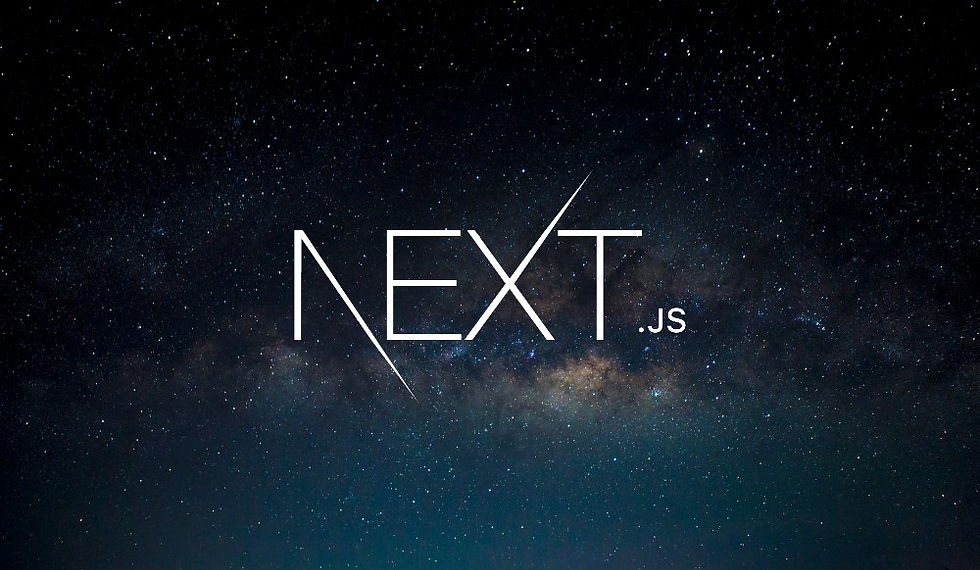
Tailwind CSS is a utility-first CSS framework that allows you to build modern and responsive user interfaces quickly. In this blog post, we will walk through the steps to install and configure Tailwind CSS in your Next.js project.
Step 1: Install Tailwind CSS
To get started, you'll need to install Tailwind CSS along with its dependencies: PostCSS and Autoprefixer. Follow these steps to install the necessary packages:
Open your terminal in your project directory.
Stop the development server if it's running.
Run the following command to install the packages:
npm install --save-dev tailwindcss postcss autoprefixer
The --save-dev option adds the packages to your devDependencies, meaning they are only required during development.
Step 2: Initialize Tailwind CSS
Next, you'll need to initialize Tailwind CSS to create the necessary configuration files. Run the following command:
npx tailwindcss init -p
This command generates two configuration files in your project:
postcss.config.js: This file configures PostCSS to use the Tailwind CSS and Autoprefixer plugins.
tailwind.config.js: This file is used to customize Tailwind's default configuration.
Step 3: Configure Tailwind CSS
Edit tailwind.config.js
Tailwind CSS needs to know where to find your HTML and JavaScript files so it can scan them for class names and include only the styles you use in your final build. Update the content property in tailwind.config.js to include the paths to your files:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./app/**/*.{jsx,tsx}',
'./components/**/*.{jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
};
This configuration tells Tailwind to scan all files with .jsx and .tsx extensions inside the app and components folders.
Edit globals.css
To use Tailwind's utility classes, you need to import Tailwind's base, components, and utilities styles in your global CSS file. Edit your globals.css as follows:
globals.css:
@tailwind base;
@tailwind components;
@tailwind utilities;
This setup ensures that Tailwind's styles are included in your project.
Step 4: Optional - Install a Tailwind CSS Extension for VS Code
If you're using Visual Studio Code, you can enhance your development experience by installing a Tailwind CSS extension. This extension provides code auto-completion for Tailwind's utility classes, making it easier to write and manage your styles.
Open Visual Studio Code.
Go to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window.
Search for "Tailwind CSS" and install the extension.
Step 5: Restart the Development Server
After configuring Tailwind CSS, restart your development server to see the changes:
npm run dev
The first time you start the server, it might take a bit longer as Tailwind compiles the styles. Once the server is running, you can start using Tailwind's utility classes in your Next.js components.
Example: Using Tailwind CSS in a Component
Here’s a quick example of how to use Tailwind CSS to style a component in your Next.js project.
ExampleComponent.jsx:
export default function ExampleComponent() {
return (
<div className="bg-gray-100 p-6 rounded-lg shadow-lg">
<h1 className="text-2xl font-bold text-gray-900">Hello, Tailwind CSS!</h1>
<p className="text-gray-700">This is a simple example of using Tailwind CSS in a Next.js project.</p>
</div>
);
}
In this example, we use Tailwind's utility classes like bg-gray-100, p-6, rounded-lg, and shadow-lg to style the component.
Conclusion
You have now successfully installed and configured Tailwind CSS in your Next.js project. Tailwind CSS provides a powerful and flexible way to style your application with utility-first classes, making it easy to build responsive and consistent designs. Start exploring Tailwind's extensive documentation to learn more about its features and capabilities. Happy coding!
Comments