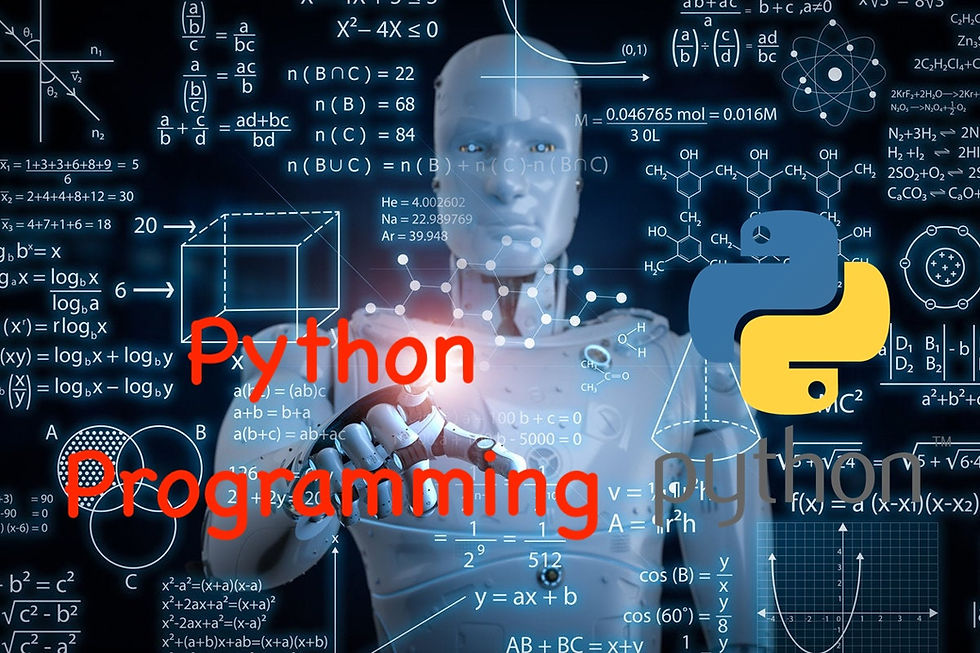
Hey everyone! Today, we're diving into some powerful data structures in Python: sets, dictionaries, and tuples. These are essential tools in Python, especially when you're doing data analysis. Let's get started!
Sets
What is a Set?
A set is an unordered collection of unique elements. This means it doesn't allow duplicates and the order of elements is not fixed.
Here’s how you can create a set:
# Creating an empty set
my_set = set()
print(my_set) # Output: set()
print(type(my_set)) # Output: <class 'set'>
# Creating set with elements
my_set = {1, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
# Duplicates are automatically removed
my_set = {1, 2, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
Adding Elements to a Set
You can add elements to a set using the add() method:
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
Set Operations
Sets support mathematical operations like union, intersection, and difference:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
# Union
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5}
# Intersection
print(set1.intersection(set2)) # Output: {3}
# Difference
print(set1.difference(set2)) # Output: {1, 2}
Dictionaries
What is a Dictionary?
A dictionary is a collection of key-value pairs. Each key is unique and maps to a value.
Here’s how you can create a dictionary:
# Creating an empty dictionary
my_dict = {}
print(my_dict) # Output: {}
print(type(my_dict)) # Output: <class 'dict'>
# Creating a dictionary with key-value pairs
my_dict = {
"name": "Revanth",
"age": 12,
"grade": "7th"
}
print(my_dict)
Accessing Dictionary Items
You can access dictionary items using their keys:
print(my_dict["name"]) # Output: Revanth
print(my_dict["age"]) # Output: 12
Adding and Removing Items
You can add new key-value pairs or update existing ones, and remove pairs using del:
# Adding or updating
my_dict["school"] = "XYZ High School"
print(my_dict)
# Removing
del my_dict["age"]
print(my_dict)
Iterating Through Dictionaries
You can loop through dictionaries to get keys, values, or both:
# Loop through keys
for key in my_dict:
print(key)
# Loop through values
for value in my_dict.values():
print(value)
# Loop through key-value pairs
for key, value in my_dict.items():
print(f"{key}: {value}")
Nested Dictionaries
Dictionaries can contain other dictionaries, allowing for more complex data structures:
car_types = {
"car1": {"model": "Audi", "year": 2010},
"car2": {"model": "BMW", "year": 2015},
"car3": {"model": "Mercedes", "year": 2020}
}
# Accessing nested dictionary items
print(car_types["car1"]["model"]) # Output: Audi
print(car_types["car3"]["year"]) # Output: 2020
Tuples
What is a Tuple?
A tuple is a collection of ordered, immutable items. Once a tuple is created, you cannot change its elements.
Here’s how you can create a tuple:
# Creating an empty tuple
my_tuple = ()
print(my_tuple) # Output: ()
# Creating a tuple with elements
my_tuple = ("apple", "banana", "cherry")
print(my_tuple) # Output: ('apple', 'banana', 'cherry')
Accessing Tuple Items
You can access items in a tuple using their index:
print(my_tuple[0]) # Output: apple
print(my_tuple[1]) # Output: banana
print(my_tuple[-1]) # Output: cherry
Immutability of Tuples
Tuples cannot be changed once created. If you try to change an element, Python will raise an error:
# This will raise an error
my_tuple[0] = "orange" # TypeError: 'tuple' object does not support item assignment
Useful Tuple Methods
Tuples have only a few methods because they are immutable. Some useful ones are count() and index():
my_tuple = ("apple", "banana", "cherry", "apple")
# Count occurrences of an item
print(my_tuple.count("apple")) # Output: 2
# Find the index of an item
print(my_tuple.index("banana")) # Output: 1
Conclusion
Understanding sets, dictionaries, and tuples in Python is crucial for efficient data handling and manipulation. These data structures help you organize your data and perform various operations with ease.
Keep practicing, and don't hesitate to explore more about these data structures. Happy coding!
Thank you for reading! If you found this post helpful, share it with your friends and family. Happy learning!
Comments