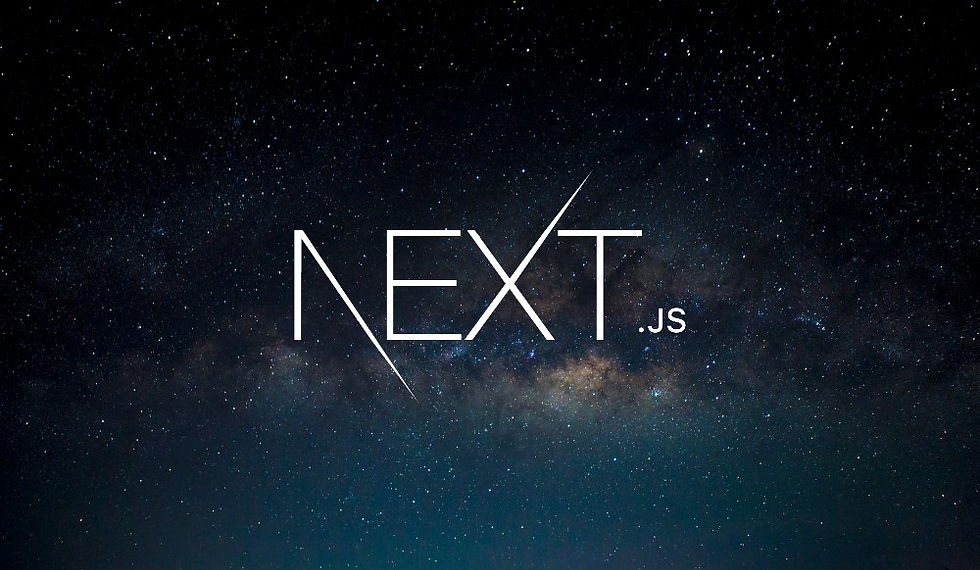
Our website is starting to look better thanks to some styling, but it's still quite plain. One way to make it more engaging is by adding images, perhaps one for each game we review. This post will guide you through adding static assets like images to your Next.js project.
Setting Up the Public Folder
First, we need a place to store our images. In a Next.js project, we can create a folder at the top level called public. Any files placed inside this folder will be treated as static assets and served directly by the Next.js server.
Creating the Public Folder
Create a public folder: At the root of your project, create a folder named public.
Add a test file: To understand how this works, create a file named test.txt inside the public folder and write some text, like "Hello world!".
You can now access this file in your browser at http://localhost:3000/test.txt. The Next.js server serves this file exactly as it is.
Adding Images
Let's add some images to our project. For this example, we will add images for the games we are reviewing.
Download the images: Download a package containing the images you want to use. For our example, let's assume you have a file named images.zip.
Unzip the file: Extract the contents of images.zip directly into the public folder. This will create an images folder inside public.
Verify the images: Ensure that the public/images folder contains the following files:
hollow-knight.jpg
stardew-valley.jpg
hellblade.jpg (Placeholder for a future review)
Displaying Images in Your Pages
Now that we have our images in place, let's display them on our review pages.
Adding an Image to the Hollow Knight Review
Open the hollow-knight review page in your project and add the following code:
// app/reviews/hollow-knight/page.jsx
import Heading from '@/components/Heading';
export default function HollowKnightPage() {
return (
<>
<Heading>Hollow Knight</Heading>
<img
src="/images/hollow-knight.jpg"
alt="Hollow Knight"
width="640"
height="360"
className="mb-2 rounded"
/>
<p>
This will be the review for Hollow Knight.
</p>
</>
);
}
In this example, we use the standard HTML <img> element to display the image. The src attribute points to the image file in the public/images folder, and we set the alt attribute for accessibility. The width and height attributes help the browser reserve the correct amount of space when rendering the page.
We also add some Tailwind CSS classes to style the image with rounded corners and a margin at the bottom.
Adding an Image to the Stardew Valley Review
Repeat the process for the stardew-valley review page:
// app/reviews/stardew-valley/page.jsx
import Heading from '@/components/Heading';
export default function StardewValleyPage() {
return (
<>
<Heading>Stardew Valley</Heading>
<img
src="/images/stardew-valley.jpg"
alt="Stardew Valley"
width="640"
height="360"
className="mb-2 rounded"
/>
<p>
This will be the review for Stardew Valley.
</p>
</>
);
}
Placeholder for Future Images
If you plan to add more reviews in the future, you can create placeholders. For example, for the hellblade review, you might add:
// app/reviews/hellblade/page.jsx (Placeholder)
import Heading from '@/components/Heading';
export default function HellbladePage() {
return (
<>
<Heading>Hellblade</Heading>
<img
src="/images/hellblade.jpg"
alt="Hellblade"
width="640"
height="360"
className="mb-2 rounded"
/>
<p>
This will be the review for Hellblade.
</p>
</>
);
}
Recap
Adding static assets like images to your Next.js project is straightforward:
Place your files in the public folder.
Access them directly using a path relative to the public folder (e.g., /images/your-image.jpg).
This approach ensures that your static files are served efficiently and can be accessed easily within your application. You can use this method for other types of static assets as well, such as videos, audio files, and documents.
By following these steps, you can make your website more visually appealing and engaging for your users. Happy coding!
Commentaires