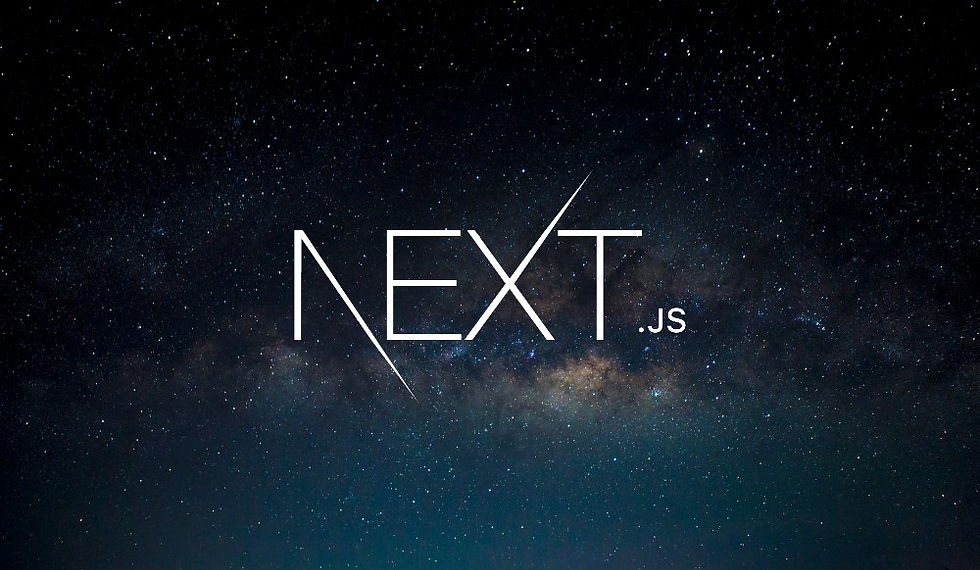
In our previous discussion, we created a custom Heading component to apply consistent CSS styles across all the headings in our project. However, as our project grows and files get nested deeper, managing import paths can become cumbersome. This is where import aliases come to the rescue.
The Problem with Relative Import Paths
Consider the following import statements:
// app/page.jsx
import Heading from '../components/Heading';
// app/reviews/page.jsx
import Heading from '../../components/Heading';
// app/reviews/hollow-knight/page.jsx
import Heading from '../../../components/Heading';
As we can see, the deeper the file is nested, the longer and messier the relative import paths become. This complexity can lead to errors, especially when moving files around. To solve this, we can configure an import alias.
Configuring an Import Alias
In a Next.js project, setting up an import alias is straightforward. We'll use a jsconfig.json file for JavaScript projects or tsconfig.json for TypeScript projects.
Creating the Configuration File
Create jsconfig.json at the root of your project:
{
"compilerOptions": {
"paths": {
"@/*": ["./*"]
}
}
}
This configuration tells the compiler that whenever it sees an import path starting with @/, it should treat it as relative to the root of the project.
Applying the Import Alias
Let's update our import statements to use the alias we just configured.
Home Page:
// app/page.jsx
import Heading from '@/components/Heading';
export default function HomePage() {
return (
<>
<Heading>Indie Gamer</Heading>
<p>
Only the best indie games, reviewed for you.
</p>
</>
);
}
Reviews Page:
// app/reviews/page.jsx
import Heading from '@/components/Heading';
import Link from 'next/link';
export default function ReviewsPage() {
return (
<>
<Heading>Reviews</Heading>
<ul>
<li>
<Link href="/reviews/hollow-knight">Hollow Knight</Link>
</li>
<li>
<Link href="/reviews/stardew-valley">Stardew Valley</Link>
</li>
</ul>
</>
);
}
Individual Review Pages:
// app/reviews/hollow-knight/page.jsx
import Heading from '@/components/Heading';
export default function HollowKnightPage() {
return (
<>
<Heading>Hollow Knight</Heading>
<p>
This will be the review for Hollow Knight.
</p>
</>
);
}
// app/reviews/stardew-valley/page.jsx
import Heading from '@/components/Heading';
export default function StardewValleyPage() {
return (
<>
<Heading>Stardew Valley</Heading>
<p>
This will be the review for Stardew Valley.
</p>
</>
);
}
Benefits of Using Import Aliases
Cleaner Code: The import paths are now concise and consistent across all files, regardless of their location in the project structure.
Easier Refactoring: Moving files around the project won't break the import paths, making refactoring a breeze.
Improved Readability: It’s easier to understand where a module is coming from when using a consistent alias.
Simplified Copying: Copying imports from one file to another becomes straightforward, as the paths don’t change.
Final Thoughts
Configuring import aliases in your Next.js project is a simple yet powerful way to keep your codebase clean and maintainable. By setting up an alias like @/, you create a more intuitive way to manage your imports, which is especially beneficial as your project scales.
Remember, if you use the Create Next App tool to initialize your Next.js project, it will automatically configure the path alias for you. However, understanding how to set it up manually is valuable knowledge that can help you in various projects.
Happy coding! Stay tuned for more tips and tricks to improve your development workflow.
Comentários