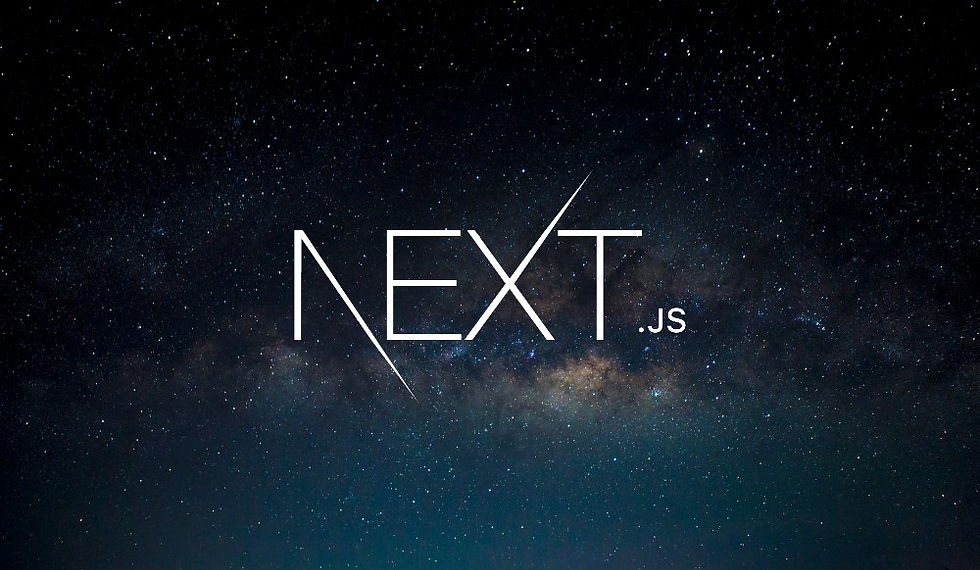
In our previous discussion, we explored how to add colors to our application using Tailwind CSS. Now, let's focus on styling the main content of our pages, starting with the heading text. To ensure consistency and avoid code duplication, we'll create a reusable Heading component in React.
Styling the Heading Text
First, let's add some initial styles to our heading text on the Home page to make it stand out.
Example:
<h1 className="font-bold text-2xl pb-3">Indie Gamer</h1>
font-bold: Makes the text bold.
text-2xl: Sets the text size to extra-large.
pb-3: Adds padding to the bottom, creating space between the heading and the following paragraph.
While this styling works for the Home page, we want to apply the same styles to headings on other pages without duplicating code. To achieve this, we'll extract the heading code into a reusable component.
Creating the Heading Component
Let's create a new file called Heading.jsx inside a components folder. This component will encapsulate the common styles for our headings.
Step-by-Step:
Create the Heading.jsx file:
// components/Heading.jsx
export default function Heading({ children }) {
return (
<h1 className="font-bold text-2xl pb-3">
{children}
</h1>
);
}
Here, the Heading component takes children as a prop, allowing us to pass different text values into the h1 element.
Update the Home Page to Use the Heading Component:
// app/page.jsx
import Heading from '../components/Heading';
export default function HomePage() {
return (
<>
<Heading>Indie Gamer</Heading>
<p>
Only the best indie games, reviewed for you.
</p>
</>
);
}
By replacing the h1 tag with our Heading component, we ensure the heading on the Home page has the correct styles.
Applying the Heading Component Across Pages
To maintain consistency, we need to use the Heading component on other pages as well. We'll follow a similar approach for each page.
Updating the About Page:
// app/about/page.jsx
import Heading from '../../components/Heading';
export default function AboutPage() {
return (
<>
<Heading>About</Heading>
<p>
A website created to learn Next.js.
</p>
</>
);
}
Updating the Reviews Page:
// app/reviews/page.jsx
import Heading from '../../components/Heading';
import Link from 'next/link';
export default function ReviewsPage() {
return (
<>
<Heading>Reviews</Heading>
<ul>
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</ul>
</>
);
}
Updating Individual Review Pages:
// app/reviews/hollow-knight/page.jsx
import Heading from '../../../components/Heading';
export default function HollowKnightPage() {
return (
<>
<Heading>Hollow Knight</Heading>
<p>
This will be the review for Hollow Knight.
</p>
</>
);
}
// app/reviews/stardew-valley/page.jsx
import Heading from '../../../components/Heading';
export default function StardewValleyPage() {
return (
<>
<Heading>Stardew Valley</Heading>
<p>
This will be the review for Stardew Valley.
</p>
</>
);
}
Summary
By creating a reusable Heading component, we've ensured that our heading styles are consistent across all pages. This approach not only reduces code duplication but also makes it easier to update the heading styles in the future. If we decide to change the heading style, we only need to modify the Heading.jsx file, and the changes will be reflected across all pages.
This technique of extracting common styles into reusable components is a powerful way to maintain clean and manageable code, especially in larger projects.
Stay tuned as we continue to enhance the styling and functionality of our application using Tailwind CSS and React! Happy coding!
Comments