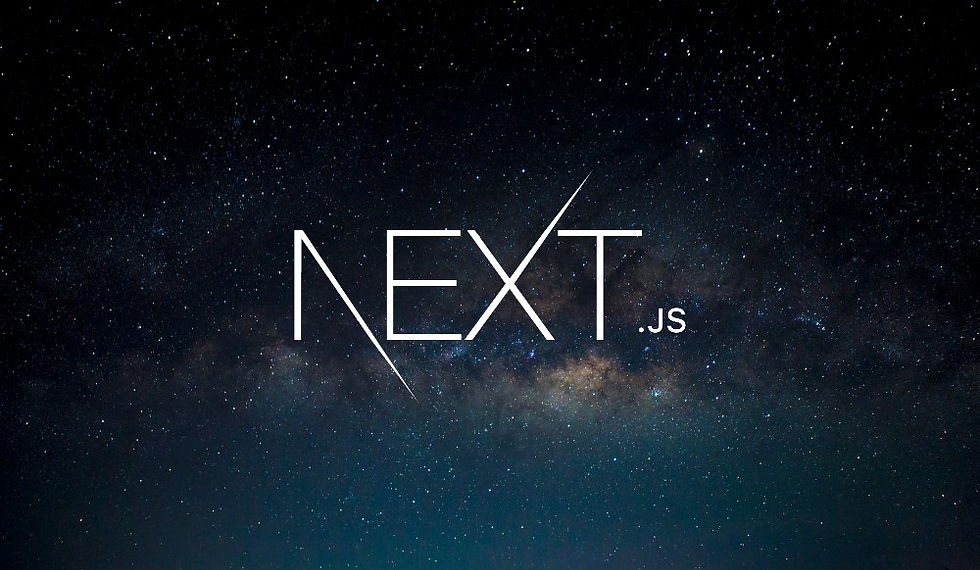
We now have a few different pages in our web application. You may be wondering why we need to create a separate folder for each route with a page.jsx file inside it. Previously, in older versions of Next.js, the approach was simpler. For example, the /about page would be in a file named about.jsx directly under the top-level pages folder, without the need to create a separate folder. So why did Next.js decide to adopt this new, seemingly more complex folder structure?
The reason is that this new structure allows us to put multiple files inside each folder, providing different bits of functionality for the same route. One of the most powerful features enabled by this structure is nested layouts.
What Are Nested Layouts?
Nested layouts allow you to define layout components that apply to specific routes or segments of your application. This means you can have a general layout for your entire application and more specific layouts for different sections. Let’s explore how this works in practice.
Step-by-Step Demo of Nested Layouts
Step 1: Root Layout
First, we have a layout.jsx file directly under our app folder. This acts as a template applied to all pages. Here's what it might look like:
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>[Header]</header>
<main>{children}</main>
<footer>[Footer]</footer>
</body>
</html>
);
}
This layout includes a header and a footer that will appear on every page.
Step 2: Create a Layout for a Specific Route
We can also create a layout for a specific route. For example, under the reviews folder, we can create a new file called layout.jsx:
export default function ReviewsLayout({ children }) {
return (
<div>
<div style={{ border: '2px solid red' }}>Reviews MenuBar</div>
{children}
</div>
);
}
Now, the reviews folder has two files: layout.jsx and page.jsx. The ReviewsLayout will apply to all pages under the /reviews path.
Step 3: Apply Nested Layouts
When we open the /reviews page, we will see the content defined in both the RootLayout and the ReviewsLayout. The nested layout applies not just to the ReviewsPage but to all pages inside this path, such as the Stardew Valley and Hollow Knight review pages.
// In RootLayout
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header style={{ border: '2px solid blue' }}>[Header]</header>
<main>{children}</main>
<footer style={{ border: '2px solid blue' }}>[Footer]</footer>
</body>
</html>
);
}
// In ReviewsLayout
export default function ReviewsLayout({ children }) {
return (
<div>
<div style={{ border: '2px solid red' }}>Reviews MenuBar</div>
{children}
</div>
);
}
With this setup, the /reviews route will display the "Reviews MenuBar" below the header and above the footer, while pages like /about or / will not show the "Reviews MenuBar".
Practical Uses of Nested Layouts
Nested layouts are particularly useful for adding common elements to specific sections of your application. For instance, you might want to show a sidebar with navigation links specific to the review pages. Here’s how you can update the ReviewsLayout to include a sidebar:
export default function ReviewsLayout({ children }) {
return (
<div style={{ display: 'flex' }}>
<aside style={{ border: '2px solid red', padding: '1rem' }}>
[Sidebar with Review Filters]
</aside>
<div style={{ marginLeft: '1rem' }}>
{children}
</div>
</div>
);
}
Conclusion
Nested layouts in Next.js provide a powerful way to create modular, reusable layout components that apply to specific parts of your application. This approach not only makes your application more organized but also allows you to easily manage and update different sections independently.
While the new folder structure may seem complex at first, it offers significant benefits in terms of scalability and maintainability. As you build more complex applications, you’ll find that nested layouts make it easier to manage shared components and styles across different routes.
Comments