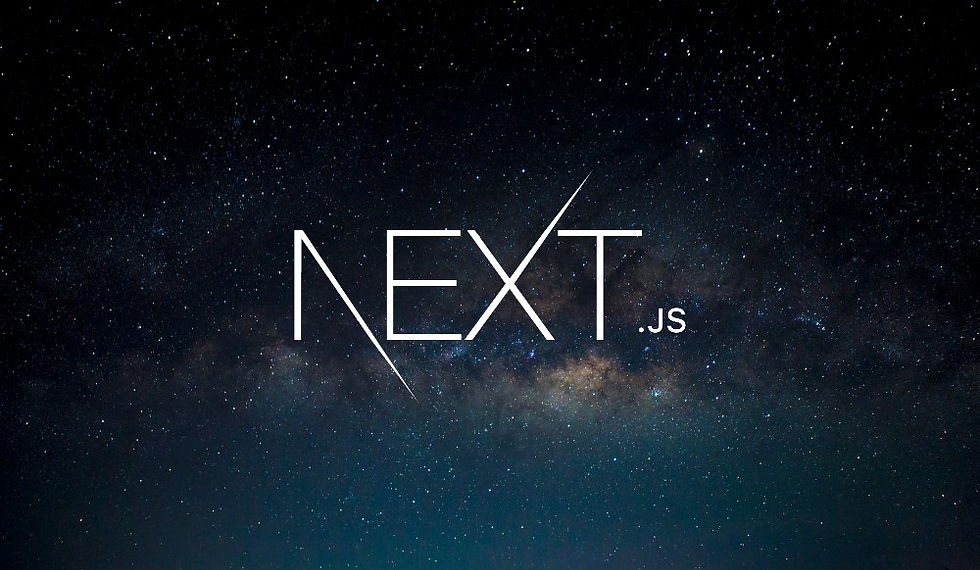
In our previous discussions, we covered how to dynamically load reviews from Markdown files and display them on the Reviews page. However, our Home page still shows hard-coded review data. Today, we'll walk through an exercise to change this by automatically displaying the latest review as the featured review on the Home page.
Objective
The goal is to modify the Home page so that it automatically loads and displays the most recent review as the featured review. This ensures that our Home page content stays up-to-date without manual intervention.
Steps to Achieve This
Create a Function to Get the Featured Review
Sort Reviews by Date
Update the Home Page to Use the Featured Review
1. Create a Function to Get the Featured Review
First, we'll add a new function in our reviews.js file called getFeaturedReview. This function will return the most recent review.
import { readdir, readFile } from 'node:fs/promises';
import matter from 'gray-matter';
import { marked } from 'marked';
export async function getFeaturedReview() {
const reviews = await getReviews();
return reviews[0];
}
2. Sort Reviews by Date
Next, we need to ensure that our reviews are sorted by date, with the most recent review first. We'll modify the getReviews function to sort the reviews accordingly.
export async function getReviews() {
const files = await readdir('./content/reviews');
const slugs = files.filter((file) => file.endsWith('.md'))
.map((file) => file.slice(0, -'.md'.length));
const reviews = [];
for (const slug of slugs) {
const review = await getReview(slug);
reviews.push(review);
}
reviews.sort((a, b) => b.date.localeCompare(a.date)); // Sort by date, most recent first
return reviews;
}
3. Update the Home Page to Use the Featured Review
Finally, we'll update the Home page to use the getFeaturedReview function. This function will fetch the latest review, which we can then display on the Home page.
import Link from 'next/link';
import Heading from '@/components/Heading';
import { getFeaturedReview } from '@/lib/reviews';
export default async function HomePage() {
const review = await getFeaturedReview();
console.log('[HomePage] rendering');
return (
<>
<Heading>Featured Review</Heading>
<p className="text-center mb-4">
Check out our latest review!
</p>
<div className="bg-white border rounded shadow w-80 hover:shadow-xl sm:w-full">
<Link href={`/reviews/${review.slug}`} className="flex flex-col sm:flex-row">
<img src={review.image} alt="" width="320" height="180" className="rounded-t sm:rounded-l sm:rounded-r-none" />
<h2 className="font-orbitron font-semibold py-1 text-center sm:px-2">
{review.title}
</h2>
</Link>
</div>
</>
);
}
Explanation
getFeaturedReview Function: This function calls getReviews to get all reviews and returns the first one, which is the most recent due to the sorting.
Sorting Reviews: In getReviews, we use the sort method to sort the reviews by date in descending order. This ensures the most recent review is at the top.
HomePage Component: The Home page component calls getFeaturedReview to fetch the latest review and displays its title and image dynamically.
Benefits
Automatic Updates: The Home page will always display the latest review without manual updates.
Consistency: Ensures the Home page and Reviews page are consistent in showing the most recent reviews first.
Scalability: As new reviews are added, the Home page will automatically update to feature the most recent one.
By following these steps, we've enhanced our Home page to dynamically feature the latest review. This exercise demonstrates the power of combining dynamic data fetching with static site generation, ensuring our site is both performant and up-to-date. Happy coding!
Comments