Part 14: Python Functions Made Easy: From Basics to Advanced Concepts
- Revanth Reddy Tondapu
- May 21, 2024
- 3 min read
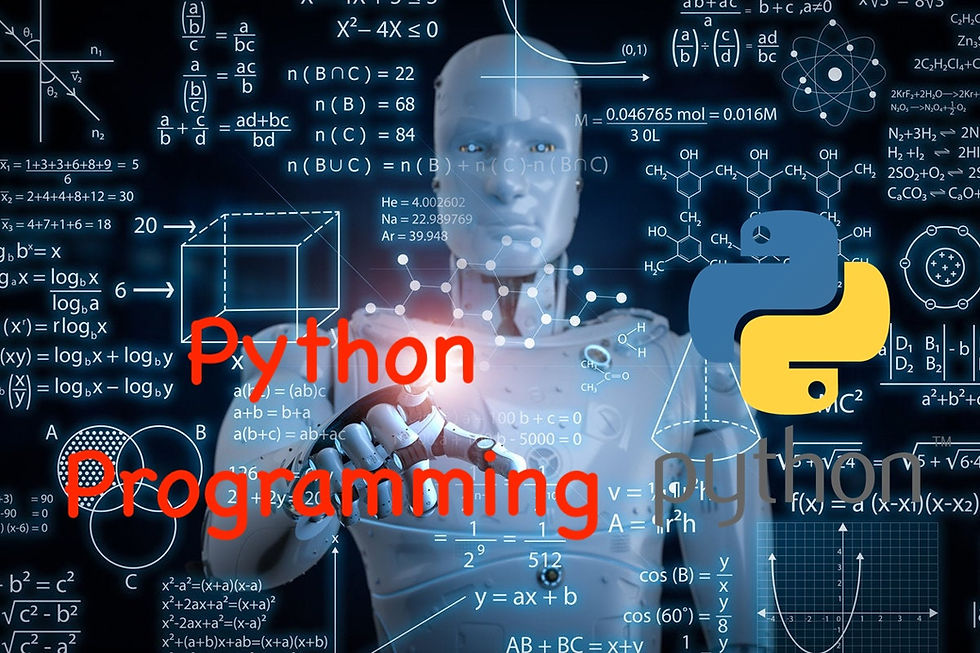
Hello everyone! Today, we're diving into an exciting topic in Python—functions! If you've been following along, you already know about various data structures in Python like lists, dictionaries, sets, and tuples. We've also touched on some fantastic libraries like NumPy, Pandas, Seaborn, and Matplotlib. Now, it's time to level up and explore the world of functions in Python. We'll also get into the difference between positional and keyword arguments. So, let's get started!
Why Do We Need Functions?
Imagine you have a piece of code that you need to use multiple times in your program. Instead of writing the same code over and over again, you can put that code in a function and call the function whenever you need it. This makes your code cleaner and easier to manage.
Example
Let's say you want to check if a number is even or odd. You can write a simple condition to do this:
number = 24
if number % 2 == 0:
print("Number is even")
else:
print("Number is odd")
Now, what if you need to check multiple numbers? Instead of writing this code repeatedly, you can create a function.
Writing a Function in Python
To define a function, you use the def keyword, followed by the function name and parentheses. Inside the parentheses, you can specify parameters.
def even_or_odd(number):
if number % 2 == 0:
print("Number is even")
else:
print("Number is odd")
Now, you can call this function with any number:
even_or_odd(24)
Calling the Function
even_or_odd(24) # Output: Number is even
even_or_odd(13) # Output: Number is odd
Positional vs. Keyword Arguments
Understanding the difference between positional and keyword arguments is crucial when dealing with functions.
Positional Arguments
Positional arguments are the most common type. These are the arguments that need to be passed in the correct order.
def greet(name, age):
print(f"Hello, my name is {name} and I am {age} years old.")
greet("Revanth", 29)
Keyword Arguments
Keyword arguments are a bit more flexible. You specify the name of the parameter along with its value, making the order irrelevant.
greet(age=29, name="Revanth")
Default Arguments
You can also set default values for parameters. If no value is provided, the default is used.
def greet(name, age=29):
print(f"Hello, my name is {name} and I am {age} years old.")
greet("Revanth") # Uses default age value of 29
greet("Revanth", 25) # Uses provided age value of 25
Print vs. Return
One important concept in functions is the difference between print and return.
print simply outputs the value to the console.
def say_hello():
print("Hello, welcome!")
say_hello()
Return
return sends back a value from the function, which you can store in a variable or use in further calculations.
def add_numbers(num1, num2):
return num1 + num2
result = add_numbers(24, 48)
print(result) # Output: 72
Returning Multiple Values
You can also return multiple values from a function.
def even_odd_sum(numbers):
even_sum = 0
odd_sum = 0
for number in numbers:
if number % 2 == 0:
even_sum += number
else:
odd_sum += number
return even_sum, odd_sum
numbers = [1, 2, 3, 4, 5, 6, 7, 8]
even_sum, odd_sum = even_odd_sum(numbers)
print(f"Even sum: {even_sum}, Odd sum: {odd_sum}")
Args and Kwargs
If you want to write a function that accepts a variable number of arguments, you can use args and *kwargs.
Args
*args allows you to pass a variable number of non-keyword arguments.
def print_numbers(*args):
for number in args:
print(number)
print_numbers(1, 2, 3, 4, 5)
Kwargs
**kwargs allows you to pass a variable number of keyword arguments.
def print_info(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_info(name="Revanth", age=29, city="New York")
Conclusion
Functions are a powerful feature in Python that help you write cleaner, more efficient code. By understanding how to use positional and keyword arguments, you can make your functions more flexible and easier to use. We also covered the difference between print and return, and how to handle multiple return values.
I hope you found this guide helpful and easy to understand. Stay tuned for our next session, where we'll dive into object-oriented concepts like classes, inheritance, and polymorphism. Until then, happy coding!
Comentarios