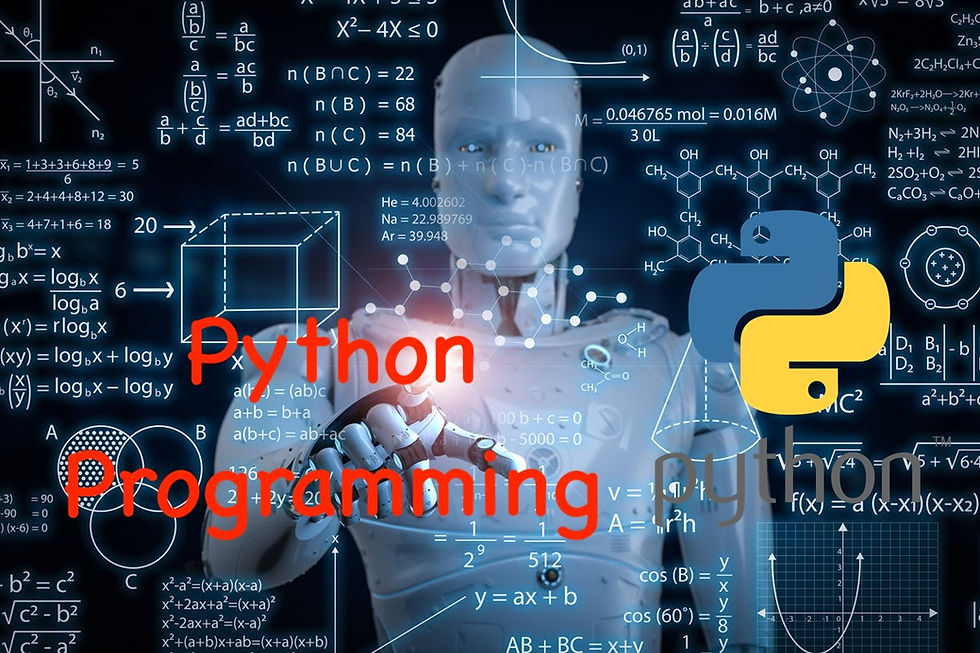
Hello everyone! Today, we are going to explore one of the most powerful libraries in Python for data manipulation and analysis: Pandas. In previous blog, we discussed Numpy, which helps us handle arrays efficiently. Now, it's time to dive into Pandas, which is essential for handling tabular data, like what you find in spreadsheets or databases.
What is Pandas?
Pandas is an open-source library that provides high-performance, easy-to-use data structures and data analysis tools for Python. It's extensively used for data manipulation, cleaning, and analysis, making it a must-have for data scientists and analysts.
Getting Started with Pandas
Installing Pandas
Before using Pandas, you need to install it. If you've installed Python manually or are using a different Python environment, you can install Pandas using pip:
pip install pandas
Or if you're using a conda environment:
conda install pandas
Importing Pandas
To use Pandas in your Python code, you need to import it. The common practice is to import Pandas using the alias pd:
import pandas as pd
Understanding DataFrames
What is a DataFrame?
A DataFrame is a two-dimensional data structure that stores data in a tabular format, similar to an Excel spreadsheet. It consists of rows and columns, where each column can have a different data type.
Creating DataFrames
You can create DataFrames in various ways. Let's start with a simple example using a dictionary:
import pandas as pd
data = {
'Column1': [1, 2, 3, 4, 5],
'Column2': [6, 7, 8, 9, 10],
'Column3': [11, 12, 13, 14, 15]
}
df = pd.DataFrame(data)
print(df)
Reading Data from Files
Pandas can read data from various file formats like CSV, Excel, SQL, and more. Here's how you can read a CSV file:
df = pd.read_csv('data.csv')
print(df.head()) # Display the first 5 rows
Saving DataFrames to Files
You can also save DataFrames to different file formats. For example, to save a DataFrame to a CSV file:
df.to_csv('output.csv', index=False)
The index=False argument ensures that the row indices are not saved in the file.
Exploring Data in DataFrames
Viewing Data
Pandas provides several methods to view data in a DataFrame:
df.head(n): Displays the first n rows (default is 5).
df.tail(n): Displays the last n rows (default is 5).
df.info(): Displays a summary of the DataFrame, including the data types and non-null counts.
df.describe(): Provides descriptive statistics for numerical columns.
print(df.head())
print(df.info())
print(df.describe())
Selecting Data
You can select specific columns or rows using various methods:
Selecting Columns: Use the column name as a key.
print(df['Column1'])
Selecting Rows: Use the iloc or loc methods.
# Using iloc (integer location-based indexing)
print(df.iloc[0]) # First row
# Using loc (label-based indexing)
print(df.loc[0]) # First row (if the index is labeled 0)
Filtering Data
You can filter data based on certain conditions:
# Filter rows where Column1 is greater than 2
filtered_df = df[df['Column1'] > 2]
print(filtered_df)
Modifying DataFrames
Adding and Removing Columns
You can easily add or remove columns in a DataFrame:
Adding a Column:
df['Column4'] = [16, 17, 18, 19, 20]
print(df)
Removing a Column:
df.drop('Column4', axis=1, inplace=True)
print(df)
Handling Missing Data
Pandas provides methods to handle missing data:
Checking for Missing Data:
print(df.isnull().sum())
Filling Missing Data:
df.fillna(0, inplace=True) # Fill missing values with 0
Dropping Missing Data:
df.dropna(inplace=True) # Remove rows with missing values
Advanced Operations
Grouping Data
You can group data by one or more columns and perform aggregate functions:
grouped_df = df.groupby('Column1').sum()
print(grouped_df)
Merging DataFrames
You can merge two DataFrames using various join operations:
df1 = pd.DataFrame({'key': ['A', 'B', 'C'], 'value': [1, 2, 3]})
df2 = pd.DataFrame({'key': ['A', 'B', 'D'], 'value': [4, 5, 6]})
merged_df = pd.merge(df1, df2, on='key', how='inner')
print(merged_df)
Conclusion
Pandas is an incredibly powerful library for data analysis and manipulation. It simplifies many tasks, from reading and writing files to exploring and modifying data. Practice using these functions, and you'll find that handling data becomes much easier.
Stay tuned for more tutorials where we'll dive deeper into data analysis with Pandas and other libraries. Happy coding!
Thank you for reading! If you found this post helpful, share it with your friends and family. Happy learning!
Comments