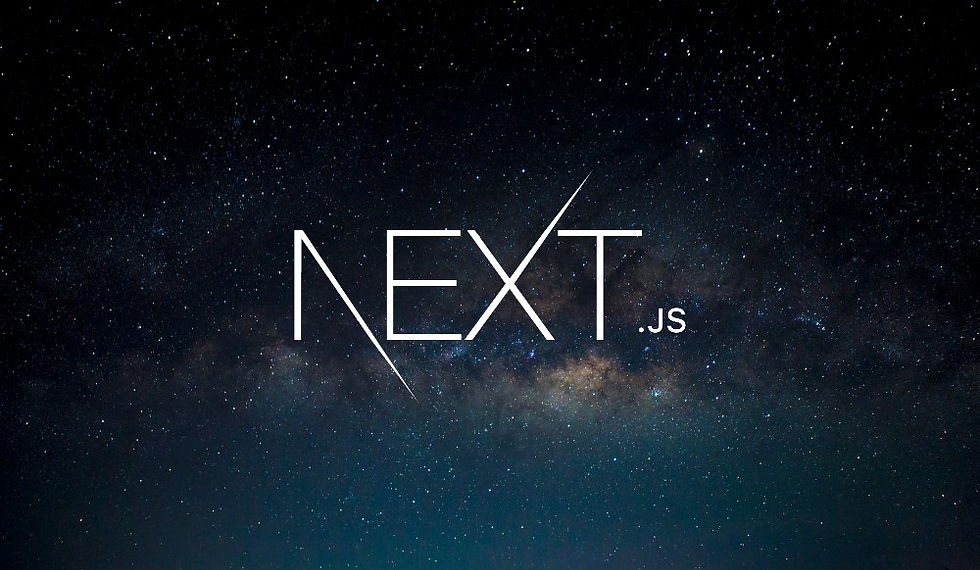
In our previous post, we discussed how to dynamically render reviews on the ReviewsPage component. Now, let's dive into how we can statically generate pages for dynamic routes in a Next.js application. This ensures that our pages are pre-rendered at build time, leading to faster load times and better performance.
Understanding Dynamic Routes
Dynamic routes in Next.js allow us to create pages that match patterns and handle variable segments in the URL. For example, a path like /reviews/[slug] can match multiple URLs such as /reviews/hellblade or /reviews/hollow-knight.
The Need for Static Generation
While dynamic routes are powerful, they typically rely on server-side rendering (SSR) at runtime. This means that every time a user requests a page, the server must generate the HTML on-the-fly. While this is flexible, it can be slower compared to serving pre-generated static pages.
Benefits of Static Generation:
Performance: Pre-generated HTML files are served instantly without the need for server-side processing.
Scalability: Static files can be served by a CDN, reducing the load on your server.
Reliability: With fewer moving parts at runtime, static sites tend to be more reliable.
Implementing Static Generation for Dynamic Routes
To statically generate pages even for dynamic routes, we need to inform Next.js about all the possible paths during the build process. This can be achieved using the generateStaticParams function.
Step-by-Step Implementation:
Create a Function to Get Slugs
First, we need a function to get all the slugs (i.e., the unique identifiers for each review). This will help us determine which pages to statically generate.
import { readdir } from 'node:fs/promises';
export async function getSlugs() {
const files = await readdir('./content/reviews');
return files.filter((file) => file.endsWith('.md'))
.map((file) => file.slice(0, -'.md'.length));
}
2. Define generateStaticParams Function
Next, we define the generateStaticParams function to return an array of paths that need to be pre-rendered. Each path is represented by an object containing the slug parameter.
export async function generateStaticParams() {
const slugs = await getSlugs();
return slugs.map((slug) => ({ slug }));
}
3. Modify the ReviewPage Component
We need to update the ReviewPage component to use the generateStaticParams function. This ensures that the component receives the correct slug parameter.
import Heading from '@/components/Heading';
import { getReview } from '@/lib/reviews';
export default async function ReviewPage({ params: { slug } }) {
const review = await getReview(slug);
console.log('[ReviewPage] rendering', slug);
return (
<>
<Heading>{review.title}</Heading>
<div dangerouslySetInnerHTML={{ __html: review.body }} />
</>
);
}
4. Build and Test
After implementing the above changes, we need to build our application to see the static generation in action.
npm run build
npm start
During the build process, Next.js will use the generateStaticParams function to determine which pages to pre-render. If successful, you'll see messages indicating that specific paths under /reviews/[slug] have been statically generated.
Conclusion
By implementing static generation for dynamic routes, we combine the flexibility of dynamic routing with the performance benefits of static sites. This approach ensures that users get fast and reliable access to our pages, while still allowing us to add new content dynamically.
With the steps outlined above, you can now pre-generate pages for any dynamic route in your Next.js application, making your site faster and more efficient. Happy coding!
Comentarios