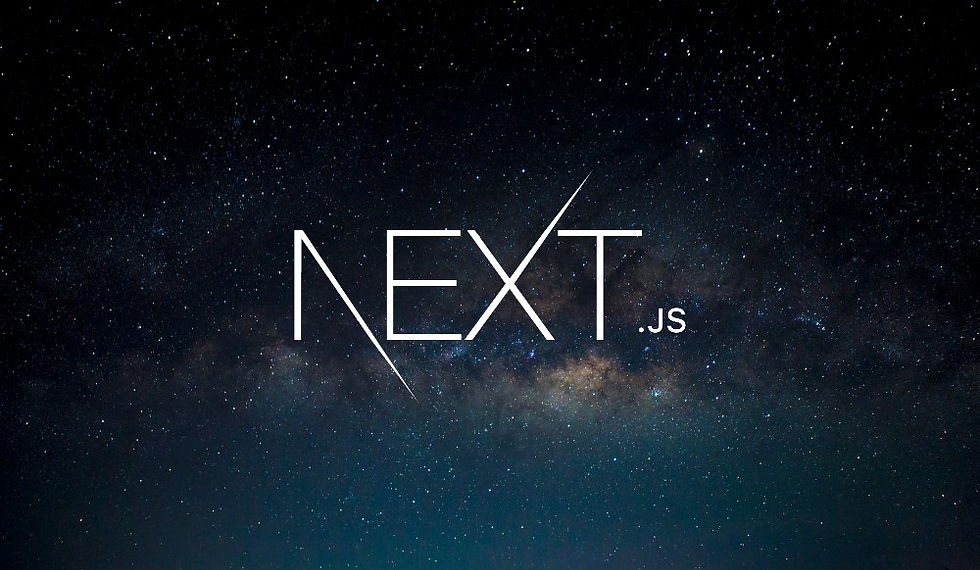
In a previous post, we discussed how to read content from Markdown files in a Next.js project. Now, we'll take it a step further and render this Markdown content as HTML on your web page. This process involves converting the raw Markdown text into HTML using a library. We'll walk through the steps to achieve this with a focus on making it easy to understand.
Installing the Markdown Library
To convert Markdown to HTML, we need a library. One popular choice is marked. Let's install it in our project.
Open your terminal.
Navigate to your project directory.
Run the following command to install marked:
npm install marked
You can run this command in a separate terminal to keep your development server running.
Updating the Component
Now that we have the marked library installed, we can update our React component to use it. Let's revisit our StardewValleyPage component and make the necessary changes.
Importing and Using marked
First, import the marked function from the marked library and use it to convert the Markdown text to HTML.
// app/StardewValleyPage.jsx
import { readFile } from 'node:fs/promises';
import { marked } from 'marked';
import Heading from '@/components/Heading';
export default async function StardewValleyPage() {
const text = await readFile('./content/reviews/stardew-valley.md', 'utf8');
const html = marked(text);
return (
<>
<Heading>Stardew Valley</Heading>
<img src="/images/stardew-valley.jpg" alt="Stardew Valley" width="640" height="360" className="mb-2 rounded" />
<article dangerouslySetInnerHTML={{ __html: html }} />
</>
);
}
Explanation of the Code
Importing marked: We import the marked function to convert Markdown to HTML.
Reading the File: We read the Markdown file using readFile, just like before.
Converting Markdown: We use marked(text) to convert the Markdown text to HTML.
Rendering HTML: We use the dangerouslySetInnerHTML prop to render the HTML content inside an <article> element.
Handling Deprecated Options
The marked library may show warnings about deprecated options like mangle and headerIds. To avoid these warnings, we can disable these options.
const html = marked(text, { mangle: false, headerIds: false });
By setting mangle and headerIds to false, we prevent these warnings from cluttering our logs.
Safely Rendering HTML
React makes it deliberately complex to render raw HTML to ensure you think carefully about security implications. Using dangerouslySetInnerHTML can expose your application to Cross-Site Scripting (XSS) attacks if you're rendering user-generated content. In our case, we're rendering Markdown files that we control, so the risk is minimal.
Styling the Rendered HTML
At this point, the Markdown content is rendered as HTML, but it may not look fully styled. For instance, headings and lists might not have proper formatting because Tailwind CSS resets most browser styles by default.
Adding Styles
To style the rendered HTML, you can extend Tailwind CSS with custom styles or use a plugin like @tailwindcss/typography.
First, install the typography plugin:
npm install @tailwindcss/typography
Then, add the plugin to your Tailwind CSS configuration:
// tailwind.config.js
module.exports = {
// ...
plugins: [
require('@tailwindcss/typography'),
],
};
Finally, apply the typography styles to your component:
<article className="prose" dangerouslySetInnerHTML={{ __html: html }} />
The prose class from the typography plugin will apply appropriate styles to your HTML content.
Conclusion
By following these steps, you can successfully render Markdown content as HTML in a Next.js project using the marked library. This approach allows you to keep your content organized and easy to manage while ensuring it is displayed correctly on your web pages.
Rendering Markdown as HTML enhances the readability and presentation of your content. With the help of Tailwind CSS and its plugins, you can also ensure your content looks visually appealing. Happy coding!
Comments