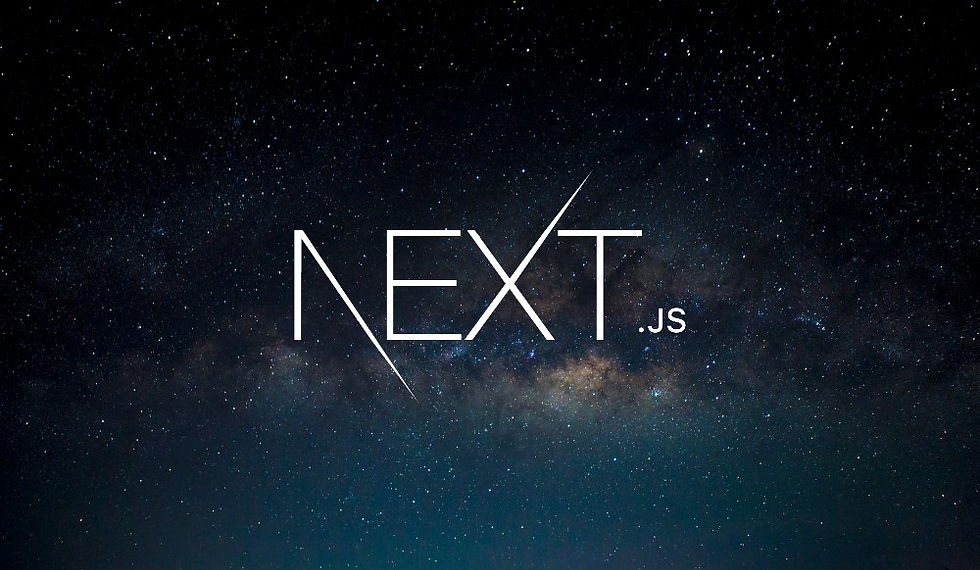
Styling your Next.js application is a crucial step in creating a visually appealing and user-friendly website. Next.js provides several options for styling, ranging from traditional CSS to modern CSS-in-JS solutions. In this blog post, we'll explore these options, ensuring that you can easily understand and apply them in your projects.
Global CSS
Step 1: Creating a Global CSS File
The simplest way to add styles that apply to your entire application is by using a global CSS file. For example, let's create a file called globals.css. This file will contain all the CSS rules that should be applied globally.
globals.css:
body {
font-family: sans-serif;
}
In this example, we set the font-family for the entire body to sans-serif, which will use the default sans-serif font on each system.
Step 2: Importing the Global CSS File
To apply these global styles, you need to import the globals.css file in your layout file. Since the RootLayout component applies to all pages, it's the perfect place for this import.
app/layout.jsx:
import Link from 'next/link';
import './globals.css';
export default function RootLayout({ children }) {
return (
<>
<header>
{/* Navigation links */}
</header>
<main>{children}</main>
</>
);
}
When you save and view your application, you'll notice that the text font has changed globally.
Locally Scoped CSS with CSS Modules
What if you need styles that apply only to specific components? This is where CSS Modules come in handy. CSS Modules provide locally scoped CSS, meaning the styles you define in a CSS file are scoped to the component that imports them.
Step 1: Creating a CSS Module
Create a CSS module file, for example, Reviews.module.css.
Reviews.module.css:
.list {
background-color: lightgray;
padding: 10px;
}
Step 2: Importing and Using the CSS Module
Import the CSS module in your component and apply the styles using a special syntax.
ReviewsPage.jsx:
import styles from './Reviews.module.css';
export default function ReviewsPage() {
return (
<>
<h1>Reviews</h1>
<p>Here we'll list all the reviews.</p>
<ul className={styles.list}>
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</ul>
</>
);
}
CSS-in-JS with Styled Components
Another popular styling solution is CSS-in-JS, where you write your CSS styles directly within your JavaScript code. This approach is often used with libraries like styled-components.
Example with Styled Components
First, install styled-components:
npm install styled-components
Then, create a styled component in your code.
ReviewsPage.jsx:
import styled from 'styled-components';
const List = styled.ul`
background-color: lightgray;
padding: 10px;
`;
export default function ReviewsPage() {
return (
<>
<h1>Reviews</h1>
<p>Here we'll list all the reviews.</p>
<List>
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</List>
</>
}
Utility-First CSS with Tailwind CSS
Tailwind CSS is a utility-first CSS framework that provides a set of predefined classes for building your design directly in your markup.
Step 1: Installing Tailwind CSS
Follow the Tailwind CSS installation guide to set it up in your Next.js project.
Step 2: Using Tailwind CSS
Once installed, you can use Tailwind's utility classes directly in your JSX.
ReviewsPage.jsx:
export default function ReviewsPage() {
return (
<>
<h1 className="text-2xl font-bold">Reviews</h1>
<p className="text-lg">Here we'll list all the reviews.</p>
<ul className="bg-gray-200 p-4">
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</ul>
</>
);
}
Conclusion
Next.js offers a variety of styling solutions, each with its strengths and use cases. Whether you prefer traditional global CSS, locally scoped CSS Modules, CSS-in-JS with styled-components, or utility-first CSS with Tailwind CSS, Next.js has you covered. By understanding and utilizing these options, you can create beautiful and maintainable styles for your application. Happy styling!
Comments