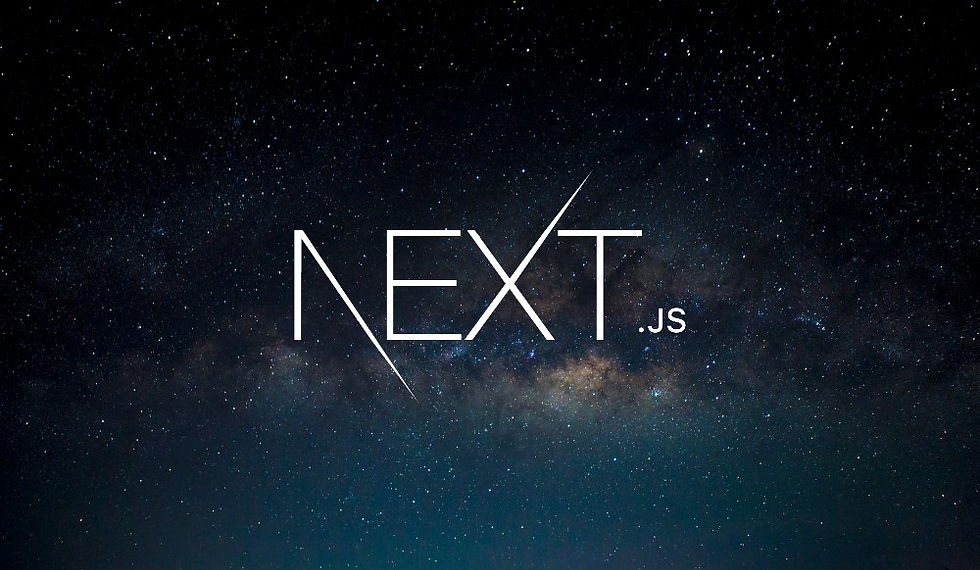
Creating Additional Pages in Next.js
We’ve set up our initial home page for our Next.js website. Now, let's explore how to create additional pages.
Understanding the URL Path
The home page is displayed at the root of our website, typically accessible via http://localhost:3000/. The URL structure is crucial for organizing your pages.
Step 1: Create a New Page
Suppose we want a page to list all the reviews. Inside the app folder, create a new folder called reviews. Inside this folder, create a file named page.jsx. Here’s a simple example of what the page.jsx file might contain:
export default function ReviewsPage() {
return (
<>
<h1>Reviews</h1>
<p>Here we'll list all the reviews.</p>
</>
);
}
Step 2: Nested Pages
If you want to create a page for an individual review, like for the game "Stardew Valley," create another folder inside reviews called stardew-valley and add a page.jsx file in there. Here’s an example:
export default function StardewValleyPage() {
return (
<>
<h1>Stardew Valley</h1>
<p>[Placeholder for review content]</p>
</>
);
}
Step 3: URL Mapping
Next.js uses file-based routing, meaning the folder structure inside the app directory maps directly to URL paths. For example:
app/page.jsx corresponds to /
app/reviews/page.jsx corresponds to /reviews
app/reviews/stardew-valley/page.jsx corresponds to /reviews/stardew-valley
Step 4: Dynamic Routing
For more dynamic routing, you might want to use dynamic segments. This can be done by creating a folder with the name in square brackets. For example, [id] inside the reviews folder would create a dynamic route like /reviews/[id].
Conclusion
Next.js makes it straightforward to manage your application's routing using a file-based approach. By organizing your pages within the app folder, you can easily map URLs to React components without additional configuration. This method not only simplifies routing but also enhances the clarity and maintainability of your project structure.
By following these steps, you can expand your Next.js application with additional pages and nested routes, providing a robust structure for your growing project.
Exercise: Creating the About Page and Hollow Knight Review Page
We now have three pages in our web application: the HomePage, the ReviewsPage (accessible at the "/reviews" path), and the review for Stardew Valley (accessible at "/reviews/stardew-valley"). These clean URLs not only improve user experience but also help search engines index the pages better for relevant keywords.
As an exercise, let's add a couple more pages to our application: an "/about" page and a review page for another game, "Hollow Knight."
