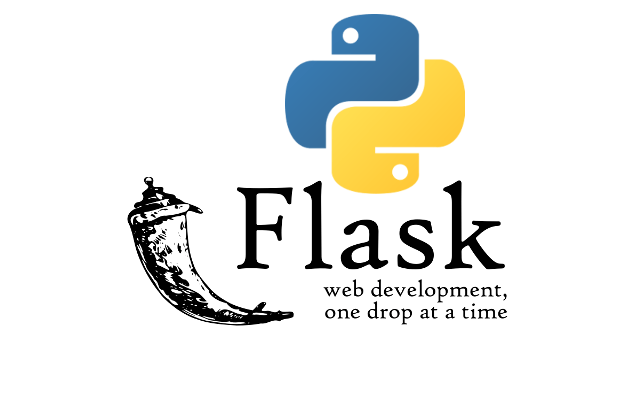
Hello everyone! Today, we're going to learn how to make our Flask web applications more visually appealing and interactive by integrating CSS and JavaScript. These two technologies are essential for creating modern, dynamic web pages. Let's dive in and see how we can use them with Flask!
What is Flask?
Flask is a web framework for Python that allows you to build web applications quickly and easily. It provides the tools you need to create web pages, handle user requests, and manage data. By integrating CSS and JavaScript, you can enhance the look and functionality of your Flask applications.
Setting Up a Simple Flask App
Before we start, let's set up a basic Flask app. Here's how you can do it:
Step 1: Install Flask
First, make sure you have Flask installed. You can do this by running the following command in your terminal:
pip install flask
Step 2: Create a Flask App
Open your code editor and create a new file called app.py. Add the following code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Step 3: Run Your App
In your terminal, navigate to the folder where app.py is located and run:
python app.py
Step 4: Visit Your App
Open your web browser and go to http://127.0.0.1:5000/. You should see your HTML page displayed.
Integrating CSS
CSS (Cascading Style Sheets) is used to style your web pages. It allows you to change colors, fonts, layouts, and more.
Creating a CSS File
Create a Static Folder: In the same directory as your app.py, create a folder named static.
Create a CSS File: Inside the static folder, create a file named style.css. Add the following CSS code:
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
h1 {
color: #333;
text-align: center;
padding: 20px;
}
p {
color: #666;
text-align: center;
}
Linking the CSS File
In your index.html, link the CSS file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>Welcome to My Flask App!</h1>
<p>This is a simple web page styled with CSS.</p>
</body>
</html>
Explanation: The {{ url_for('static', filename='style.css') }} function generates the URL for the CSS file.
Integrating JavaScript
JavaScript is used to add interactivity to your web pages. It allows you to create dynamic content, handle events, and more.
Creating a JavaScript File
Create a JavaScript File: Inside the static folder, create a file named script.js. Add the following JavaScript code:
document.addEventListener('DOMContentLoaded', function() { alert('Welcome to My Flask App!'); });
Linking the JavaScript File
In your index.html, link the JavaScript file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>Welcome to My Flask App!</h1>
<p>This is a simple web page styled with CSS.</p>
<script src="{{ url_for('static', filename='script.js') }}"></script>
</body>
</html>
Explanation: The <script> tag is used to include the JavaScript file. The {{ url_for('static', filename='script.js') }} function generates the URL for the JavaScript file.
Conclusion
Congratulations! You've learned how to integrate CSS and JavaScript into your Flask web applications. By using these technologies, you can create web pages that are not only functional but also visually appealing and interactive. As you continue to explore Flask, you'll discover more ways to enhance your web projects.
Stay tuned for more tutorials where we'll dive deeper into building complex web applications with Flask. Happy coding!
Comentarios