Streamlining Your Workflow with AI-Driven Tools
- Revanth Reddy Tondapu
- Oct 22, 2024
- 5 min read
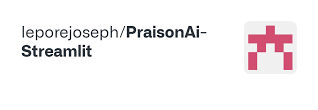
In today's digital landscape, integrating AI into your daily workflow can significantly enhance productivity and efficiency. From managing calendars to analyzing stock prices and generating creative content, AI has the potential to transform routine tasks into seamless operations. This blog post explores how you can leverage AI tools to automate and optimize various aspects of your professional life.
Automating Calendar Management
Managing your calendar can often be a time-consuming task, especially when juggling multiple meetings and appointments. By integrating AI with your calendar, you can automate scheduling, updating, and managing events effortlessly.
Setting Up and Managing Meetings
Here's a simple scenario: You need to schedule a meeting on Monday the 14th at 10:00 a.m. to discuss AI integration. Using an AI-driven tool, you can:
Create a Meeting: Schedule the meeting with a specific title, such as "AI Collaboration," and set it for the desired date and time.
Update Details: Change the meeting title or other details as needed, with just a few commands.
Reschedule: Move the meeting to a different date or time, e.g., changing it to Tuesday the 15th at the same time, ensuring no conflicts arise.
This level of automation allows you to focus on the content of your meetings rather than the logistics.
Analyzing Stock Prices
Staying updated with stock market trends is crucial for making informed investment decisions. AI tools can assist you by providing quick access to historical stock price data and visualizing trends through charts.
Example Use Case
Suppose you want to analyze the stock prices of a company over the past five days. An AI tool can:
Fetch Data: Retrieve closing stock prices for each day.
Visualize Trends: Generate charts that display the price movements over the specified period, allowing for easy comparison and analysis.
This capability simplifies the process of tracking and interpreting stock market performance.
Accessing the Latest AI News
Keeping up with advancements in AI technology is essential for staying competitive. AI tools can aggregate the latest news and updates, delivering them directly to you.
Example Scenario
Imagine you need to know about recent developments in AI, such as new features or product releases. By integrating an AI news aggregator, you can:
Receive Summaries: Get concise updates on the latest AI advancements, such as improvements in personal AI assistants.
Stay Informed: Access in-depth articles and analyses, helping you understand the implications of these developments.
This integration ensures you are always informed about the latest trends and innovations in AI.
Creating Visual Content
AI has made significant strides in generating creative content, including images and graphics, with minimal input. This capability can be particularly useful for marketing, presentations, and more.
Example Application
Suppose you want to create an image of an astronaut walking on Mars. An AI tool can:
Generate Images: Use simple commands to create high-quality visuals based on your specifications.
Customize Outputs: Tailor the generated images to fit your specific needs, whether for professional or personal use.
This functionality empowers you to produce engaging content quickly and efficiently.
Building Custom AI Tools
For those looking to tailor AI solutions to their unique workflows, creating custom tools is a viable option. By leveraging available APIs and libraries, you can build applications that meet your specific requirements.
Steps to Create a Custom Tool
Install Necessary Libraries: Use package managers to install required libraries, such as those for accessing calendar APIs.
Set Up Authentication: Configure OAuth credentials to authenticate API requests securely.
Develop Functions: Write Python functions to automate tasks like checking and updating calendar events.
Integrate with Your Workflow: Seamlessly incorporate these functions into your daily operations, enhancing productivity.
pip install google-api-python-client google-auth-oauthlib
# tools.py
from google.oauth2.credentials import Credentials
from googleapiclient.discovery import build
from google.auth.transport.requests import Request
from google_auth_oauthlib.flow import Flow
from google_auth_oauthlib.flow import InstalledAppFlow
import os
import json
import webbrowser
from http.server import HTTPServer, BaseHTTPRequestHandler
from urllib.parse import urlparse, parse_qs
import threading
from datetime import datetime, timedelta
import logging
# Set up logging
log_level = os.getenv('LOGLEVEL', 'INFO').upper()
logging.basicConfig(level=log_level)
logger = logging.getLogger(__name__)
logger.setLevel(log_level)
# Set up Google Calendar API
SCOPES = ['https://www.googleapis.com/auth/calendar']
def get_calendar_service():
logger.debug("Getting calendar service")
creds = None
token_dir = os.path.join(os.path.expanduser('~'), '.praison')
token_path = os.path.join(token_dir, 'token.json')
credentials_path = os.path.join(os.getcwd(), 'credentials.json')
if os.path.exists(token_path):
creds = Credentials.from_authorized_user_file(token_path, SCOPES)
logger.debug(f"Credentials loaded from {token_path}")
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
logger.debug(f"Refreshing credentials")
creds.refresh(Request())
else:
logger.debug(f"Starting new OAuth 2.0 flow")
flow = InstalledAppFlow.from_client_secrets_file(credentials_path, SCOPES)
logger.debug(f"Credentials path: {credentials_path}")
creds = flow.run_local_server(port=8090)
logger.debug(f"Setting up flow from {credentials_path}")
# creds = flow.run_local_server(port=8090) # Use run_local_server from InstalledAppFlow
# Ensure the ~/.praison directory exists
os.makedirs(os.path.dirname(token_path), exist_ok=True)
logger.debug(f"Saving credentials to {token_path}")
