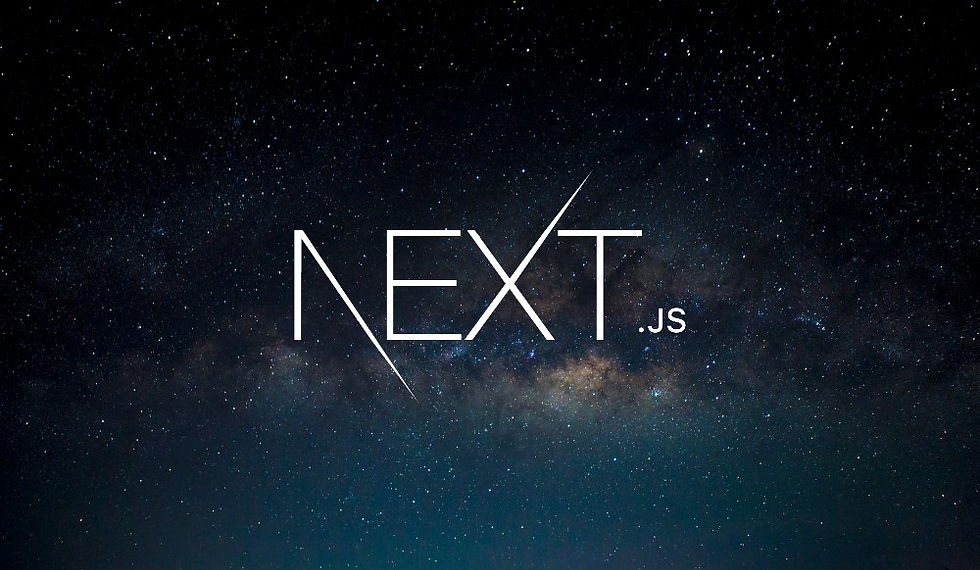
In our previous post, we added images to our individual game review pages. Now, let's take it a step further by enhancing the page that lists all the reviews. We'll create visually appealing cards for each review, complete with images and titles.
Setting Up Image Cards
We want each review to be represented by a card that includes an image and the game title. These cards will be clickable, allowing users to navigate to the detailed review pages.
Creating the Review Cards
First, let's open the file for the page listing all the reviews. We'll start by creating a card for each review.
Copy the Image Element: Let's copy the image element from one of our review pages since it will be very similar.
Adjust the Image Size: We'll make the image smaller (320 by 180 pixels) to fit multiple cards on the page.
Here's an example of how to structure a single card:
// app/reviews/index.jsx
import Link from 'next/link';
import Heading from '@/components/Heading';
export default function ReviewsPage() {
return (
<>
<Heading>Reviews</Heading>
<ul className="flex flex-col gap-3">
<li className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href="/reviews/hollow-knight">
<img src="/images/hollow-knight.jpg" alt="Hollow Knight"
width="320" height="180" className="rounded-t"
/>
<h2 className="py-1 text-center">
Hollow Knight
</h2>
</Link>
</li>
<li className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href="/reviews/stardew-valley">
<img src="/images/stardew-valley.jpg" alt="Stardew Valley"
width="320" height="180" className="rounded-t"
/>
<h2 className="py-1 text-center">
Stardew Valley
</h2>
</Link>
</li>
</ul>
</>
);
}
In this code, we create a list of cards, each containing an image and a title. The images are smaller to fit multiple cards on the page.
Styling the Cards
Next, we'll style each card to make them visually appealing.
Use Flexbox for Layout: We'll set the list element to use flexbox, displaying the items in a column with a gap between them.
Style Each Card: Each card will have a border, a white background, and rounded corners. We'll also add a shadow to give the impression that the card is slightly raised.
Hover Effect: To provide visual feedback when a user hovers over a card, we'll increase the shadow size.
Here's the updated code with the added styles:
// app/reviews/index.jsx
import Link from 'next/link';
import Heading from '@/components/Heading';
export default function ReviewsPage() {
return (
<>
<Heading>Reviews</Heading>
<ul className="flex flex-col gap-3">
<li className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href="/reviews/hollow-knight">
<img src="/images/hollow-knight.jpg" alt="Hollow Knight"
width="320" height="180" className="rounded-t"
/>
<h2 className="py-1 text-center">
Hollow Knight
</h2>
</Link>
</li>
<li className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href="/reviews/stardew-valley">
<img src="/images/stardew-valley.jpg" alt="Stardew Valley"
width="320" height="180" className="rounded-t"
/>
<h2 className="py-1 text-center">
Stardew Valley
</h2>
</Link>
</li>
</ul>
</>
);
}
Optimizing the Images
Currently, the images are rendered at 320x180 pixels, but the original images are much larger (1280x720 pixels). Ideally, we should resize each image to match the dimensions we use on the page to optimize loading times.
For now, we won't worry about this, as Next.js has built-in image optimization features that we'll cover later. These features will automatically optimize images for different screen sizes and resolutions, enhancing page load times and performance.
Recap
In this post, we enhanced our reviews page by creating visually appealing cards for each review. We used flexbox for layout, styled the cards with borders, rounded corners, and shadows, and added hover effects for better user interaction.
By following these steps, you can make your reviews page more engaging and user-friendly. Stay tuned for future posts where we'll delve into image optimization and other advanced features. Happy coding!
ความคิดเห็น