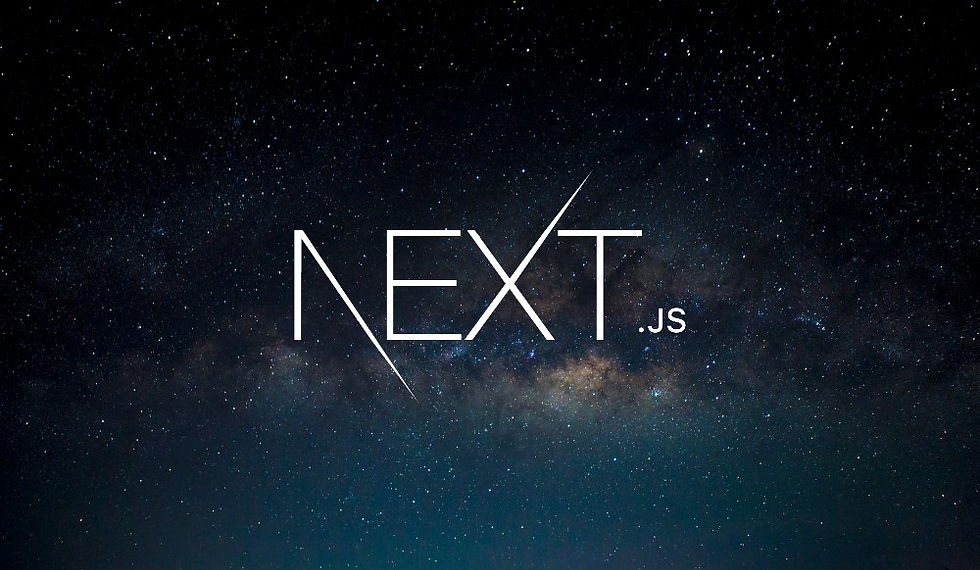
Getting started with Next.js can seem daunting, especially with the introduction of different routers and numerous features. This guide will walk you through setting up your first Next.js project, ensuring you understand every step along the way.
Initializing a New Project
The easiest way to initialize a new Next.js project is to use the create-next-app command, which you can run in a terminal with npx. This command will ask you a few questions, like the name of your project, if you want to use TypeScript rather than plain JavaScript, and a few other things. It then generates a project for you with everything already set up based on your choices.
While create-next-app is convenient, it can be overwhelming when you're learning Next.js for the first time because it generates many different files using various features. Therefore, we'll set up our first project manually, adding one feature at a time to ensure you fully understand all the various moving parts.
Setting Up the Project Manually
Step 1: Create a Project Folder
First, open your code editor. We'll use Cursor for this example. Start by creating a new folder for your project. You can name it something like next-reviews.
Step 2: Create a project using the following command
Automatic Installation:
npx create-next-app@latest
You will be prompted to provide the following information:
• What is your project named? my-app
• Would you like to use TypeScript? Yes / No
• Would you like to use ESLint? Yes / No
• Would you like to use Tailwind CSS? Yes / No
• Would you like your code inside a src/ directory? Yes / No
• Would you like to use App Router (recommended)? Yes / No
• Would you like to use Turbopack for next dev? Yes / No
• Would you like to customize the import alias (@/* by default)? Yes / No
• What import alias would you like configured? @/*
Manual Installation:
lternatively, you can manually create a project using the following command:
npm install next@latest react@latest react-dom@latest
Step 3: Initialize package.json
With the new folder open in Cursor, create a new file called package.json. This file will manage your project dependencies using npm. Here's a basic example of what package.json should contain:
{
"name": "next-reviews",
"private": true,
"dependencies": {
"next": "^14.0.0",
"react": "^18.2.0",
"react-dom": "^18.2.0"
}
}
Step 4: Install Dependencies
Open a new terminal in Cursor and run the following command to install the necessary dependencies:
npm install
This command will download Next.js, React, and ReactDOM, along with their transitive dependencies, and save them in the node_modules folder.
Step 5: Create the app Folder and Root Layout
Next, create a folder named app inside your project folder. This will be the directory for your pages and routes. Inside the app folder, create a file called layout.jsx. This file will contain the root layout, which serves as a template HTML document for all your pages.
Here’s what the layout.jsx file should look like:
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
{children}
</body>
</html>
);
}
This file exports a React component as the default export. The component returns JSX elements, starting with the html element, which contains the body. The children prop will insert the contents for each page.
Step 6: Running the Development Server
In the terminal, you can run the development server using the next command. This command is located inside the node_modules/.bin folder, and you can execute it using npx:
npx next dev
This command starts a local development server on port 3000. Open your web browser and go to http://localhost:3000. You should see a 404 page indicating that no pages have been created yet, but it confirms that the development server is working.
Creating Your First Page
We have our initial Next.js project set up and started the local development server with the next dev command. Now, if we try opening our website in the browser, at the moment we just get a "not found" message because we don't have any pages in our app yet.
Let's stop the server for the time being and see how to define a page.
Step 7: Define a Home Page
We can add a home page simply by creating a new file inside the app folder, called page.jsx. Just like the layout file, the page file must export a React component. Let's call it HomePage. Here’s a simple example:
export default function HomePage() {
return (
<h1>My first Next.js page</h1>
);
}
This will be the content of our home page. Save this file, and let's start the server again in the terminal.
Step 8: Add Scripts to package.json
Since we'll be using the next dev command all the time, it's better if we add a script to our package.json file. We can define a dev script that runs the next dev command. Here’s how you can do it:
{
"scripts": {
"dev": "next dev"
}
}
This way, in the terminal, we can type npm run dev instead, and this will execute next dev, starting the server on port 3000. By listing all our scripts in the package.json, it's also easier to see which commands are available in our project.
Step 9: View Your Home Page
Go back to the browser and reload the page. You should now see the heading "My first Next.js page," indicating that our home page is being displayed correctly.
Step 10: Using Developer Tools
While working on our pages, I recommend always using the Chrome Developer Tools, which you can open from the browser menu or with a keyboard shortcut. This way, you can see any log messages printed to the Console.
For most development, it’s convenient to keep the browser in a smaller window on the right side and the Cursor window on the left, so you can see both the code and the live updates in the browser.
Step 11: Live Updates
The development server will automatically update the page whenever we modify our code. For example, let's change the heading to "Indie Gamer," which will be the name of our website. As soon as we save this change, the page will update and show the new text.
export default function HomePage() {
return (
<>
<h1>Indie Gamer</h1>
<p>Only the best indie games, reviewed for you.</p>
</>
);
}
In JSX, we must have a single root element, so wrap everything in a React fragment (<> </>), which allows the page to render both the heading and the paragraph.
Step 12: Update the Root Layout
What’s special about Next.js and the App Router is that the content in the page will be inserted into the document defined by the RootLayout. Let's update the layout.jsx to include a header and footer.
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>[Header]</header>
<main>{children}</main>
<footer>[Footer]</footer>
</body>
</html>
);
}
By updating the layout, you can see that our page now has a header and a footer. The elements we return in the page component are rendered inside those from the layout component. In this sense, you can think of the layout as a template that will apply to all the pages.
Conclusion
By following these steps, you’ve set up your first Next.js project manually. This approach helps you understand the project structure and dependencies better than using an automated setup tool like create-next-app. You’ve learned how to:
Initialize a new project with package.json
Install necessary dependencies
Create a root layout component
Define a home page
Start a local development server
Use live updates and developer tools
In future steps, you can create individual pages and explore more advanced features of Next.js. Happy coding!
Comments