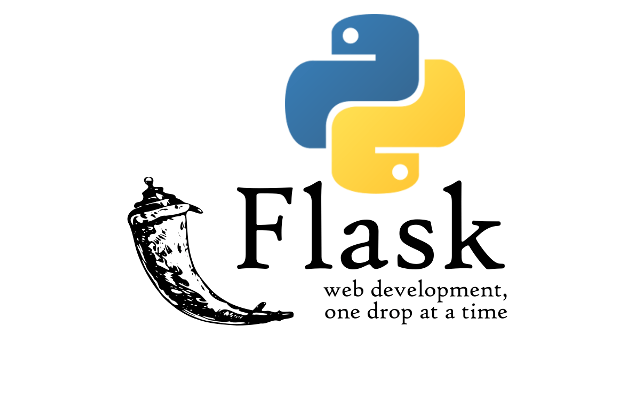
Hello everyone! Today, we're going to continue our journey with Flask, a web framework in Python, by learning how to integrate HTML and use different HTTP verbs like GET and POST. These are essential skills for building interactive web applications. Let's dive in!
What is Flask?
Flask is a tool that helps you create websites and web applications using Python. It's like a set of building blocks that you can use to put together your own web projects. Today, we'll focus on how to make your web pages more dynamic and interactive by integrating HTML and using HTTP methods.
Setting Up a Simple Flask App
Before we start, let's set up a basic Flask app. Here's how you can do it:
Step 1: Install Flask
First, make sure you have Flask installed. You can do this by running the following command in your terminal:
pip install flask
Step 2: Create a Flask App
Open your code editor and create a new file called app.py. Add the following code:
from flask import Flask, render_template, request, redirect, url_for
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Step 3: Run Your App
In your terminal, navigate to the folder where app.py is located and run:
python app.py
Step 4: Visit Your App
Open your web browser and go to http://127.0.0.1:5000/. You should see your HTML page displayed.
Integrating HTML with Flask
To make your Flask app display HTML pages, you need to create an HTML file and use Flask's render_template function.
Creating an HTML File
Create a Templates Folder: In the same directory as your app.py, create a folder named templates.
Create an HTML File: Inside the templates folder, create a file named index.html. Add the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome</title>
</head>
<body>
<h1>Welcome to My Flask App!</h1>
<form action="/submit" method="post">
<label for="science">Science:</label>
<input type="text" id="science" name="science" value="0"><br>
<label for="maths">Maths:</label>
<input type="text" id="maths" name="maths" value="0"><br>
<label for="c">C Programming:</label>
<input type="text" id="c" name="c" value="0"><br>
<label for="data_science">Data Science:</label>
<input type="text" id="data_science" name="data_science" value="0"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Rendering the HTML Page
In your app.py, use the render_template function to display the HTML page:
@app.route('/')
def home():
return render_template('index.html')
Using HTTP Verbs: GET and POST
HTTP verbs are methods used to communicate with web servers. The most common ones are GET and POST.
Handling Form Submission with POST
Let's create a route to handle form submissions using the POST method:
@app.route('/submit', methods=['POST'])
def submit():
science = float(request.form['science'])
maths = float(request.form['maths'])
c = float(request.form['c'])
data_science = float(request.form['data_science'])
total_score = (science + maths + c + data_science) / 4
if total_score >= 50:
result = "Pass"
else:
result = "Fail"
return render_template('result.html', result=result)
Creating a Result Page
Create another HTML file named result.html in the templates folder:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Result</title>
</head>
<body>
<h2>Final Results</h2>
<p>The result is: {{ result }}</p>
</body>
</html>
Conclusion
Congratulations! You've learned how to integrate HTML with Flask and use HTTP verbs to handle form submissions. This is a crucial step in building interactive web applications. As you continue to explore Flask, you'll discover more ways to enhance your web projects.
Stay tuned for more tutorials where we'll dive deeper into integrating CSS and JavaScript with Flask. Happy coding!
Comments