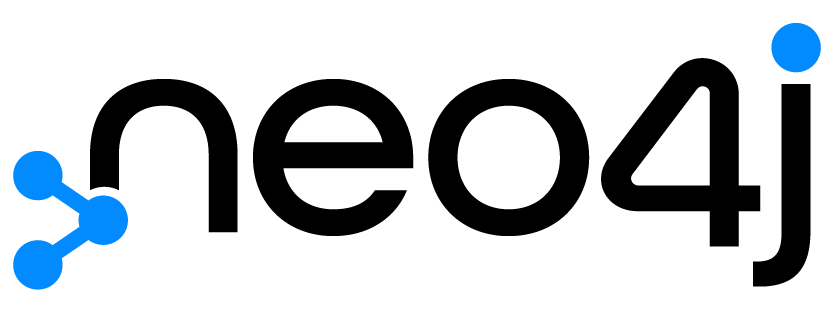
Introduction
In the previous part of this blog, we set up Neo4j using Docker Compose. Now, we will continue by showing how to interact with Neo4j from a Python application. We'll cover examples of creating, retrieving, updating, and deleting data in Neo4j using Python.
Prerequisites
To follow along, you should have the following:
Neo4j running via Docker Compose (as set up in the previous part).
Python installed on your machine.
Conda installed for managing virtual environments.
Step 1: Create a Virtual Environment
Let's start by setting up a virtual environment. This will help manage our dependencies and keep our project organized.
conda create -p venv python=3.10
conda activate venv/
Step 2: Install Required Packages
Next, create a requirements.txt file with the following content:
neo4j
python-dotenv
Install the required packages using pip:
pip install -r requirements.txt
Step 3: Set Up Environment Variables
Create a .env file to securely store your API key and other sensitive information:
NEO4J_URI=bolt://localhost:7687
NEO4J_USERNAME=neo4j
NEO4J_PASSWORD=securepassword
Load these environment variables in your Python script using the python-dotenv package.
Step 4: Connecting to Neo4j
Create a new Python file, say neo4j_example.py, and start by importing the necessary modules and setting up the connection to Neo4j.
import os
from neo4j import GraphDatabase
from dotenv import load_dotenv
# Load environment variables from .env file
load_dotenv()
# Connection details
uri = os.getenv("NEO4J_URI")
username = os.getenv("NEO4J_USERNAME")
password = os.getenv("NEO4J_PASSWORD")
# Create a Neo4j driver instance
driver = GraphDatabase.driver(uri, auth=(username, password))
# Define a function to close the driver
def close_driver():
driver.close()
Step 5: Creating Data
Let's create some data in the Neo4j database. We'll define a function to create a node for a person.
def create_person(tx, name, age):
query = (
"CREATE (p:Person {name: $name, age: $age}) "
"RETURN p"
)
result = tx.run(query, name=name, age=age)
return result.single()[0]
with driver.session() as session:
session.write_transaction(create_person, "Alice", 30)
session.write_transaction(create_person, "Bob", 25)
Step 6: Retrieving Data
Now, let's retrieve the data we just created. We'll define a function to get all persons from the database.
def get_all_persons(tx):
query = "MATCH (p:Person) RETURN p"
result = tx.run(query)
return [record["p"] for record in result]
with driver.session() as session:
persons = session.read_transaction(get_all_persons)
for person in persons:
print(person)
Step 7: Updating Data
Next, let's update a person's age. We'll define a function to update the age of a person by their name.
def update_person_age(tx, name, new_age):
query = (
"MATCH (p:Person {name: $name}) "
"SET p.age = $new_age "
"RETURN p"
)
result = tx.run(query, name=name, new_age=new_age)
return result.single()[0]
with driver.session() as session:
updated_person = session.write_transaction(update_person_age, "Alice", 31)
print(f"Updated person: {updated_person}")
Step 8: Deleting Data
Finally, let's delete a person from the database. We'll define a function to delete a person by their name.
def delete_person(tx, name):
query = (
"MATCH (p:Person {name: $name}) "
"DELETE p"
)
tx.run(query, name=name)
with driver.session() as session:
session.write_transaction(delete_person, "Bob")
Step 9: Closing the Driver
Don't forget to close the driver when you're done.
close_driver()
Full Script
Here is the full script combining all the parts:
import os
from neo4j import GraphDatabase
from dotenv import load_dotenv
# Load environment variables from .env file
load_dotenv()
# Connection details
uri = os.getenv("NEO4J_URI")
username = os.getenv("NEO4J_USERNAME")
password = os.getenv("NEO4J_PASSWORD")
# Create a Neo4j driver instance
driver = GraphDatabase.driver(uri, auth=(username, password))
# Define a function to close the driver
def close_driver():
driver.close()
# Function to create a person node
def create_person(tx, name, age):
query = (
"CREATE (p:Person {name: $name, age: $age}) "
"RETURN p"
)
result = tx.run(query, name=name, age=age)
return result.single()[0]
# Function to get all persons
def get_all_persons(tx):
query = "MATCH (p:Person) RETURN p"
result = tx.run(query)
return [record["p"] for record in result]
# Function to update a person's age
def update_person_age(tx, name, new_age):
query = (
"MATCH (p:Person {name: $name}) "
"SET p.age = $new_age "
"RETURN p"
)
result = tx.run(query, name=name, new_age=new_age)
return result.single()[0]
# Function to delete a person
def delete_person(tx, name):
query = (
"MATCH (p:Person {name: $name}) "
"DELETE p"
)
tx.run(query, name=name)
# Main script
with driver.session() as session:
session.write_transaction(create_person, "Alice", 30)
session.write_transaction(create_person, "Bob", 25)
persons = session.read_transaction(get_all_persons)
print("Persons in the database:")
for person in persons:
print(person)
updated_person = session.write_transaction(update_person_age, "Alice", 31)
print(f"Updated person: {updated_person}")
session.write_transaction(delete_person, "Bob")
persons = session.read_transaction(get_all_persons)
print("Persons in the database after deletion:")
for person in persons:
print(person)
# Close the driver
close_driver()
Conclusion
In this continuation of our Neo4j setup guide, we demonstrated how to interact with Neo4j using Python. We covered creating, retrieving, updating, and deleting nodes in the Neo4j graph database. Setting up a virtual environment and using environment variables ensures a clean and secure setup for your development.
Feel free to explore further and integrate Neo4j into your projects. Happy coding!
Comentarios