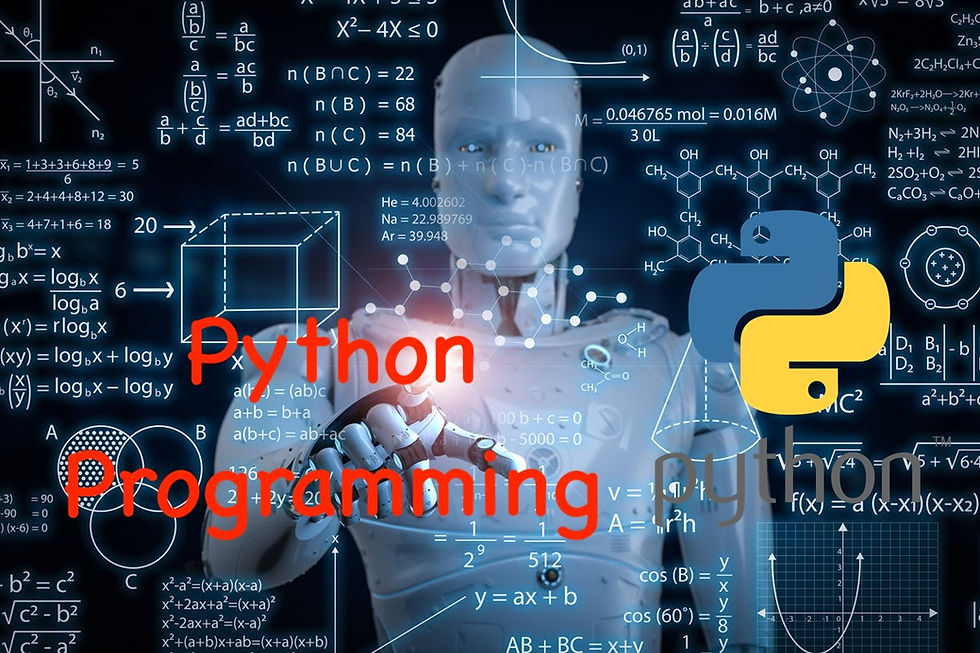
Hello everyone! Today, we're going to talk about an exciting topic in Python—lambda functions! If you've ever wondered what lambda functions are and why they can be beneficial, you're in the right place. We'll break it down step-by-step, using simple and easy-to-understand examples. By the end of this post, you'll be a lambda function pro!
What Are Lambda Functions?
Lambda functions in Python are also known as anonymous functions. Unlike regular functions, lambda functions do not have a name. They are used to create small, one-time, and quick functions without having to formally define a function using the def keyword.
Why Use Lambda Functions?
Lambda functions are particularly useful when you need a short function for a specific task and you don't want to formally define a function using def. They are faster to write and can make your code cleaner.
Creating a Simple Function
Let's start with a simple example. We'll create a regular function that adds two numbers.
def addition(a, b):
return a + b
# Using the function
print(addition(4, 5)) # Output: 9
This function takes two parameters, a and b, and returns their sum. Now, let's see how to convert this into a lambda function.
Converting to a Lambda Function
To convert the above function into a lambda function, we use the lambda keyword, followed by the parameters and the expression.
# Lambda function
addition = lambda a, b: a + b
# Using the lambda function
print(addition(4, 5)) # Output: 9
In this example:
lambda a, b: specifies that the function takes two parameters, a and b.
a + b is the expression that the lambda function will execute.
Notice how compact the lambda function is compared to the regular function.
More Examples
Checking If a Number Is Even
Let's create a function that checks if a number is even.
# Regular function
def is_even(num):
return num % 2 == 0
print(is_even(24)) # Output: True
Now, let's convert it into a lambda function.
# Lambda function
is_even = lambda a: a % 2 == 0
print(is_even(24)) # Output: True
Adding Three Numbers
Let's create a function that adds three numbers.
# Regular function
def add_three_numbers(x, y, z):
return x + y + z
print(add_three_numbers(2, 3, 4)) # Output: 9
Now, let's convert it into a lambda function.
# Lambda function
add_three_numbers = lambda x, y, z: x + y + z
print(add_three_numbers(2, 3, 4)) # Output: 9
Multiplying Three Numbers
We can also create a lambda function for multiplying three numbers.
# Lambda function
multiply_three_numbers = lambda x, y, z: x * y * z
print(multiply_three_numbers(2, 3, 4)) # Output: 24
Important Points to Remember
Single Expression: Lambda functions can only contain a single expression. If your function has multiple lines of code or complex logic, you should stick to using the def keyword.
Anonymous: Lambda functions do not have a name. They are often used as arguments to higher-order functions (functions that take other functions as arguments).
Quick and Simple: Lambda functions are best used for small tasks that are simple and quick to write.
When to Use Lambda Functions
Lambda functions are perfect for:
Short, throwaway functions that you will use only once.
Situations where you want to define a function in a concise way.
Small utility functions that are used as arguments to higher-order functions like map(), filter(), and reduce().
Conclusion
Lambda functions are a powerful feature in Python that can make your code more concise and readable. They are perfect for small, simple tasks that require a quick function definition. Remember, lambda functions can only contain a single expression and are best used for short, one-time functions.
I hope you found this guide helpful and easy to understand. Stay tuned for our next session, where we'll dive into list comprehensions and other exciting Python concepts. Until then, happy coding!
Comments