print(greeting("Revanth"))
In this example:
We define a function greeting that takes a name as an argument.
Inside the function, we create a string with {} as a placeholder.
We use .format(name) to replace the placeholder with the actual name.
Example 2: Complex Message
Now let's create a more complex message with multiple placeholders:
def welcome_message(first_name, age):
return "Welcome, {}. You are {} years old.".format(first_name, age)
# Using the function
print(welcome_message("Revanth", 12))
Here, we:
Define a function welcome_message that takes first_name and age as arguments.
Create a string with two placeholders {}.
Use .format(first_name, age) to replace the placeholders with the actual values in the correct order.
Using Named Placeholders
To make sure the placeholders are filled correctly, you can use named placeholders. This way, the order of values doesn't matter:
Example 3: Named Placeholders
def detailed_welcome(first_name, age):
return "Hello, {name}. You are {age} years old.".format(name=first_name, age=age)
# Using the function
print(detailed_welcome("Revanth", 12))
In this example:
We use {name} and {age} as named placeholders.
Inside .format(), we specify name=first_name and age=age to match the placeholders.
Common Errors
If you forget to provide a value for each placeholder, Python will give you an error. For example:
def incomplete_message(name):
return "Hello, {}. Welcome!".format(name)
# Using the function
print(incomplete_message())
This code will cause an error because we didn't provide a value for name.
Conclusion
String formatting in Python is a powerful tool that lets you create flexible and dynamic text. Whether you're greeting friends, displaying information, or creating complex messages, string formatting makes your code cleaner and more efficient.
Here's a quick summary of what we've learned:
Use {} as placeholders in your strings.
Replace placeholders with actual values using the .format() method.
Use named placeholders to avoid errors and make your code clearer.
I hope you enjoyed this fun guide to string formatting in Python. Practice using these tips, and soon you'll be creating amazing text in your programs. Happy coding, and see you next time!
Comments