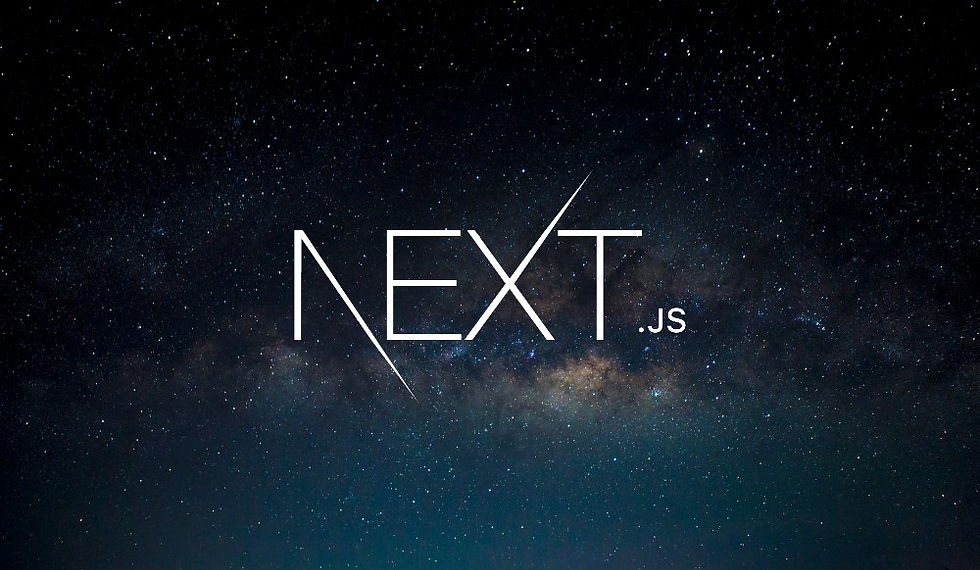
Dynamic routes have simplified our application by allowing us to use a single component to render multiple review pages based on the URL. This means that adding a new review is as simple as creating a new Markdown file. Let's explore how we can further enhance our application by automatically listing all available reviews without manually updating our code each time we add a new review.
Adding a New Review
To add a new review, we simply create a new Markdown file. For example, let's add a review for the game "Hellblade".
Create the Markdown File: Duplicate an existing review file and rename it to hellblade.md.
Update the Content: Change the front matter properties (title, date, image) and add the body content.
---
title: "Hellblade"
date: "2023-05-06"
image: "/images/hellblade.jpg"
---
This is the review for __Hellblade__.
With this new file in place, navigating to /reviews/hellblade will automatically display the Hellblade review content, thanks to our dynamic route setup.
Listing All Reviews Automatically
Currently, our ReviewsPage component lists only two reviews manually. We want to automate this process so that the list updates automatically whenever we add a new Markdown file. Here's how we can achieve this.
Step 1: Implement the getReviews Function
We'll start by implementing a getReviews function in our reviews.js file. This function will list all Markdown files in the content/reviews folder, extract their properties, and return them as an array.
import { readdir, readFile } from 'node:fs/promises';
import matter from 'gray-matter';
import { marked } from 'marked';
export async function getReview(slug) {
const text = await readFile(`./content/reviews/${slug}.md`, 'utf8');
const { content, data: { title, date, image } } = matter(text);
const body = marked(content);
return { slug, title, date, image, body };
}
export async function getReviews() {
const files = await readdir('./content/reviews');
const slugs = files.filter((file) => file.endsWith('.md'))
.map((file) => file.slice(0, -'.md'.length));
const reviews = [];
for (const slug of slugs) {
const review = await getReview(slug);
reviews.push(review);
}
return reviews;
}
Step 2: Update the ReviewsPage Component
Next, we'll update the ReviewsPage component to call the getReviews function and display the list of reviews.
import Link from 'next/link';
import Heading from '@/components/Heading';
import { getReviews } from '@/lib/reviews';
export default async function ReviewsPage() {
const reviews = await getReviews();
console.log('[ReviewsPage] reviews:', reviews);
return (
<>
<Heading>Reviews</Heading>
<ul>
{reviews.map((review) => (
<li key={review.slug}>
<Link href={`/reviews/${review.slug}`}>
<a>{review.title}</a>
</Link>
</li>
))}
</ul>
</>
);
}
Step 3: Test the Implementation
With these changes, our ReviewsPage component will automatically list all reviews found in the content/reviews folder. Each time we add a new Markdown file, it will be included in the list without any additional code changes.
Conclusion
By automating the process of listing reviews, we've made our application more dynamic and easier to maintain. Adding a new review is now as simple as creating a new Markdown file, and the ReviewsPage component will automatically update to include the new review. This approach leverages the power of dynamic routes and file system operations, making our application more robust and scalable.
Stay tuned for more improvements and happy coding!
Komentar