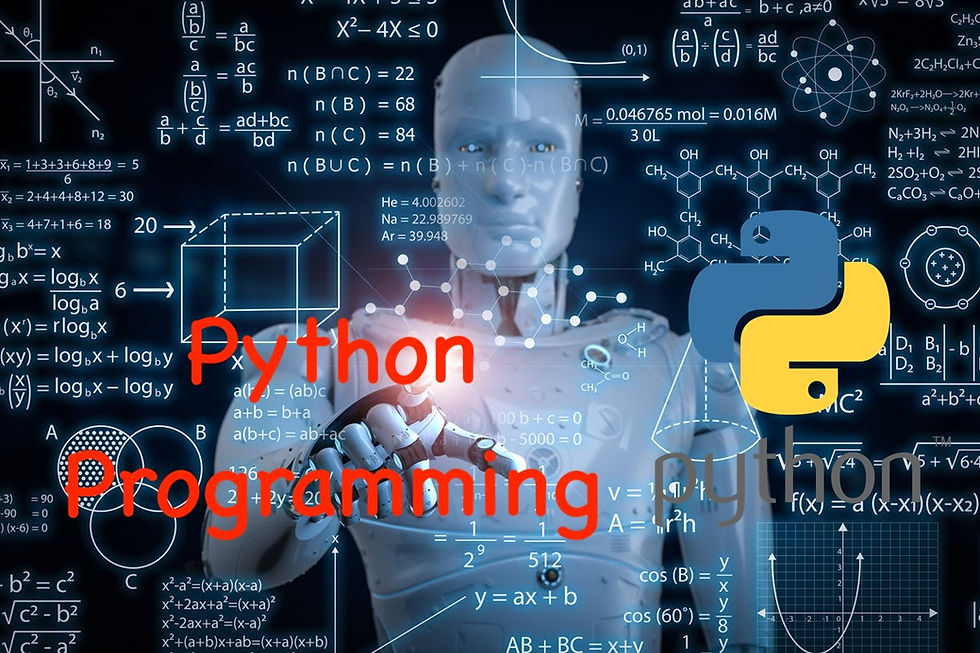
Hello young coders! Welcome to another exciting tutorial in our Advanced Python Series. Today, we are diving into two fascinating topics: Iterators and Generators. These concepts might sound tricky at first, but don't worry! We'll break them down step-by-step, so you can master them in no time. Ready to get started? Let’s go!
What Are Iterators?
Imagine you have a list of your favorite fruits: apples, bananas, and oranges. An iterator helps you go through each fruit one by one, just like flipping through pages of a book.
How Do Iterators Work?
An iterator is an object that contains a countable number of values. You can create an iterator from any iterable object like a list, tuple, or string.
Here’s a simple example:
fruits = ["apple", "banana", "orange"]
fruit_iterator = iter(fruits)
print(next(fruit_iterator)) # Output: apple
print(next(fruit_iterator)) # Output: banana
print(next(fruit_iterator)) # Output: orange
Explanation:
iter() function: This function is used to create an iterator object.
next() function: This function retrieves the next item from the iterator. When there are no more items, it raises a StopIteration exception.
What Are Generators?
Generators are special functions that allow you to create iterators in a more convenient way. They help you generate items one at a time and only when needed, which saves memory.
How Do Generators Work?
Generators use the yield keyword instead of return to produce a series of values.
Here’s a simple example:
def fruit_generator():
yield "apple"
yield "banana"
yield "orange"
fruits = fruit_generator()
print(next(fruits)) # Output: apple
print(next(fruits)) # Output: banana
print(next(fruits)) # Output: orange
Explanation:
yield keyword: This keyword is used in place of return to generate a value and pause the function, saving its state for the next call.
Why Use Generators?
Generators are useful because they allow you to create sequences of items without storing all of them in memory at once. This is especially helpful when working with large datasets.
Example: Generating a Sequence of Numbers
Let’s create a generator that produces the first 5 even numbers.
def even_numbers():
number = 0
while number <= 8:
yield number
number += 2
evens = even_numbers()
print(next(evens)) # Output: 0
print(next(evens)) # Output: 2
print(next(evens)) # Output: 4
print(next(evens)) # Output: 6
print(next(evens)) # Output: 8
Explanation:
while loop: This keeps generating even numbers until the number exceeds 8.
yield statement: This produces each even number and pauses the function until the next call.
Key Differences Between Iterators and Generators
Creation:
Iterator: Created using the iter() function.
Generator: Created using a function with the yield keyword.
Memory Usage:
Iterator: Can use more memory as it might store all items.
Generator: Uses less memory because it generates items one at a time.
Syntax:
Iterator: Requires defining an iterable and using iter() and next().
Generator: Simplifies iteration with the yield keyword inside a function.
Conclusion
Iterators and generators are powerful tools in Python that help you manage sequences of data efficiently. Iterators allow you to traverse through elements one by one, while generators let you create items on the fly without using too much memory.
Keep practicing these concepts, and soon you'll be a pro at writing efficient Python code. Stay tuned for more exciting tutorials, and happy coding!
If you enjoyed this tutorial, make sure to share it with your friends and keep exploring the world of Python. Thank you for joining us, and see you in the next tutorial!
Comentarios