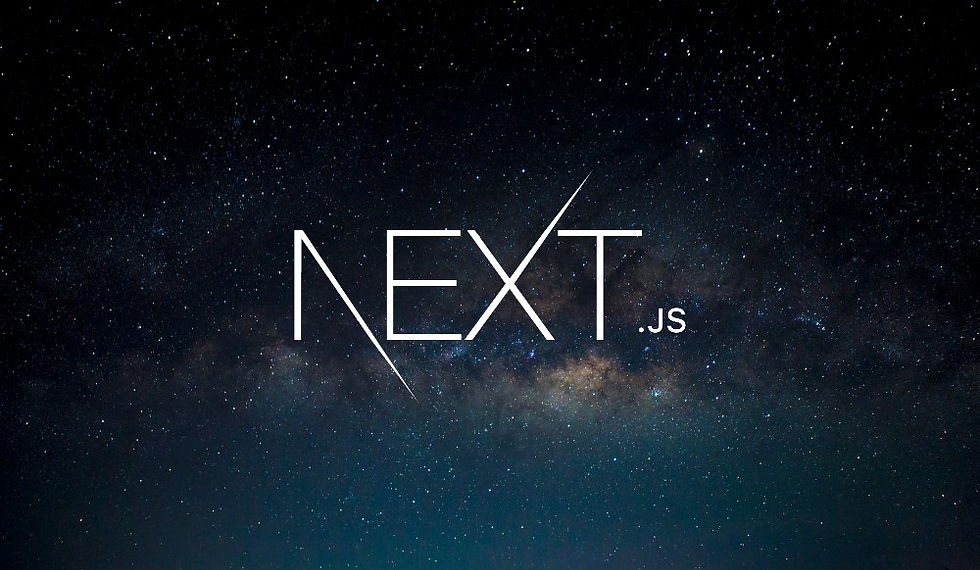
Creating a well-structured and efficient layout is a crucial aspect of building a web application. In this blog post, we'll explore how to set up a basic layout and navigation using Next.js, focusing on the Link component for internal navigation and the nuances of rendering different parts of your application.
Setting Up a Simple Navigation Bar
A navigation bar is essential for guiding users through your website. In Next.js, we use the Link component to enable client-side navigation, which provides a seamless transition between pages without reloading the entire page.
Using the Link Component
The Link component in Next.js should be used for navigating within your application. This ensures that only the necessary parts of the page are updated, making the navigation faster and smoother.
Here's an example of a simple navigation bar using the Link component:
import Link from 'next/link';
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>
<nav>
<ul>
<li><Link href="/">Home</Link></li>
<li><Link href="/reviews">Reviews</Link></li>
<li><Link href="/about">About</Link></li>
</ul>
</nav>
</header>
<main>{children}</main>
<footer>
Game data and images courtesy of <a href="https://rawg.io/" target="_blank" rel="noopener noreferrer">RAWG</a>
</footer>
</body>
</html>
);
}
External Links
For external links, there's no need to use the Link component. Instead, a regular anchor (<a>) element works perfectly. You can also set the target attribute to _blank to open the link in a new tab.
In our example, we have a footer with a link to an external website:
<footer>
Game data and images courtesy of <a href="https://rawg.io/" target="_blank" rel="noopener noreferrer">RAWG</a>
</footer>
The rel="noopener noreferrer" attribute is added for security reasons, preventing the new page from gaining access to the window.opener property.
Understanding the Layout Component
In Next.js, the layout component is not just a convenient way to share common elements (like headers and footers) across pages. It also plays a crucial role in how pages are rendered when navigating from one route to another.
Rendering Efficiency
When navigating between pages, Next.js only re-renders the page-specific elements inside the main element. The header and footer remain unchanged, which is more efficient and provides a better user experience.
Here's a detailed look at how this works:
Initial Load: When the application loads, the entire layout, including the header, main content, and footer, is rendered.
Navigation: When navigating to a different page (e.g., from Home to Reviews), only the content inside the main element is updated. The header and footer stay the same.
You can observe this behavior using your browser's DevTools. When you click on a link, you'll notice that only the main element flashes, indicating that it was updated, while the header and footer remain static.
Example Navigation
Let's take a closer look at what happens when we navigate from the Home page to the Reviews page:
Home Page: The initial load renders the entire layout, including the header, main content, and footer.
Click "Reviews": Only the content inside the main element is updated to display the Reviews page content.
import Link from 'next/link';
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>
<nav>
<ul>
<li><Link href="/">Home</Link></li>
<li><Link href="/reviews">Reviews</Link></li>
<li><Link href="/about">About</Link></li>
</ul>
</nav>
</header>
<main>{children}</main>
<footer>
Game data and images courtesy of <a href="https://rawg.io/" target="_blank" rel="noopener noreferrer">RAWG</a>
</footer>
</body>
</html>
);
}
Deciding Where to Place Elements
When designing your application, consider which elements should be part of the layout and which should be page-specific. Generally, elements that are common across multiple pages, such as headers, footers, and sidebars, should be included in the layout component. This minimizes re-rendering and enhances performance.
Conclusion
By effectively using the layout component and the Link component in Next.js, you can create a more efficient and user-friendly web application. The layout component helps maintain a consistent structure across your pages and optimizes the rendering process, while the Link component ensures smooth client-side navigation.
Remember to use regular anchor elements for external links and consider the placement of your elements carefully to enhance the overall performance and user experience of your application.
Comments