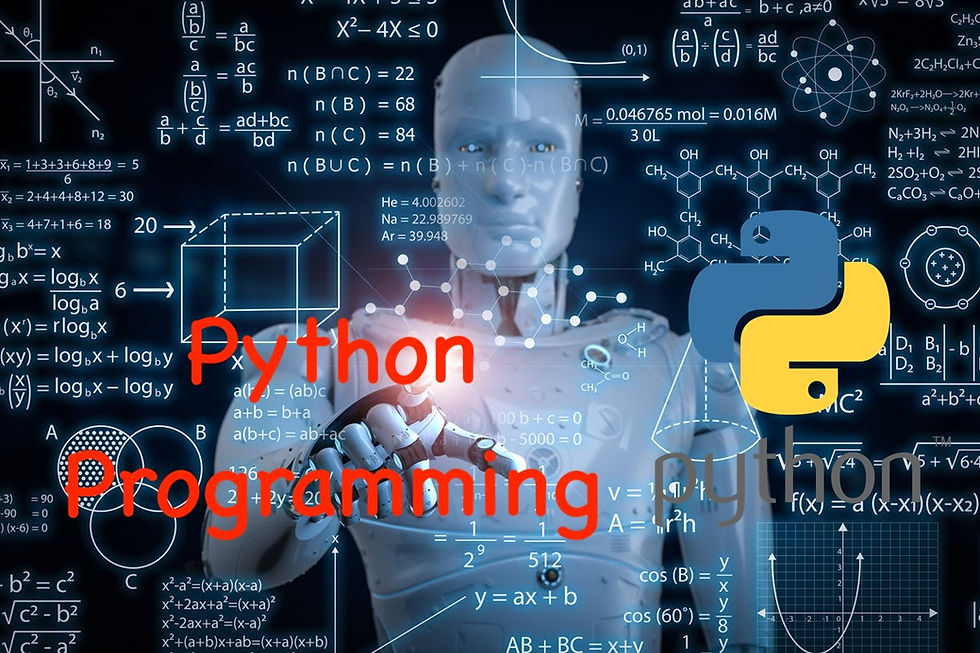
Hello everyone! Today, we're diving into one of the most important libraries in Python for data analysis: Numpy. Numpy is a powerful tool that helps us handle multi-dimensional arrays and perform a variety of mathematical operations efficiently. Let's get started!
What is Numpy?
Numpy is a general-purpose array-processing package. It provides high-performance multi-dimensional array objects and tools for working with these arrays. It's especially useful in machine learning and data analysis where dealing with arrays is a common task.
Why Use Numpy?
Numpy is fast because it has bindings of C++ libraries, which means operations are executed quickly. Whether you're multiplying arrays or performing complex mathematical calculations, Numpy makes everything faster and easier.
Getting Started with Numpy
Installing Numpy
Before using Numpy, you need to install it. If you've installed Python manually or are using a different Python environment, you can install Numpy using pip:
pip install numpy
Or if you're using a conda environment:
conda install numpy
Importing Numpy
To use Numpy in your Python code, you need to import it. The common practice is to import Numpy using the alias np:
import numpy as np
Working with Arrays
What is an Array?
An array is a data structure that stores values of the same data type. Unlike lists, arrays in Numpy require all elements to be of the same type.
Creating Arrays
You can create arrays in different ways. Let's start with a one-dimensional array:
# Import Numpy
import numpy as np
# Creating a list
my_list = [1, 2, 3, 4, 5]
# Converting list to Numpy array
my_array = np.array(my_list)
print(my_array) # Output: [1 2 3 4 5]
print(type(my_array)) # Output: <class 'numpy.ndarray'>
Understanding Array Dimensions
Arrays can have multiple dimensions. Here’s how you can create a two-dimensional array:
# Creating a 2D array
my_2d_array = np.array([
[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10],
[11, 12, 13, 14, 15]
])
print(my_2d_array)
Checking the Shape of an Array
The shape of an array tells you its dimensions:
print(my_2d_array.shape) # Output: (3, 5)
This means the array has 3 rows and 5 columns.
Reshaping Arrays
You can reshape arrays to different dimensions as long as the total number of elements remains the same:
# Reshaping the array to 5 rows and 3 columns
reshaped_array = my_2d_array.reshape(5, 3)
print(reshaped_array)
Indexing Arrays
Indexing helps you retrieve specific elements from an array. For one-dimensional arrays, it's straightforward:
print(my_array[2]) # Output: 3
For two-dimensional arrays, you need to specify the row and column index:
print(my_2d_array[1, 3]) # Output: 9
Useful Numpy Functions
Creating Arrays with Specific Values
Numpy provides functions to create arrays with specific values:
np.arange(start, stop, step): Creates an array with values from start to stop, with a step.
arr = np.arange(0, 10, 2)
print(arr) # Output: [0 2 4 6 8]
np.linspace(start, stop, num): Creates an array with num equally spaced values between start and stop.
arr = np.linspace(0, 10, 5)
print(arr) # Output: [ 0. 2.5 5. 7.5 10.]
np.ones(shape): Creates an array filled with ones.
arr = np.ones((3, 4))
print(arr)
np.zeros(shape): Creates an array filled with zeros.
arr = np.zeros((2, 5))
print(arr)
Random Values
Numpy can generate arrays with random values:
np.random.rand(shape): Generates an array with random values between 0 and 1.
arr = np.random.rand(3, 3)
print(arr)
np.random.randint(low, high, size): Generates an array with random integers between low and high.
arr = np.random.randint(0, 10, (2, 3))
print(arr)
Operations on Arrays
You can perform various operations on arrays like addition, multiplication, and more:
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# Addition
result = arr1 + arr2
print(result) # Output: [5 7 9]
# Multiplication
result = arr1 * arr2
print(result) # Output: [ 4 10 18]
Broadcasting
Broadcasting allows you to perform operations on arrays of different shapes:
arr = np.array([1, 2, 3])
result = arr + 2
print(result) # Output: [3 4 5]
Copying Arrays
When you assign an array to another variable, it doesn't create a copy; it just references the original array. Use the copy() function to create a true copy:
arr1 = np.array([1, 2, 3])
arr2 = arr1.copy()
arr2[0] = 99
print(arr1) # Output: [1 2 3]
print(arr2) # Output: [99 2 3]
Conclusion
Numpy is an essential library for anyone working with data in Python. It simplifies array operations and makes your code more efficient. Practice using these functions, and you'll find that handling arrays becomes a breeze.
Stay tuned for more tutorials where we'll dive deeper into data analysis with Numpy and other libraries. Happy coding!
Thank you for reading! If you found this post helpful, share it with your friends and family. Happy learning!
Commenti