Real-Time Updates with Strapi and Next.js Using Socket.IO: A Step-by-Step Guide
- Revanth Reddy Tondapu
- Sep 20, 2024
- 3 min read
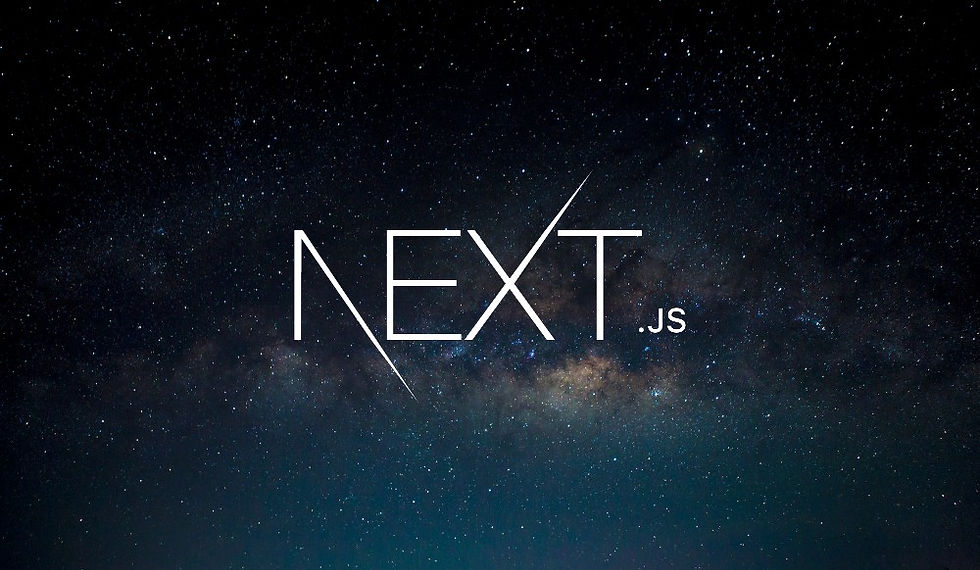
Real-time updates are crucial for modern web applications, offering instantaneous feedback and a seamless user experience. This blog post will guide you through creating a proof of concept (POC) that employs Socket.IO for real-time updates between a Strapi backend and a Next.js frontend. The POC will involve creating a custom API endpoint in Strapi that triggers an event, and the frontend will receive this event in real-time.
Overview
Step 1: Backend Setup (Strapi)
1.1 Create a Strapi Project
First, create a new Strapi project.
npx create-strapi-app my-strapi-app --quickstart
This command will generate a new Strapi project in a folder named my-strapi-app.
1.2 Install Dependencies
Navigate to your Strapi project directory and install socket.io.
cd my-strapi-app
npm install socket.io
1.3 Configure Socket.IO in Strapi
Create or update the bootstrap function to initialize Socket.IO.
config/functions/bootstrap.js
"use strict";
module.exports = async ({ strapi }) => {
const { Server } = require("socket.io");
const io = new Server(strapi.server.httpServer, {
cors: {
origin: "http://localhost:3000",
methods: ["GET", "POST"],
},
});
strapi.io = io;
io.on("connection", (socket) => {
console.log("A user connected");
socket.emit("message", "Hello from socket.io");
});
};
1.4 Create a Custom API Endpoint
Create a controller and route for a custom API endpoint that will trigger a Socket.IO event.
src/api/custom/controllers/custom.js
"use strict";
module.exports = {
async triggerEvent(ctx) {
try {
const payload = ctx.request.body;
const response = { success: true, data: payload };
// Emit a Socket.IO event
strapi.io.emit("customEvent", response);
ctx.send({ response });
} catch (error) {
strapi.log.error("Error triggering event", error);
ctx.internalServerError("Error triggering event");
}
},
};
src/api/custom/routes/custom.js
"use strict";
module.exports = {
routes: [
{
method: 'POST',
path: '/custom/trigger-event',
handler: 'custom.triggerEvent',
config: {
policies: [],
middlewares: [],
},
},
],
};
Step 2: Frontend Setup (Next.js)
2.1 Create a Next.js Project
First, create a new Next.js project.
npx create-next-app my-next-app
Navigate to your Next.js project directory.
cd my-next-app
2.2 Install Dependencies
Install socket.io-client to connect to the Socket.IO server.
npm install socket.io-client
2.3 Set Up Socket.IO Client in Next.js
Create a Next.js page that connects to the Socket.IO server and listens for events.
pages/socket-io.js
import { useEffect, useState } from 'react';
import io from 'socket.io-client';
const socket = io("http://localhost:1337"); // Replace with your Strapi server URL
function SocketIOPage() {
const [data, setData] = useState(null);
useEffect(() => {
socket.on('customEvent', (eventData) => {
console.log("Received custom event:", eventData);
setData(eventData.data);
});
return () => {
socket.off('customEvent');
};
}, []);
const triggerEvent = async () => {
await fetch('http://localhost:1337/custom/trigger-event', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ sample: "data" }),
});
};
return (
<div>
<button onClick={triggerEvent}>Trigger Event</button>
<div>
{data && <pre>{JSON.stringify(data, null, 2)}</pre>}
</div>
</div>
);
}
export default SocketIOPage;
Step 3: Testing Your POC
3.1 Start the Backend
Navigate to your Strapi project directory and run the server.
cd my-strapi-app
npm run develop
Ensure your Strapi server is running. You should see the Socket.IO server start up as part of Strapi’s initialization.
3.2 Start the Frontend
Navigate to your Next.js project directory and run the development server.
cd my-next-app
npm run dev
Open your browser and navigate to http://localhost:3000/socket-io.
3.3 Test the Integration
Click the "Trigger Event" button on your Next.js app.
Verify that the event is emitted from Strapi and received by the Next.js frontend.
Check the console logs and UI to ensure that the data is displayed correctly.
Summary
Backend (Strapi):
Frontend (Next.js):
Created a new Next.js project.
Installed socket.io-client to connect to the Socket.IO server.
Created a page that triggers the API and listens for events.
Conclusion
By following these steps, you should have a fully functional POC for real-time updates between Strapi and a Next.js frontend using Socket.IO. This setup can serve as a powerful foundation for building more complex real-time features in your applications. Real-time communication can significantly enhance user experience by providing instant feedback and seamless interactions. If you encounter any issues or need further assistance, feel free to reach out!
Comments