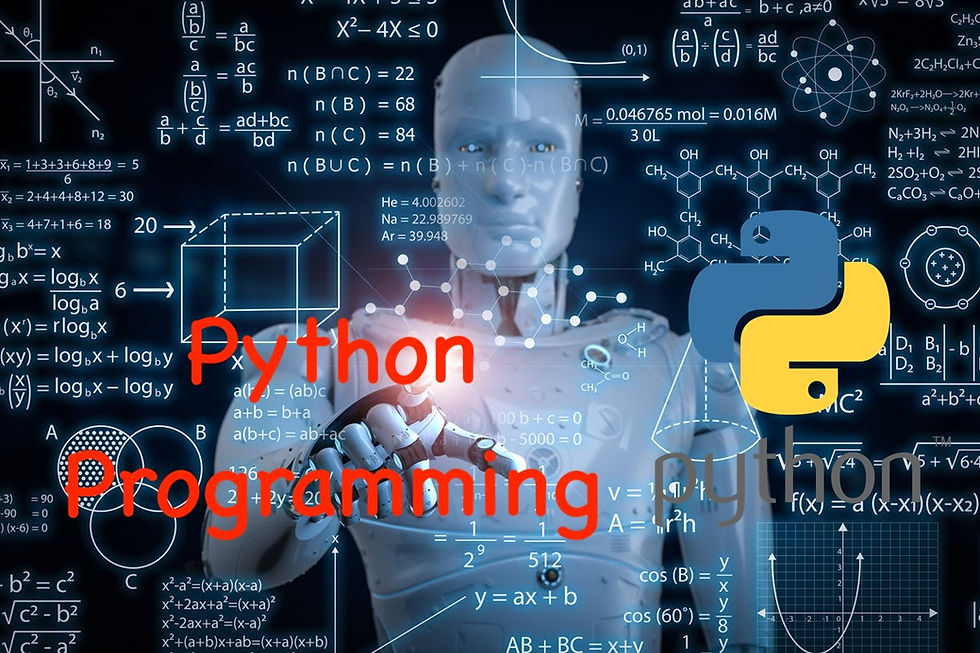
Hello young Python enthusiasts! Welcome to another exciting tutorial on advanced Python topics. Today, we will dive into two important concepts: Assertions and Exception Handling. These concepts help make your code more robust and error-free. By the end of this blog, you'll be able to write safer and more reliable programs. Let's get started!
What Are Assertions?
Assertions are like little checkpoints in your code. They help you ensure that certain conditions hold true while your program runs. If an assertion fails, it means something went wrong, and Python will stop the program to alert you.
How Do Assertions Work?
An assertion is written using the assert statement followed by a condition. If the condition is true, the program continues. If it's false, the program stops, and an error message is displayed.
Here's a simple example:
def check_even(number):
assert number % 2 == 0, "The number is not even!"
print(f"{number} is an even number.")
check_even(4) # This will pass
check_even(3) # This will fail
Explanation:
Condition: number % 2 == 0 checks if the number is even.
Message: "The number is not even!" is displayed if the assertion fails.
What Is Exception Handling?
Exception handling is a way to manage errors in your code. Instead of letting your program crash, you can catch errors and handle them gracefully.
How Do You Handle Exceptions?
Python provides try, except, else, and finally blocks to handle exceptions.
Example:
Let's write a program that asks the user to enter an even number. If the user enters an odd number, we'll handle the error.
try:
number = int(input("Enter an even number: "))
assert number % 2 == 0, "The number is not even!"
except ValueError:
print("That's not a valid number!")
except AssertionError as e:
print(e)
else:
print(f"{number} is an even number.")
finally:
print("Thank you for using the program.")
Explanation:
try block: This is where you write the code that might raise an error.
except block: This catches and handles specific errors. In this case, we handle ValueError (if the input is not a number) and AssertionError (if the number is not even).
else block: This runs if no exceptions are raised.
finally block: This always runs, no matter what. It's a good place to clean up resources or say goodbye to the user.
Putting It All Together
Let's combine assertions and exception handling in a fun program that checks if a number is even and handles any errors gracefully.
Example:
def check_even_number():
try:
number = int(input("Enter an even number: "))
assert number % 2 == 0, "The number is not even!"
except ValueError:
print("That's not a valid number! Please enter an integer.")
except AssertionError as e:
print(e)
else:
print(f"Great job! {number} is an even number.")
finally:
print("Thank you for participating!")
# Run the function
check_even_number()
Explanation:
Function Definition: We define a function check_even_number() that performs our task.
try block: We ask the user to input a number and check if it's even using an assertion.
except blocks: We handle both ValueError and AssertionError.
else block: We congratulate the user if no errors occur.
finally block: We thank the user for participating, no matter what happens.
Conclusion
Assertions and exception handling are powerful tools that help you write safer and more reliable code. Assertions act as checkpoints to ensure your program runs correctly, while exception handling allows you to manage errors gracefully.
Keep practicing these concepts, and soon you'll be writing robust Python programs with ease. Stay tuned for more exciting tutorials, and happy coding!
If you found this tutorial helpful, don't forget to share it with your friends and keep learning. Thank you for joining us today, and see you in the next tutorial!
Commenti