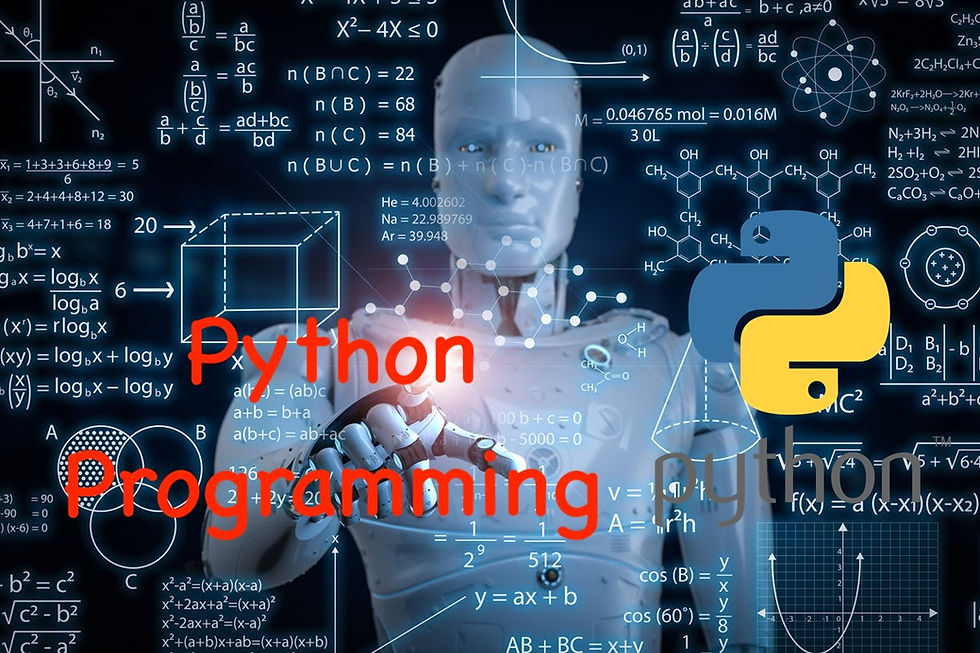
Hello everyone! Today, we continue our exciting journey into the world of Pandas, the powerful Python library for data manipulation and analysis. In our previous post, we explored accessing data elements, in-built functions like value_counts and unique, and how to handle DataFrames. Now, let’s dive deeper into reading various types of data files and understanding more in-built functions.
Reading Different File Formats with Pandas
Reading CSV Files
CSV (Comma-Separated Values) files are one of the most common data formats. Let's start by reading a simple CSV file.
import pandas as pd
# Reading a CSV file
df = pd.read_csv('data.csv')
print(df.head()) # Display the first 5 rows
Reading Excel Files
Pandas can also read Excel files. You need to specify the sheet name if your Excel file contains multiple sheets.
# Reading an Excel file
df_excel = pd.read_excel('data.xlsx', sheet_name='Sheet1')
print(df_excel.head())
Reading Other File Formats
Pandas can read various other file formats, such as JSON, HTML, and SQL databases. Here are some examples:
# Reading a JSON file
df_json = pd.read_json('data.json')
print(df_json.head())
# Reading data from an HTML table
df_html = pd.read_html('https://example.com/table')[0]
print(df_html.head())
Exploring Data with In-Built Functions
Pandas provides several in-built functions to help you understand your data better.
info()
The info() function provides a summary of the DataFrame, including the data types and non-null counts.
print(df.info())
describe()
The describe() function provides descriptive statistics for numerical columns.
print(df.describe())
value_counts()
The value_counts() function counts the unique values in a column.
print(df['Column1'].value_counts())
unique()
The unique() function returns the unique values in a column.
print(df['Column1'].unique())
Handling Different Delimiters in CSV Files
Sometimes, CSV files use different delimiters. Here’s how you can handle them:
Example with Semicolon (;) as Delimiter
If your CSV file uses a semicolon (;) as a delimiter, you can specify it using the sep parameter.
df_semicolon = pd.read_csv('data_semicolon.csv', sep=';')
print(df_semicolon.head())
Example with Tab (\t) as Delimiter
If your CSV file uses a tab (\t) as a delimiter, specify it using the sep parameter.
df_tab = pd.read_csv('data_tab.csv', sep='\t')
print(df_tab.head())
Handling Index Columns
Sometimes, you may want to set a specific column as the index.
Setting an Index Column
You can set an index column using the index_col parameter.
df_index = pd.read_csv('data.csv', index_col=0)
print(df_index.head())
Using Multiple Columns as Index
You can also set multiple columns as the index.
df_multi_index = pd.read_csv('data.csv', index_col=[0, 1])
print(df_multi_index.head())
Advanced Data Reading Techniques
Using usecols to Select Specific Columns
You can use the usecols parameter to read specific columns from a CSV file.
df_usecols = pd.read_csv('data.csv', usecols=['Column1', 'Column3'])
print(df_usecols.head())
Handling Missing Values
You can handle missing values while reading a CSV file using the na_values parameter.
df_na = pd.read_csv('data.csv', na_values=['NA', 'None'])
print(df_na.head())
Saving DataFrames to Files
You can save DataFrames to different file formats. For example, to save a DataFrame to a CSV file:
df.to_csv('output.csv', index=False)
You can also save it to other formats like Excel or JSON:
# Saving to Excel
df.to_excel('output.xlsx', index=False)
# Saving to JSON
df.to_json('output.json')
Conclusion
Pandas is an incredibly versatile library that makes data manipulation and analysis straightforward and efficient. By mastering the various ways to read data and use in-built functions, you can handle almost any data-related task. Practice these techniques, and you’ll become a Pandas pro in no time!
Stay tuned for more tutorials where we'll dive deeper into data analysis with Pandas and other libraries. Happy coding!
Thank you for reading! If you found this post helpful, share it with your friends and family. Happy learning!
Коментарі