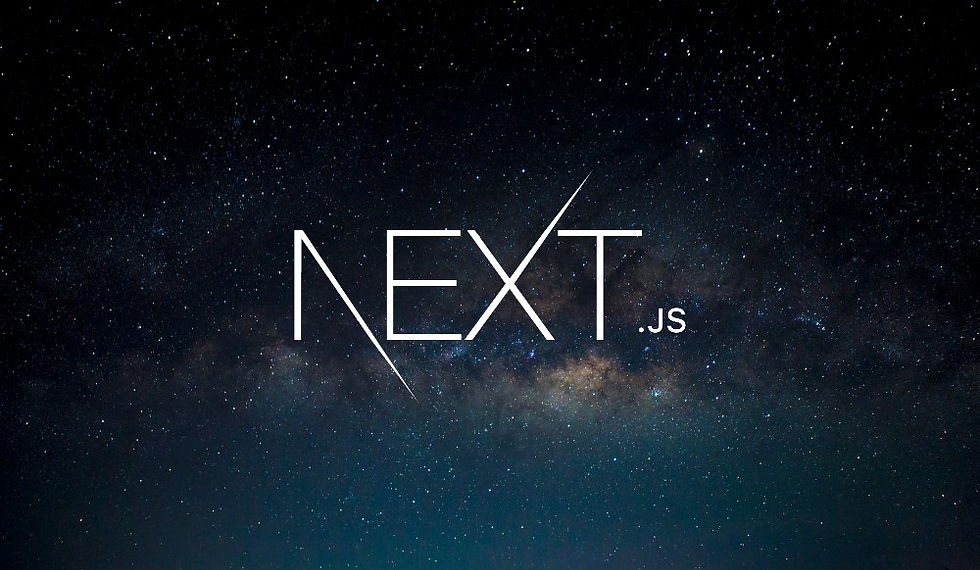
In our previous discussions, we explored how to add custom fonts to a Next.js application. We used the className approach to apply fonts to specific elements. However, since we are using Tailwind CSS for most of our styling, it makes sense to integrate our custom fonts directly into Tailwind's configuration. This allows us to use Tailwind's utility classes for a more seamless experience.
In this blog post, we will walk through the process of integrating a custom font into Tailwind CSS in a Next.js application. We'll use the Orbitron font as an example, but the steps are applicable to any font.
Why Integrate Custom Fonts with Tailwind CSS?
Consistency: Ensures that font styles are applied uniformly across the entire application.
Ease of Use: Allows you to use Tailwind utility classes for fonts, simplifying the process of applying custom fonts.
Scalability: Makes it easier to manage and update fonts as your project grows.
Step-by-Step Guide
Step 1: Initialize the Custom Font with a CSS Variable
First, we need to initialize our custom font and assign it to a CSS variable. This allows us to use the font globally across our application.
// app/fonts.js
import { Orbitron } from 'next/font/google';
export const orbitron = Orbitron({
subsets: ['latin'],
variable: '--font-orbitron',
});
Here, we initialize the Orbitron font and assign it to the CSS variable --font-orbitron.
Step 2: Define the CSS Variable Globally
Next, we need to define this CSS variable globally by adding it to the top-level html element.
// app/layout.jsx
import NavBar from '../components/NavBar';
import { orbitron } from './fonts';
import './globals.css';
export default function RootLayout({ children }) {
return (
<html lang="en" className={orbitron.variable}>
<body className="bg-orange-50 flex flex-col px-4 py-2 min-h-screen">
<header>
<NavBar />
</header>
{children}
</body>
</html>
);
}
We import the orbitron font and pass orbitron.variable as a CSS class to the html element, making the CSS variable globally available.
Step 3: Configure Tailwind to Use the Custom Font
We now need to configure Tailwind to recognize our custom font. This is done by extending the Tailwind configuration.
// tailwind.config.js
module.exports = {
content: [
'./app/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {
fontFamily: {
orbitron: ['var(--font-orbitron)', 'sans-serif'],
},
},
},
plugins: [],
};
In the tailwind.config.js file, we extend the theme and add a new fontFamily entry for orbitron. We use the CSS variable var(--font-orbitron) as the primary font and provide sans-serif as a fallback.
Step 4: Apply the Custom Font Using Tailwind Utility Classes
Now that our custom font is configured in Tailwind, we can use it like any other Tailwind utility class.
Example: Applying the Font to a Heading
// components/Heading.jsx
export default function Heading({ children }) {
return (
<h1 className="font-bold font-orbitron pb-3 text-2xl">
{children}
</h1>
);
}
We apply the font-orbitron class to the h1 element, making it use the Orbitron font.
Example: Applying the Font to the Navigation Bar
// components/NavBar.jsx
export default function NavBar() {
return (
<ul className="flex gap-2">
<li>
<Link href="/" className="font-bold font-orbitron text-orange-800 hover:underline">
Indie Gamer
</Link>
</li>
<li className="ml-auto">
<Link href="/reviews" className="text-orange-800 hover:underline">
Reviews
</Link>
</li>
<li>
<Link href="/about" className="text-orange-800 hover:underline">
About
</Link>
</li>
</ul>
);
}
We apply the font-orbitron class to the Link element, making the text use the Orbitron font.
Example: Applying the Font to Review Titles
// app/reviews/page.jsx
export default function ReviewsPage() {
return (
<div className="flex flex-col gap-4">
<Link href="/review/hollow-knight" className="block">
<img src="/images/hollow-knight.jpg" alt="" width="320" height="180" className="rounded-t" />
<h2 className="font-orbitron font-semibold py-1 text-center">
Hollow Knight
</h2>
</Link>
<Link href="/review/stardew-valley" className="block">
<img src="/images/stardew-valley.jpg" alt="" width="320" height="180" className="rounded-t" />
<h2 className="font-orbitron font-semibold py-1 text-center">
Stardew Valley
</h2>
</Link>
</div>
);
}
We apply the font-orbitron and font-semibold classes to the h2 elements, making the review titles use the Orbitron font.
Conclusion
Integrating custom fonts with Tailwind CSS in a Next.js application involves a few initial configuration steps, but it significantly simplifies the process of applying custom fonts throughout your project. By following the steps outlined in this blog post, you can easily configure and use custom fonts as Tailwind utility classes, making your development workflow more efficient and your application more consistent.
Happy coding!
Comments