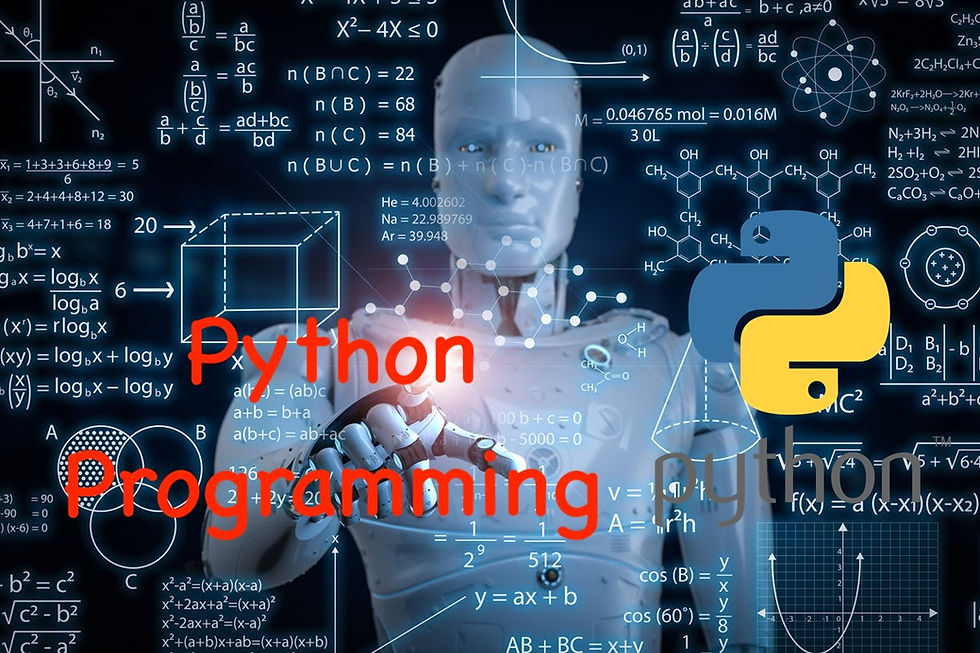
Hello, young coders! Welcome to a fun and exciting lesson on Python's iterables and iterators. These might sound like complicated terms, but don't worry, we'll break them down into simple, easy-to-understand pieces. Let's embark on this coding adventure together!
What Are Iterables?
First things first, let's talk about iterables. Simply put, an iterable is any Python object that you can loop over (or iterate through). A list is a perfect example of an iterable. Let's see how it works with a simple example:
Example: Iterating Through a List
# Creating a list
numbers = [1, 2, 3, 4, 5, 6, 7]
# Using a for loop to iterate through the list
for num in numbers:
print(num)
In this example:
We create a list called numbers with some values.
We use a for loop to go through each item in the list and print it.
Since we can loop through the list, we call it an iterable. Easy, right?
Introducing Iterators
Now, let's move on to iterators. An iterator is a special object that allows you to traverse through all the elements of an iterable, but it does so one element at a time. This might sound similar to a for loop, but there's a key difference.
Example: Converting a List to an Iterator
You can convert an iterable (like a list) into an iterator using the iter() function. Here's how:
# Converting the list into an iterator
numbers_iterator = iter(numbers)
print(numbers_iterator)
When you run this code, you'll see something like <list_iterator object at 0x...>. This shows that numbers_iterator is now an iterator.
Using the next() Function
To retrieve elements from an iterator, we use the next() function. Let's see it in action:
Example: Retrieving Elements from an Iterator
# Getting elements one by one using next()
print(next(numbers_iterator)) # Output: 1
print(next(numbers_iterator)) # Output: 2
print(next(numbers_iterator)) # Output: 3
Each time we call next(), it retrieves the next element in the iterator. Unlike a list, the iterator only gives you one element at a time.
Why Use Iterators?
You might wonder why we need iterators when we have lists. Here’s why: Iterators are more memory efficient. They don't store all the elements in memory at once. Instead, they generate each element only when you need it.
Imagine you have a list with millions of elements. Storing all of them in memory at once can be very inefficient. Instead, you can use an iterator to handle this data more efficiently.
Using Iterators in a Loop
Just like iterables, you can also use iterators in a loop. Here's how:
Example: Looping Through an Iterator
# Converting the list into an iterator again
numbers_iterator = iter(numbers)
# Using a for loop to go through the iterator
for num in numbers_iterator:
print(num)
This will print all the numbers in the list, one by one.
Handling StopIteration Error
When you reach the end of an iterator, calling next() again will raise a StopIteration error. This error is automatically handled when you use a for loop, but it's good to know what’s happening behind the scenes.
Example: Handling StopIteration
# Converting the list into an iterator again
numbers_iterator = iter(numbers)
try:
while True:
print(next(numbers_iterator))
except StopIteration:
print("End of iterator reached")
In this code:
We use a while True loop to keep calling next().
When we reach the end of the iterator, a StopIteration exception is raised.
We catch this exception and print a message.
Conclusion
Iterables and iterators are powerful tools in Python that help you work with data efficiently. Here’s a quick summary of what we’ve learned:
Iterables: Objects you can loop through, like lists.
Iterators: Objects that let you traverse through an iterable one element at a time.
iter() function: Converts an iterable into an iterator.
next() function: Retrieves the next element from an iterator.
Efficiency: Iterators are memory efficient, especially for handling large data sets.
I hope you enjoyed this fun guide to iterables and iterators in Python. Practice using these tips, and soon you'll be managing data like a pro. Happy coding, and see you next time!
Comments