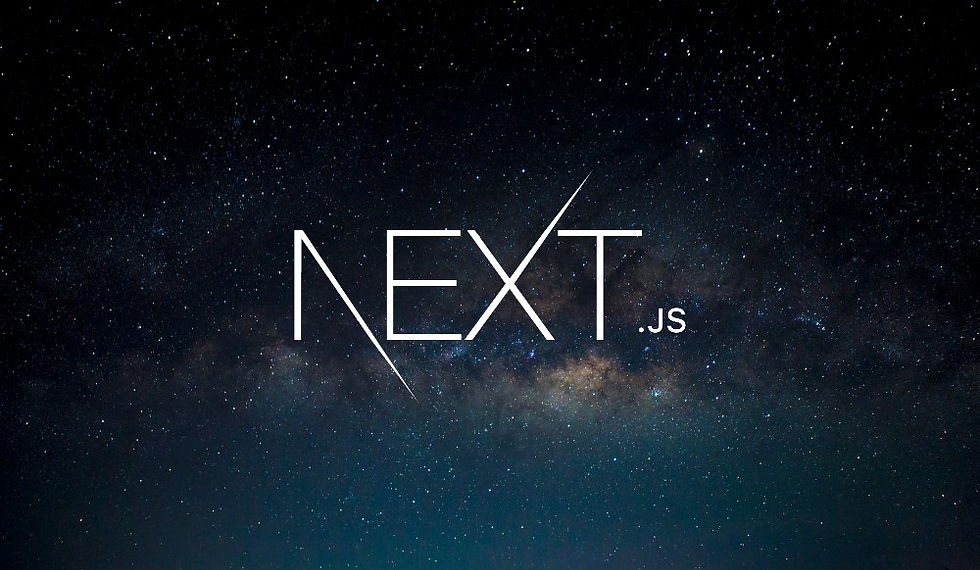
In our previous posts, we discussed how to fetch and manage review data using a getReviews function, which lists all the Markdown files and loads each review. In this post, we'll focus on rendering this data dynamically in our React component. This means we'll be displaying each review automatically, without manually coding each list item.
Fetching Reviews
First, let's ensure that our getReviews function is properly implemented. This function reads all Markdown files in the content/reviews folder, extracts their properties, and returns them as an array of review objects.
Here's a quick recap of our getReviews function:
import { readdir, readFile } from 'node:fs/promises';
import matter from 'gray-matter';
import { marked } from 'marked';
export async function getReview(slug) {
const text = await readFile(`./content/reviews/${slug}.md`, 'utf8');
const { content, data: { title, date, image } } = matter(text);
const body = marked(content);
return { slug, title, date, image, body };
}
export async function getReviews() {
const files = await readdir('./content/reviews');
const slugs = files.filter((file) => file.endsWith('.md'))
.map((file) => file.slice(0, -'.md'.length));
const reviews = [];
for (const slug of slugs) {
const review = await getReview(slug);
reviews.push(review);
}
return reviews;
}
Rendering the Reviews
Now, let's move on to rendering these reviews dynamically in our ReviewsPage component. Instead of hardcoding the list items, we'll loop through the reviews array and render each review as a list item.
Step 1: Fetch Reviews in the Component
First, we need to fetch the reviews data when the component is rendered. We'll do this by calling the getReviews function inside our ReviewsPage component.
import Link from 'next/link';
import Heading from '@/components/Heading';
import { getReviews } from '@/lib/reviews';
export default async function ReviewsPage() {
const reviews = await getReviews();
console.log('[ReviewsPage] reviews:', reviews);
return (
<>
<Heading>Reviews</Heading>
<ul className="flex flex-row flex-wrap gap-3">
{reviews.map((review) => (
<li key={review.slug}
className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href={`/reviews/${review.slug}`}>
<img src={review.image} alt=""
width="320" height="180" className="rounded-t"
/>
<h2 className="font-orbitron font-semibold py-1 text-center">
{review.title}
</h2>
</Link>
</li>
))}
</ul>
</>
);
}
Step 2: Dynamic Rendering with map
In the code above, we use the map method to iterate over the reviews array. For each review, we return a list item (<li>) containing the review's image and title. The key attribute is set to the review's slug, ensuring each list item has a unique identifier, which is important for React's rendering performance.
Step 3: Styling and Layout
To make our reviews look good on different screen sizes, we use some CSS classes:
flex flex-row flex-wrap: Displays the items in a row and wraps them if there's not enough space.
gap-3: Adds a gap between the items.
bg-white border rounded shadow w-80 hover:shadow-xl: Adds styling to each review card for a clean look and a hover effect.
Here's the styled list:
<ul className="flex flex-row flex-wrap gap-3">
{reviews.map((review) => (
<li key={review.slug}
className="bg-white border rounded shadow w-80 hover:shadow-xl">
<Link href={`/reviews/${review.slug}`}>
<img src={review.image} alt=""
width="320" height="180" className="rounded-t"
/>
<h2 className="font-orbitron font-semibold py-1 text-center">
{review.title}
</h2>
</Link>
</li>
))}
</ul>
Step 4: Test the Implementation
After saving the changes, our ReviewsPage will automatically list all available reviews. Each time a new Markdown file is added to the content/reviews folder, it will be included in the list without any additional code changes.
Conclusion
By dynamically rendering review data, we've made our application more flexible and easier to maintain. The map method allows us to loop through the reviews and render them efficiently, while CSS classes help ensure that the layout looks good on any screen size. This approach not only saves time but also enhances the user experience by automatically updating the reviews list.
Stay tuned for more tips and tricks on building dynamic web applications! Happy coding!
Comments