with open(token_path, 'w') as token:
token.write(creds.to_json())
logger.debug("Building calendar service")
return build('calendar', 'v3', credentials=creds)
check_calendar_def = {
"name": "check_calendar",
"description": "Check Google Calendar for events within a specified time range",
"parameters": {
"type": "object",
"properties": {
"start_time": {"type": "string", "description": "Start time in ISO format (e.g., '2023-04-20T09:00:00-07:00')"},
"end_time": {"type": "string", "description": "End time in ISO format (e.g., '2023-04-20T17:00:00-07:00')"}
},
"required": ["start_time", "end_time"]
}
}
async def check_calendar_handler(start_time, end_time):
try:
service = get_calendar_service()
events_result = service.events().list(calendarId='primary', timeMin=start_time,
timeMax=end_time, singleEvents=True,
orderBy='startTime').execute()
events = events_result.get('items', [])
logger.debug(f"Found {len(events)} events in the calendar")
logger.debug(f"Events: {events}")
return json.dumps(events)
except Exception as e:
return {"error": str(e)}
check_calendar = (check_calendar_def, check_calendar_handler)
add_calendar_event_def = {
"name": "add_calendar_event",
"description": "Add a new event to Google Calendar",
"parameters": {
"type": "object",
"properties": {
"summary": {"type": "string", "description": "Event title"},
"start_time": {"type": "string", "description": "Start time in ISO format"},
"end_time": {"type": "string", "description": "End time in ISO format"},
"description": {"type": "string", "description": "Event description"}
},
"required": ["summary", "start_time", "end_time"]
}
}
async def add_calendar_event_handler(summary, start_time, end_time, description=""):
try:
service = get_calendar_service()
event = {
'summary': summary,
'description': description,
'start': {'dateTime': start_time, 'timeZone': 'UTC'},
'end': {'dateTime': end_time, 'timeZone': 'UTC'},
}
event = service.events().insert(calendarId='primary', body=event).execute()
logger.debug(f"Event added: {event}")
return {"status": "success", "event_id": event['id']}
except Exception as e:
return {"error": str(e)}
add_calendar_event = (add_calendar_event_def, add_calendar_event_handler)
list_calendar_events_def = {
"name": "list_calendar_events",
"description": "List Google Calendar events for a specific date",
"parameters": {
"type": "object",
"properties": {
"date": {"type": "string", "description": "Date in YYYY-MM-DD format"}
},
"required": ["date"]
}
}
async def list_calendar_events_handler(date):
try:
service = get_calendar_service()
start_of_day = f"{date}T00:00:00Z"
end_of_day = f"{date}T23:59:59Z"
events_result = service.events().list(calendarId='primary', timeMin=start_of_day,
timeMax=end_of_day, singleEvents=True,
orderBy='startTime').execute()
events = events_result.get('items', [])
logger.debug(f"Found {len(events)} events in the calendar for {date}")
logger.debug(f"Events: {events}")
return json.dumps(events)
except Exception as e:
return {"error": str(e)}
list_calendar_events = (list_calendar_events_def, list_calendar_events_handler)
update_calendar_event_def = {
"name": "update_calendar_event",
"description": "Update an existing Google Calendar event",
"parameters": {
"type": "object",
"properties": {
"event_id": {"type": "string", "description": "ID of the event to update"},
"summary": {"type": "string", "description": "New event title"},
"start_time": {"type": "string", "description": "New start time in ISO format"},
"end_time": {"type": "string", "description": "New end time in ISO format"},
"description": {"type": "string", "description": "New event description"}
},
"required": ["event_id"]
}
}
async def update_calendar_event_handler(event_id, summary=None, start_time=None, end_time=None, description=None):
try:
service = get_calendar_service()
event = service.events().get(calendarId='primary', eventId=event_id).execute()
if summary:
event['summary'] = summary
if description:
event['description'] = description
if start_time:
event['start'] = {'dateTime': start_time, 'timeZone': 'UTC'}
if end_time:
event['end'] = {'dateTime': end_time, 'timeZone': 'UTC'}
updated_event = service.events().update(calendarId='primary', eventId=event_id, body=event).execute()
logger.debug(f"Event updated: {updated_event}")
return {"status": "success", "updated_event": updated_event}
except Exception as e:
return {"error": str(e)}
update_calendar_event = (update_calendar_event_def, update_calendar_event_handler)
delete_calendar_event_def = {
"name": "delete_calendar_event",
"description": "Delete a Google Calendar event",
"parameters": {
"type": "object",
"properties": {
"event_id": {"type": "string", "description": "ID of the event to delete"}
},
"required": ["event_id"]
}
}
async def delete_calendar_event_handler(event_id):
try:
service = get_calendar_service()
service.events().delete(calendarId='primary', eventId=event_id).execute()
logger.debug(f"Event deleted: {event_id}")
return {"status": "success", "message": f"Event with ID {event_id} has been deleted"}
except Exception as e:
return {"error": str(e)}
delete_calendar_event = (delete_calendar_event_def, delete_calendar_event_handler)
tools = [
check_calendar,
add_calendar_event,
list_calendar_events,
update_calendar_event,
delete_calendar_event,
]
By following these steps, you can create bespoke solutions that address your precise needs, leveraging the power of AI to its fullest potential.
For those interested in exploring and contributing to these AI tools, you can find a wealth of resources and code examples in the GitHub Repository.
Conclusion
Integrating AI into your workflow can revolutionize how you manage tasks, analyze data, and create content. Whether you're scheduling meetings, tracking stock prices, staying updated on AI news, or generating images, AI tools offer unparalleled efficiency and convenience. Explore these possibilities to transform your professional life and stay ahead in the ever-evolving digital landscape.
Comments