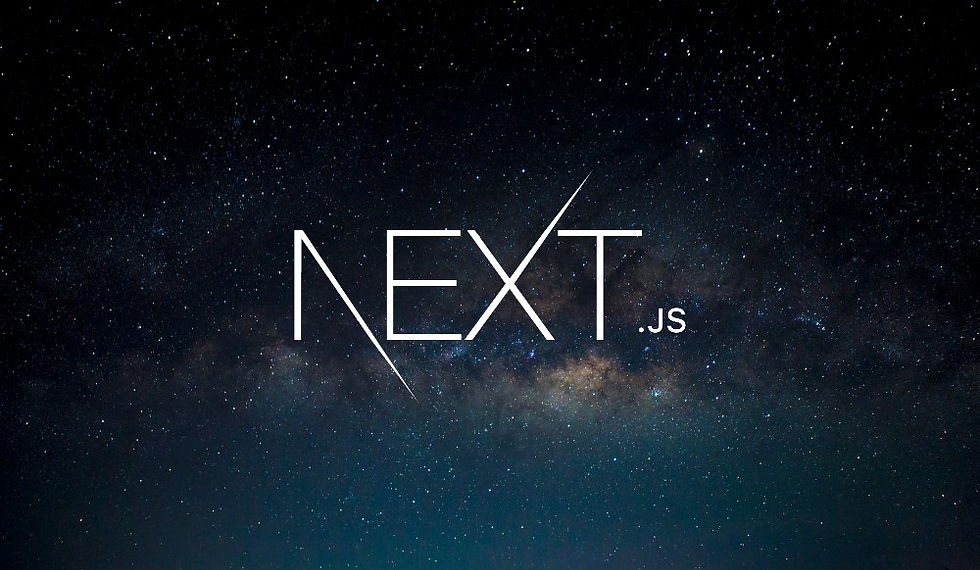
Navigation is a crucial part of any web application. In this blog post, we will explore how to implement navigation in a Next.js application using the Link component, and understand the benefits of client-side navigation.
Setting Up Navigation
To allow users to navigate between different pages of our application, we can add a navigation bar in the RootLayout component. This navigation bar will be displayed on all pages, making it easy for users to switch between different sections of the site.
Adding Navigation Links
First, let's create a basic navigation bar using HTML elements:
Add a nav Element: We will add a nav element inside the header of our RootLayout component. This nav element will contain an unordered list (ul) with list items (li) for each link.
Use HTML Anchor Tags: Initially, we will use the standard HTML anchor (<a>) tags to create links. Each anchor tag will have an href attribute pointing to the URL of the target page.
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/reviews">Reviews</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
</header>
<main>{children}</main>
</body>
</html>
);
}
Testing the Navigation
With the above setup, clicking on any of the links will navigate to the corresponding page, but it will refresh the entire page each time. This is typical of a Multi-Page Application (MPA), where each navigation action reloads the whole page from the server.
Introducing Client-Side Navigation
To enhance the navigation experience and make it faster, we can switch to client-side navigation using the Link component provided by Next.js.
Replacing Anchor Tags with Link Component
The Link component in Next.js allows for client-side navigation, which means that clicking on a link will dynamically update the content without a full page reload.
Import the Link Component: Import the Link component from the next/link module.
Replace Anchor Tags: Replace the standard HTML anchor (<a>) tags with the Link component. The Link component also accepts an href prop, similar to the anchor tag.
import Link from 'next/link';
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<header>
<nav>
<ul>
<li><Link href="/">Home</Link></li>
<li><Link href="/reviews">Reviews</Link></li>
<li><Link href="/about">About</Link></li>
</ul>
</nav>
</header>
<main>{children}</main>
</body>
</html>
);
}
Benefits of Client-Side Navigation
By using the Link component, we enable client-side navigation, which offers several advantages:
Faster Navigation: Clicking on a link updates the content without reloading the entire page. This results in a smoother and faster user experience.
Prefetching: Next.js prefetches linked pages in the background, making the navigation even faster. When you hover over a link, Next.js may start loading the necessary data before you even click.
Single Page Application Behavior: Once the initial page is loaded, subsequent navigations behave like a Single Page Application (SPA). The browser doesn't need to make full-page requests, reducing load times and improving performance.
Observing Network Requests
To see the difference in action, you can use the browser's developer tools to inspect network requests. Here's what you'll notice:
Initial Page Load: When you first load a page, the browser makes a request to fetch the full HTML document.
Client-Side Navigation: After the initial load, clicking on links no longer results in full HTML document requests. Instead, the content updates dynamically, and the navigation feels instantaneous.
Prefetching: Hovering over a link may trigger prefetch requests, which fetch data needed for the target page in advance. This helps reduce the time required to display the new content when you click the link.
Conclusion
Using the Link component in Next.js transforms your application into a hybrid of Multi-Page Application (MPA) and Single Page Application (SPA). It provides the best of both worlds: the initial page load is rendered on the server, while subsequent navigations are handled on the client-side for a faster and more responsive experience.
By simply replacing standard HTML anchor tags with the Link component, you can significantly enhance the performance and user experience of your Next.js application.
Comentários