Step 1: Create the About Page
Create a Folder for the About Page: Inside the app folder, create a new folder named about.
Add the Page File: Inside the about folder, create a file named page.jsx.
Define the Component: Add the following code to page.jsx:
export default function AboutPage() {
return (
<>
<h1>About</h1>
<p>
A website created to learn Next.js
</p>
</>
);
}
Step 2: Create the Hollow Knight Review Page
Create a Folder for the Hollow Knight Review: Inside the app/reviews folder, create a new folder named hollow-knight.
Add the Page File: Inside the hollow-knight folder, create a file named page.jsx.
Define the Component: Add the following code to page.jsx:
export default function HollowKnightPage() {
return (
<>
<h1>Hollow Knight</h1>
<p>
This will be the review for Hollow Knight.
</p>
</>
);
}
Verify the New Pages
If you completed the exercise, you should now have the following structure in your app folder:
app/about/page.jsx
app/reviews/hollow-knight/page.jsx
To view these pages, start your development server if it’s not already running:
npm run dev
Then, open your browser and navigate to:
http://localhost:3000/about to see the About page.
http://localhost:3000/reviews/hollow-knight to see the Hollow Knight review page.
Adding Pages with TypeScript
If you prefer using TypeScript, the steps are almost identical, but the file extensions and some minor syntax changes will be needed.
Step 1: Create the About Page with TypeScript
Create a Folder for the About Page: Inside the app folder, create a new folder named about.
Add the Page File: Inside the about folder, create a file named page.tsx.
Define the Component: Add the following code to page.tsx:
export default function AboutPage() {
return (
<>
<h1>About</h1>
<p>
A website created to learn Next.js
</p>
</>
);
}
Step 2: Create the Hollow Knight Review Page with TypeScript
Create a Folder for the Hollow Knight Review: Inside the app/reviews folder, create a new folder named hollow-knight.
Add the Page File: Inside the hollow-knight folder, create a file named page.tsx.
Define the Component: Add the following code to page.tsx:
export default function HollowKnightPage() {
return (
<>
<h1>Hollow Knight</h1>
<p>
This will be the review for Hollow Knight.
</p>
</>
);
}
Verify the New TypeScript Pages
To view these TypeScript pages, start your development server if it’s not already running:
npm run dev
Then, open your browser and navigate to:
http://localhost:3000/about to see the About page.
http://localhost:3000/reviews/hollow-knight to see the Hollow Knight review page.
Understanding the File-Based Routing
Next.js uses a file-based routing system, where the folder structure inside the app directory maps directly to URL paths. Each folder represents a route segment, and each page.jsx or page.tsx file inside these folders maps to a specific URL path.
URL Path Mapping
app/page.jsx maps to /
app/reviews/page.jsx maps to /reviews
app/reviews/stardew-valley/page.jsx maps to /reviews/stardew-valley
app/about/page.jsx or app/about/page.tsx maps to /about
app/reviews/hollow-knight/page.jsx or app/reviews/hollow-knight/page.tsx maps to /reviews/hollow-knight
This structure helps you organize your application’s routes in a clear and intuitive manner.
Advantages of File-Based Routing
While it might seem a bit redundant to have many page.jsx or page.tsx files, this approach has several advantages:
Clarity: The folder structure clearly indicates the URL paths.
Organization: You can easily find and manage your pages.
Scalability: Adding new pages or nested routes is straightforward.
Navigating the Project
If you have many page.jsx or page.tsx files, finding the right one might seem challenging at first. However, most code editors, like Cursor, provide powerful search features. You can use shortcuts to quickly find files:
On macOS: Command + P
On Windows/Linux: Control + P
By typing part of the path or filename, you can quickly locate the specific page.jsx or page.tsx file you need.
Conclusion
By following these steps, you’ve learned how to add new pages to your Next.js application and understand the file-based routing system. This exercise reinforces how simple and powerful Next.js routing can be, making it easy to manage and scale your application.
In future posts, we’ll explore more advanced features, such as nested layouts and dynamic routing. For now, continue experimenting with adding new pages and get comfortable with the structure. Happy coding!
Comments