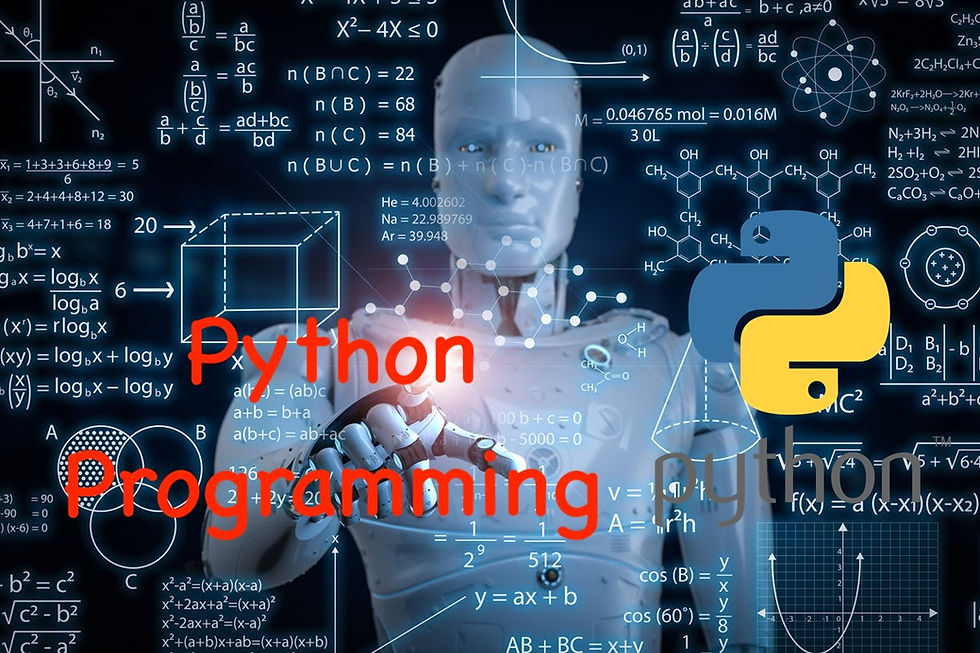
Hello, young coders and future Python experts! Today, we're going to dive into an amazing topic called "Data Classes" in Python. This topic is super beneficial and can save you a lot of time when working with classes and objects. By the end of this post, you'll see how data classes can simplify your code, making it cleaner and easier to understand. Let's jump right in!
What Are Data Classes?
Data classes are a feature introduced in Python 3.7. They provide a concise way to define classes that are primarily used to store data. With data classes, Python automatically generates several useful methods for you, such as init, repr, and eq. This makes your code cleaner and saves you from writing boilerplate code.
Traditional Way vs. Data Classes
Let's start by looking at the traditional way of creating a class in Python.
Traditional Way
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# Creating an object of Person
person1 = Person("Revanth", 31)
print(person1.name) # Output: Revanth
print(person1.age) # Output: 31
In the traditional way, you have to write the init method to initialize the attributes of the class. Now, let's see how we can simplify this using data classes.
Using Data Classes
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
# Creating an object of Person
person1 = Person("Revanth", 31)
print(person1.name) # Output: Revanth
print(person1.age) # Output: 31
In this example, we use the @dataclass decorator to define our class. We don't need to write the init method because the data class takes care of it for us!
More Features of Data Classes
Default Values
You can also provide default values for your attributes.
@dataclass
class Person:
name: str
age: int
profession: str = "Student"
# Creating an object of Person
person1 = Person("Revanth", 31)
print(person1.profession) # Output: Student
If you don't provide a value for profession, it will default to "Student".
Immutable Data Classes
Sometimes, you might want your class to be immutable, meaning its attributes can't be changed after the object is created. You can achieve this by setting frozen=True.
from dataclasses import dataclass
@dataclass(frozen=True)
class Point:
x: int
y: int
# Creating an object of Point
point = Point(3, 4)
print(point.x) # Output 3
# Trying to change the value will raise an error
# point.x = 5 # This will raise an error
Inheritance with Data Classes
Data classes also support inheritance. Let's see an example.
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
@dataclass
class Employee(Person):
employee_id: str
department: str
# Creating an object of Employee
employee = Employee("Revanth", 31, "123", "AI")
print(employee.name) # Output: Revanth
print(employee.department) # Output: AI
In this example, Employee inherits from Person, and we can still use all the attributes of Person.
Nested Data Classes
You can also nest data classes within other data classes.
from dataclasses import dataclass
@dataclass
class Address:
street: str
city: str
zip_code: str
@dataclass
class Person:
name: str
age: int
address: Address
# Creating an object of Address
address = Address("123 Main St", "Bangalore", "560001")
# Creating an object of Person
person = Person("Revanth", 31, address)
print(person.address.city) # Output: Bangalore
In this example, Person has an address attribute, which is an instance of the Address class.
Conclusion
Data classes are a powerful feature in Python that can simplify your code significantly. They reduce the amount of boilerplate code you need to write, making your code cleaner and easier to maintain. Whether you're dealing with default values, immutability, inheritance, or nested classes, data classes have got you covered.
So, start using data classes in your projects and see how they make your coding life easier. Happy coding, and keep exploring the wonders of Python!
Thank you for reading, and stay tuned for more exciting content. Have a great day!
Comments