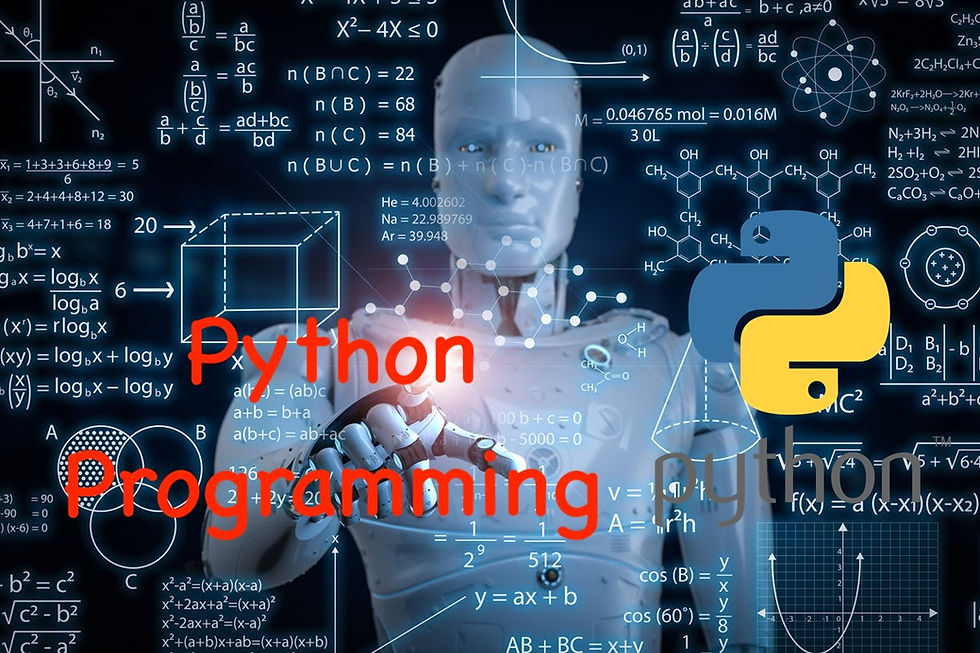
Hey there, curious coders! Today, we’re diving into the fascinating world of Python clones—specifically, the difference between shallow copy and deep copy. By the end of this post, you’ll understand how these two types of copies work and when to use each one. Let’s get started with some cool examples!
What Are We Learning Today?
= (Assignment) Operation
Shallow Copy
Deep Copy
Assignment Operation (=)
First, let's talk about the basic assignment operation. When you use the = operator to assign one list to another, both lists point to the same memory location.
Example
list1 = [1, 2, 3, 4]
list2 = list1 # Assignment operation
# Changing an element in list2
list2[1] = 100
print("List1:", list1) # Output: [1, 100, 3, 4]
print("List2:", list2) # Output: [1, 100, 3, 4]
In this example, changing an element in list2 also changes it in list1 because both lists point to the same memory location.
Shallow Copy
A shallow copy creates a new object but does not create copies of the objects within the original object. It copies the references of the objects within the collection.
Shallow Copy with Simple List
list1 = [1, 2, 3, 4]
list2 = list1.copy() # Shallow copy
# Changing an element in list2
list2[1] = 100
print("List1:", list1) # Output: [1, 2, 3, 4]
print("List2:", list2) # Output: [1, 100, 3, 4]
Here, changing an element in list2 does not affect list1 because they are in different memory locations.
Shallow Copy with Nested List
Now, let’s see what happens with a nested list.
list1 = [1, [2, 3], 4]
list2 = list1.copy() # Shallow copy
# Changing an element in the nested list in list2
list2[1][0] = 100
print("List1:", list1) # Output: [1, [100, 3], 4]
print("List2:", list2) # Output: [1, [100, 3], 4]
Here, changing an element in the nested list in list2 also changes it in list1. This happens because the nested list is still pointing to the same memory location in both list1 and list2.
Deep Copy
A deep copy creates a new object and recursively copies all objects found in the original object. This means that changes in the copied object do not affect the original object.
Example
To make a deep copy, we need to use the copy module.
import copy
list1 = [1, [2, 3], 4]
list2 = copy.deepcopy(list1) # Deep copy
# Changing an element in the nested list in list2
list2[1][0] = 100
print("List1:", list1) # Output: [1, [2, 3], 4]
print("List2:", list2) # Output: [1, [100, 3], 4]
Here, changing an element in the nested list in list2 does not affect list1 because they are in different memory locations.
Summary
Assignment Operation (=): Both variables point to the same memory location.
Shallow Copy: Copies the object but not the objects within the object. Nested objects are still shared.
Deep Copy: Creates a new object and recursively copies all objects. No objects are shared between the original and the copy.
Conclusion
Understanding the difference between shallow copy and deep copy is essential for managing data in Python. Use shallow copy when you want to create a new object but don’t mind sharing nested objects. Use deep copy when you need a completely independent copy of an object, including all nested objects.
I hope you enjoyed this tutorial and found it helpful. Keep practicing these concepts, and soon you’ll be a Python master! Stay tuned for more exciting tutorials and happy coding!
See you in the next post, and have a fantastic day!
Beginners often don't know that the append() function call in loop e.g. new_list.append(original_nested_list[counter]) is also a shallow copy operation. If this operation is run several times in a loop, each element of the new_list will contain the last set of elements from the original_nested_list. In such cases, the correct method is new_list.append(copy.deepcopy(original_nested_list[counter]) so that each element of the new_list will actually contain the element set of the original_nested_list as per counter.