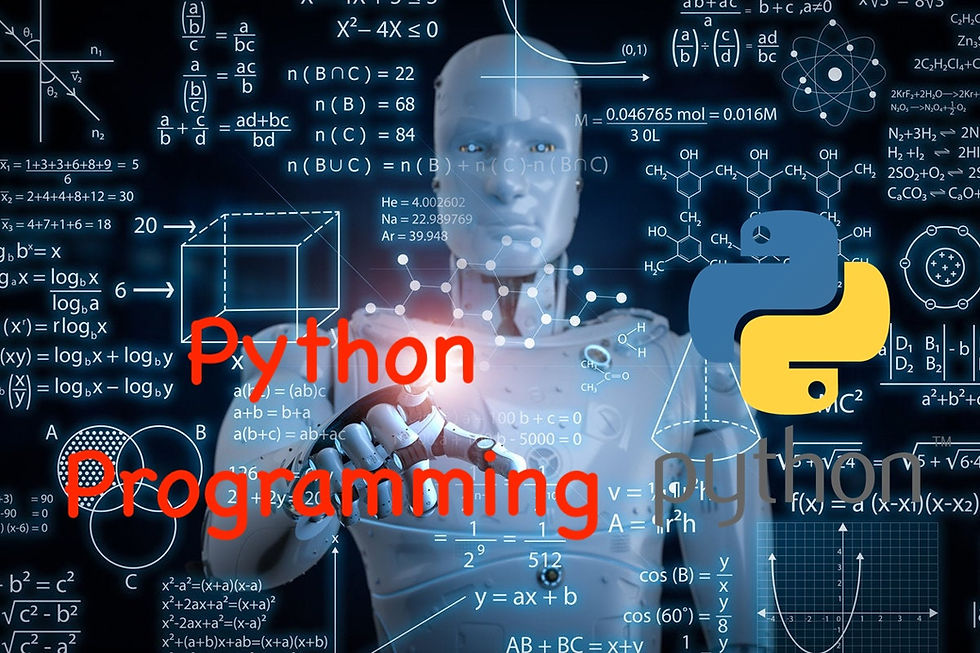
Hello, young coders! Today, we're going to uncover the magic behind one of Python's most powerful features—list comprehensions. Imagine if you could create lists in just one line of code. Sounds amazing, right? Well, that's exactly what list comprehensions allow you to do. Let's dive in and see how they work!
What is a List Comprehension?
In simple terms, a list comprehension is a concise way to create lists. Instead of writing several lines of code to fill a list, you can do it all in one line. This makes your code cleaner, easier to read, and often faster.
Why Use List Comprehensions?
List comprehensions are useful because they:
Make your code shorter and more readable
Reduce the need for loops and temporary variables
Are often faster than traditional methods for creating lists
Traditional Way vs. List Comprehensions
Let's start with a simple example. Suppose we want to create a list that contains the squares of numbers from another list. We'll first do it the traditional way and then see how much simpler it is with list comprehensions.
Traditional Way
def list_square(numbers):
new_list = []
for i in numbers:
new_list.append(i * i)
return new_list
# Using the function
numbers = [1, 2, 3, 4, 5]
squared_numbers = list_square(numbers)
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Here, we:
Defined a function called list_square
Created an empty list called new_list
Used a loop to go through each number in the input list
Squared each number and added it to new_list
Returned the new list of squared numbers
Using List Comprehensions
Now, let's see how we can do the same thing with a list comprehension.
List Comprehension Way
numbers = [1, 2, 3, 4, 5]
squared_numbers = [i * i for i in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Wow! Look how short and sweet that is. Let's break it down:
We start with square brackets [] to indicate we're creating a list.
Inside the brackets, we write the expression i * i (what we want to do with each item).
Then, we add for i in numbers to loop through each item in the list.
And that's it! This single line of code does everything our function did in multiple lines.
Adding Conditions
List comprehensions also allow us to add conditions. Let's say we want to square only the even numbers from our list. We can add an if condition to our list comprehension.
Adding a Condition
numbers = [1, 2, 3, 4, 5, 6]
even_squared_numbers = [i * i for i in numbers if i % 2 == 0]
print(even_squared_numbers) # Output: [4, 16, 36]
Here, we:
Added if i % 2 == 0 at the end to check if the number is even.
Only square the number if the condition is true.
Comparing Traditional and List Comprehensions
To see the power of list comprehensions, let's compare both methods side by side:
Traditional Way with Condition
def list_square_even(numbers):
new_list = []
for i in numbers:
if i % 2 == 0:
new_list.append(i * i)
return new_list
# Using the function
numbers = [1, 2, 3, 4, 5, 6]
squared_even_numbers = list_square_even(numbers)
print(squared_even_numbers) # Output: [4, 16, 36]
List Comprehension Way with Condition
numbers = [1, 2, 3, 4, 5, 6]
squared_even_numbers = [i * i for i in numbers if i % 2 == 0]
print(squared_even_numbers) # Output: [4, 16, 36]
The list comprehension is much shorter and easier to read. Plus, it's easier to spot what the code is doing at a glance.
Conclusion
List comprehensions are a powerful and elegant way to create lists in Python. They make your code shorter, cleaner, and more efficient. Whether you need to create a list of squares, filter items, or even combine multiple lists, list comprehensions can help you do it all in a single line of code.
I hope you enjoyed learning about list comprehensions and are excited to start using them in your projects. Keep practicing, and you'll soon find that writing Python code becomes faster and more fun!
Stay tuned for more exciting Python tutorials. Happy coding!
Comments