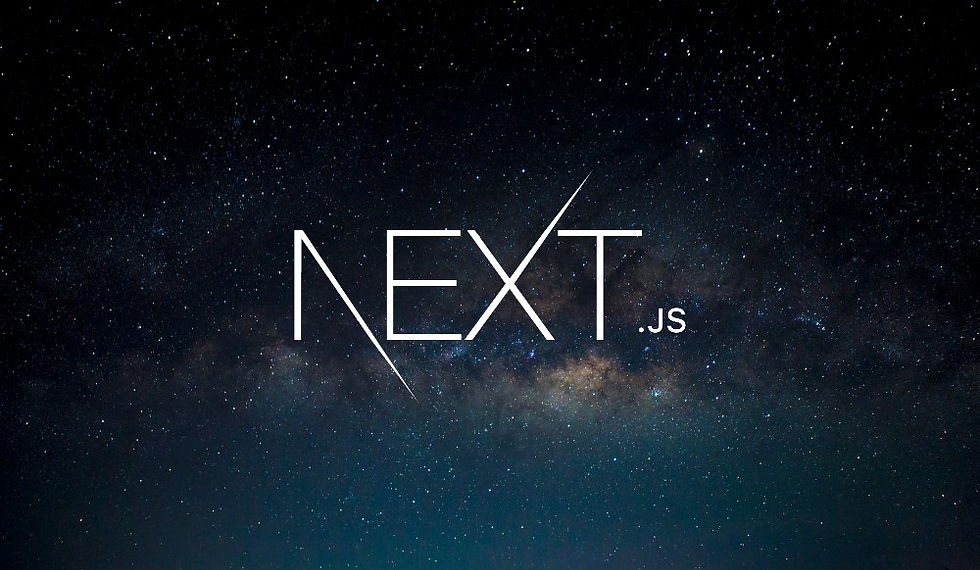
In this blog post, we'll walk you through a quick exercise to add navigation links for individual game reviews on a Reviews page. This exercise will help you understand how to use the Link component in Next.js to create internal links that facilitate client-side navigation. By the end of this post, you'll have a clear idea of how to structure your links and optimize your navigation.
Step-by-Step Exercise: Adding Review Links
Setting the Scene
We already have a basic navigation setup in our header, visible across all pages of our website. Now, our goal is to add a list of links on the Reviews page, each pointing to a specific game review.
The Task
Add an unordered list to the Reviews page.
Create a list item for each game review.
Use the Link component to ensure smooth client-side navigation.
Let's Code
Here's how you can achieve this:
1. Import the Link Component: First, ensure you have the Link component imported from Next.js.
import Link from 'next/link';
2. Create the Reviews Page Component: Next, structure your Reviews page component to include an unordered list (<ul>) with list items (<li>) for each game review.
export default function ReviewsPage() {
return (
<>
<h1>Reviews</h1>
<p>Here we'll list all the reviews.</p>
<ul>
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</ul>
</>
);
}
Understanding the Code
Header: The <h1> and <p> elements provide the heading and a brief description for the Reviews page.
Unordered List: The <ul> element contains multiple <li> elements, each representing a review link.
Link Component: The Link component from Next.js is used to wrap the game titles. The href attribute specifies the path to the individual review pages.
Testing the Links
Once you have added the links, navigate to the Reviews page in your browser. You should see a list of game titles. Clicking on a game title will take you to the corresponding review page.
Hollow Knight: Clicking on this link should navigate to /reviews/hollow-knight.
Stardew Valley: Clicking on this link should navigate to /reviews/stardew-valley.
Client-Side Navigation
When you click on a link, you'll notice that the navigation is smooth and doesn't cause a full page reload. This is because the Link component utilizes client-side navigation, improving the user experience.
Going Deeper: Prefetching
Next.js automatically prefetches linked pages in the background, enhancing the speed of subsequent navigation. You can inspect network requests using browser Developer Tools to see how prefetching works.
Open Chrome Developer Tools: Right-click on the page and select "Inspect" or press Ctrl+Shift+I (Cmd+Option+I on Mac).
Network Tab: Navigate to the "Network" tab to observe network requests.
Navigate to Reviews: Start from the home page and navigate to the Reviews page.
Inspect Prefetching: Notice how Next.js prefetches the additional linked pages (Hollow Knight and Stardew Valley reviews).
Conclusion
This exercise demonstrates how to add navigation links for individual reviews using the Link component in Next.js. By following these steps, you can create a well-structured Reviews page with efficient client-side navigation. Additionally, exploring prefetching helps you understand how Next.js optimizes performance behind the scenes.
With this knowledge, you can further enhance your web application by adding more reviews and links, improving the overall user experience. Happy coding!
Comments