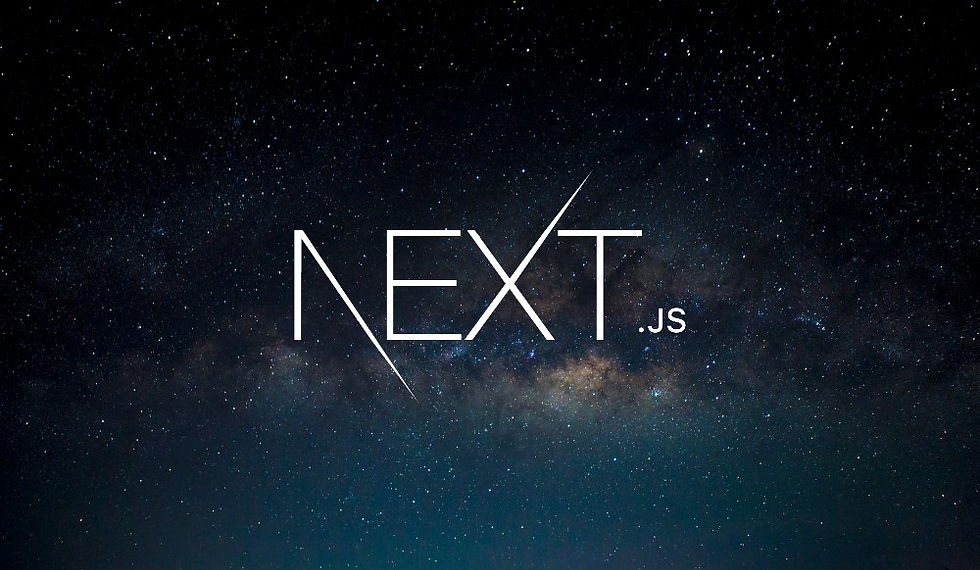
In our previous post, we covered how to add custom fonts to your Next.js application and configure them in Tailwind CSS. We used the font-orbitron class to apply the Orbitron font to various elements. But what if we want to set a custom font as the default for all text elements, like in the footer or the body of a review article?
In this blog post, we'll walk through the steps to override the default font in Tailwind CSS with a custom font. We'll use the Exo 2 font as an example, but you can choose any font you prefer.
Why Change the Default Font?
Consistency: Ensures a uniform look and feel across your application.
Readability: A more readable font can improve the user experience, especially for long texts.
Aesthetics: Custom fonts can give your website a unique and appealing look.
Step-by-Step Guide
Step 1: Initialize the Custom Font
First, we need to initialize our custom font and assign it to a CSS variable. This makes the font globally accessible in our application.
// app/fonts.js
import { Exo_2, Orbitron } from 'next/font/google';
export const exo2 = Exo_2({
subsets: ['latin'],
variable: '--font-exo2',
});
export const orbitron = Orbitron({
subsets: ['latin'],
variable: '--font-orbitron',
});
Here, we initialize the Exo 2 font and assign it to the CSS variable --font-exo2.
Step 2: Add the CSS Variable Globally
Next, we need to define this CSS variable globally by adding it to the top-level html element.
// app/layout.jsx
import NavBar from '../components/NavBar';
import { exo2, orbitron } from './fonts';
import './globals.css';
export default function RootLayout({ children }) {
return (
<html lang="en" className={`${exo2.variable} ${orbitron.variable}`}>
<body className="bg-orange-50 flex flex-col px-4 py-2 min-h-screen">
<header>
<NavBar />
</header>
{children}
</body>
</html>
);
}
We import the exo2 and orbitron fonts and pass their variables as CSS classes to the html element, making the CSS variables globally available.
Step 3: Configure Tailwind to Use the Custom Font as Default
We now need to configure Tailwind to recognize our custom font as the default font. This is done by extending the Tailwind configuration.
// tailwind.config.js
module.exports = {
content: [
'./app/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {
fontFamily: {
sans: ['var(--font-exo2)', 'sans-serif'],
orbitron: ['var(--font-orbitron)', 'sans-serif'],
},
},
},
plugins: [],
};
In the tailwind.config.js file, we extend the theme and redefine the sans font family to use the --font-exo2 CSS variable as the primary font. We also provide sans-serif as a fallback.
Step 4: Verify the Changes
With these changes, all text elements that use the default sans font family in Tailwind will now use the Exo 2 font. Let's verify this by looking at some text elements on the page.
Example: Footer Text
If you have a footer component, it will automatically use the new default font.
// components/Footer.jsx
export default function Footer() {
return (
<footer className="text-center py-4">
<p>© 2023 Indie Gamer. All rights reserved.</p>
</footer>
);
}
The text in the footer will now use the Exo 2 font because it inherits the default sans font family.
Example: Review Body Text
For a review article, the body text will also use the new default font.
// app/reviews/page.jsx
export default function ReviewsPage() {
return (
<div className="flex flex-col gap-4">
<Link href="/review/hollow-knight" className="block">
<img src="/images/hollow-knight.jpg" alt="" width="320" height="180" className="rounded-t" />
<h2 className="font-orbitron font-semibold py-1 text-center">
Hollow Knight
</h2>
<p className="text-justify">
The Hollow Knight is an incredible game that takes you on a journey through a beautifully crafted world...
</p>
</Link>
<Link href="/review/stardew-valley" className="block">
<img src="/images/stardew-valley.jpg" alt="" width="320" height="180" className="rounded-t" />
<h2 className="font-orbitron font-semibold py-1 text-center">
Stardew Valley
</h2>
<p className="text-justify">
Stardew Valley is a charming farming simulation game that allows you to build and manage your own farm...
</p>
</Link>
</div>
);
}
The paragraph text will use the new default font, Exo 2.
Conclusion
Changing the default font in Tailwind CSS is a straightforward process that can significantly enhance the look and feel of your Next.js application. By following the steps outlined in this blog post, you can easily configure and use a custom font as the default, making your application more unique and visually appealing.
If you encounter any issues with fonts or styles, remember that you can always use the Developer Tools in your browser to inspect elements and see which fonts and styles are being applied.
Happy coding!
Comments