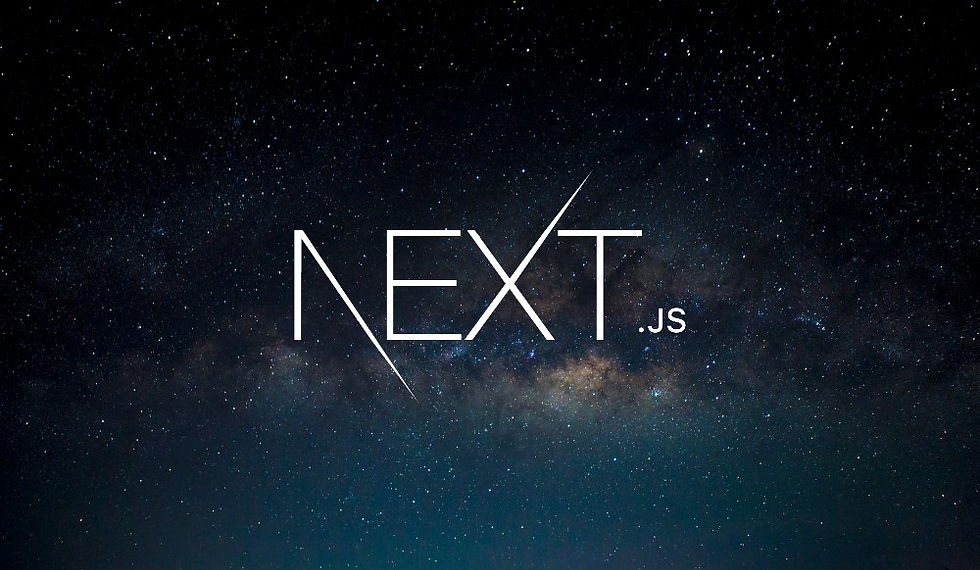
We've recently added images to our game review pages, making our website visually appealing. As we continue to enhance the user experience, it's time to focus on typography. Custom fonts can significantly impact the look and feel of your website, making it more engaging and polished.
In this blog post, we'll walk you through the process of adding and optimizing custom fonts in a Next.js application. We'll be using a futuristic font, which is perfect for our gaming website.
Why Font Optimization Matters
Before diving into the technical details, let's understand why font optimization is crucial:
Performance: Optimized fonts load faster, improving overall page load times.
Privacy: Hosting fonts locally ensures that no external requests are made, enhancing user privacy.
Customization: Custom fonts can align better with your website's theme and branding.
Adding a Custom Font
We'll start by adding a custom font to our Next.js application. For this example, we'll use a futuristic font that fits our gaming website's theme.
Step 1: Install the Font
First, we need to install the font. While you can link fonts directly from online repositories, it's better to host them locally for privacy and performance reasons. Next.js makes this process straightforward.
Step 2: Create a Font Configuration File
Inside your app folder, create a new file called fonts.js. This file will handle the font setup.
// app/fonts.js
import { Orbitron } from 'next/font/google';
export const orbitron = Orbitron({
subsets: ['latin'],
});
In this file, we import the Orbitron font from the next/font/google module. We then initialize the font with the required subset (in this case, latin).
Step 3: Apply the Font to Components
Next, we need to apply this custom font to our components. Let's start with the Heading component.
// components/Heading.jsx
import { orbitron } from '@/app/fonts';
export default function Heading({ children }) {
return (
<h1 className={`font-bold pb-3 text-2xl ${orbitron.className}`}>
{children}
</h1>
);
}
Here, we import the orbitron font and apply its className property to the h1 element using a backtick-delimited string. This ensures that our heading text uses the Orbitron font.
Step 4: Build and Test
Finally, build your application to see the custom font in action.
npm run build
npm start
Open your application in a browser and inspect the network requests using Chrome DevTools. You should see that the font file is served locally, not from an external server.
Inspecting the Font
To confirm that our custom font is applied correctly, let's inspect the heading element using the browser's Developer Tools.
Open Chrome DevTools: Right-click on the heading text and select "Inspect."
Check the Network Tab: Look for requests of type "font" to verify that the font file is loaded.
Inspect the Element: In the Elements panel, check the styles applied to the h1 tag. You should see a font-family rule using our custom Orbitron font.
Recap
In this blog post, we covered the steps to add and optimize a custom font in a Next.js application:
Installed the Font: We chose the Orbitron font and set it up in our project.
Created a Font Configuration File: We initialized the font with the required subsets.
Applied the Font to Components: We modified the Heading component to use the custom font.
Built and Tested the Application: We verified that the font is served locally and applied correctly.
By following these steps, you can easily enhance the typography of your Next.js application, improving both performance and user experience. Stay tuned for more tips and tricks on optimizing your web projects!
Comments