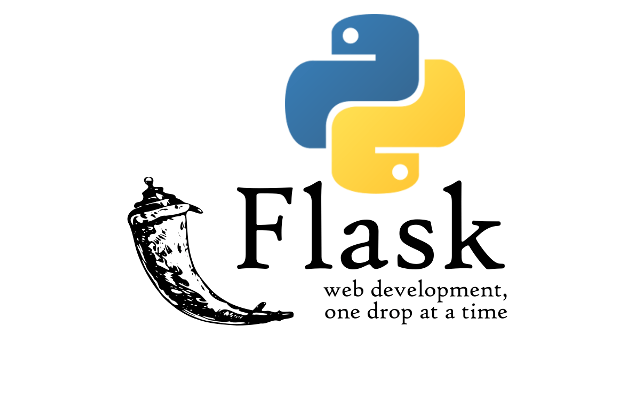
Hello everyone! Today, we're going to explore the Jinja2 template engine, a powerful tool used in Flask to create dynamic web pages. This is an exciting topic because it allows you to integrate HTML with data, making your web applications more interactive and responsive. Let's dive in!
What is Jinja2?
Jinja2 is a template engine for Python, used by Flask to render HTML pages. It allows you to combine HTML with data from your application, so you can display dynamic content on your web pages. This means you can create web pages that change based on the data you provide.
Setting Up a Simple Flask App
Before we start, let's set up a basic Flask app. Here's how you can do it:
Step 1: Install Flask
First, make sure you have Flask installed. You can do this by running the following command in your terminal:
pip install flask
Step 2: Create a Flask App
Open your code editor and create a new file called app.py. Add the following code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Step 3: Run Your App
In your terminal, navigate to the folder where app.py is located and run:
python app.py
Step 4: Visit Your App
Open your web browser and go to http://127.0.0.1:5000/. You should see your HTML page displayed.
Using Jinja2 in HTML
Let's create an HTML file and use Jinja2 to display dynamic content.
Creating an HTML File
Create a Templates Folder: In the same directory as your app.py, create a folder named templates.
Create an HTML File: Inside the templates folder, create a file named index.html. Add the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome</title>
</head>
<body>
<h1>Welcome to My Flask App!</h1>
<p>The result is: {{ result }}</p>
</body>
</html>
Rendering Dynamic Content
In your app.py, pass data to the HTML page using Jinja2:
@app.route('/')
def home():
result = "Pass"
return render_template('index.html', result=result)
Explanation: The {{ result }} in the HTML file is a Jinja2 expression. It will be replaced by the value of the result variable from the Flask app.
Advanced Jinja2 Techniques
Jinja2 allows you to use more advanced techniques like loops and conditionals in your HTML.
Using Conditionals
You can use if-else statements to display different content based on conditions:
{% if result == "Pass" %}
<p>Congratulations! You passed.</p>
{% else %}
<p>Sorry, you failed. Try again!</p>
{% endif %}
Using Loops
You can also use loops to iterate over data:
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
Explanation: This loop will create a list item for each element in the items list.
Adding Comments
You can add comments in Jinja2 that won't appear in the final HTML:
{# This is a comment #}
Conclusion
Congratulations! You've learned how to use the Jinja2 template engine in Flask to create dynamic web pages. This is a crucial step in building interactive web applications. As you continue to explore Flask, you'll discover more ways to enhance your web projects.
Stay tuned for more tutorials where we'll dive deeper into integrating CSS and JavaScript with Flask. Happy coding!
This version is structured, uses consistent formatting for code blocks, and provides clear explanations, making it easier to follow and understand.
Comments